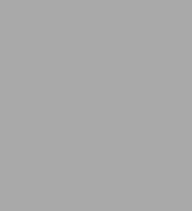
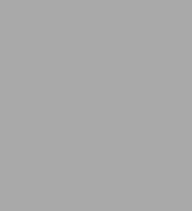
eBook
Related collections and offers
Overview
This edition of Robert Sedgewick's popular work provides current and comprehensive coverage of important algorithms for Java programmers. Michael Schidlowsky and Sedgewick have developed new Java implementations that both express the methods in a concise and direct manner and provide programmers with the practical means to test them on real applications.
Many new algorithms are presented, and the explanations of each algorithm are much more detailed than in previous editions. A new text design and detailed, innovative figures, with accompanying commentary, greatly enhance the presentation. The third edition retains the successful blend of theory and practice that has made Sedgewick's work an invaluable resource for more than 400,000 programmers!
This particular book, Parts 1-4, represents the essential first half of Sedgewick's complete work. It provides extensive coverage of fundamental data structures and algorithms for sorting, searching, and related applications. Although the substance of the book applies to programming in any language, the implementations by Schidlowsky and Sedgewick also exploit the natural match between Java classes and abstract data type (ADT) implementations.
Highlights
- Java class implementations of more than 100 important practical algorithms
- Emphasis on ADTs, modular programming, and object-oriented programming
- Extensive coverage of arrays, linked lists, trees, and other fundamental data structures
- Thorough treatment of algorithms for sorting, selection, priority queue ADT implementations, and symbol table ADT implementations (search algorithms)
- Complete implementations for binomial queues, multiway radix sorting, randomized BSTs, splay trees, skip lists, multiway tries, B trees, extendible hashing, and many other advanced methods
- Quantitative information about the algorithms that gives you a basis for comparing them
- More than 1,000 exercises and more than 250 detailed figures to help you learn properties of the algorithms
Whether you are learning the algorithms for the first time or wish to have up-to-date reference material that incorporates new programming styles with classic and new algorithms, you will find a wealth of useful information in this book.
Product Details
ISBN-13: | 9780321623973 |
---|---|
Publisher: | Pearson Education |
Publication date: | 07/23/2002 |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 768 |
File size: | 15 MB |
Note: | This product may take a few minutes to download. |
About the Author
Robert Sedgewick is the William O. Baker Professor of Computer Science at Princeton University. He is a Director of Adobe Systems and has served on the research staffs at Xerox PARC, IDA, and INRIA. He earned his Ph.D from Stanford University under Donald E. Knuth.
Table of Contents
I. FUNDAMENTALS.
1. Introduction.Algorithms.
A Sample Problem: Connectivity.
Union-Find Algorithms.
Perspective.
Summary of Topics. 2. Principles of Algorithm Analysis.
Implementation and Empirical Analysis.
Analysis of Algorithms.
Growth of Functions.
Big-Oh notation.
Basic Recurrences.
Examples of Algorithm Analysis.
Guarantees, Predictions, and Limitations.
II. DATA STRUCTURES.
3. Elementary Data Structures.Building Blocks.
Arrays.
Linked Lists.
Elementary List Processing.
Memory Allocation for Lists.
Strings.
Compound Data Structures. 4. Abstract Data Types.
Collections of Items.
Pushdown Stack ADT.
Examples of Stack ADT Clients.
Stack ADT Implementations.
Generic Implementations.
Creation of a New ADT.
FIFO Queues and Generalized Queues.
Duplicate and Index Items.
First-Class ADTs.
Application-Based ADT Example.
Perspective. 5. Recursion and Trees.
Recursive Algorithms.
Divide and Conquer.
Dynamic Programming.
Trees.
Mathematical Properties of Trees.
Tree Traversal.
Recursive Binary-Tree Algorithms.
Graph Traversal.
Perspective.
III. SORTING.
6. Elementary Sorting Methods.Rules of the Game.
Generic Sort Implementations.
Selection Sort.
Insertion Sort.
Bubble Sort.
Performance Characteristics of Elementary Sorts.
Algorithm Visualization.
Shellsort.
Sorting Linked Lists.
Key-Indexed Counting. 7. Quicksort 315.
The Basic Algorithm.
Performance Characteristics of Quicksort.
Stack Size.
Small Subfiles.
Median-of-Three Partitioning.
Duplicate Keys.
Strings and Vectors.
Selection. 8. Merging and Mergesort.
Two-Way Merging.
Abstract In-Place Merge.
Top-Down Mergesort.
Improvements to the Basic Algorithm.
Bottom-Up Mergesort.
Performance Characteristics of Mergesort.
Linked-List Implementations of Mergesort.
Recursion Revisited. 9. Priority Queues and Heapsort.
Elementary Implementations.
Heap Data Structure.
Algorithms on Heaps.
Heapsort.
Priority-Queue ADT.
Priority Queues for Index Items.
Binomial Queues. 10. Radix Sorting.
Bits, Bytes, and Words.
Binary Quicksort.
MSD Radix Sort.
Three-Way Radix Quicksort.
LSD Radix Sort.
Performance Characteristics of Radix Sorts.
Sublinear-Time Sorts. 11. Special-Purpose Sorts.
Batcher's Odd-Even Mergesort.
Sorting Networks.
Sorting In Place.
External Sorting.
Sort-Merge Implementations.
Parallel Sort-Merge.
IV. SEARCHING.
12. Symbol Tables and BSTs.Symbol-Table Abstract Data Type.
Key-Indexed Search.
Sequential Search.
Binary Search.
Index Implementations with Symbol Tables.
Binary Search Trees.
Performance Characteristics of BSTs.
Insertion at the Root in BSTs.
BST Implementations of Other ADT Functions. 13. Balanced Trees.
Randomized BSTs.
Splay BSTs.
Top-Down 2-3-4 Trees.
Red-Black Trees.
Skip Lists.
Performance Characteristics. 14. Hashing.
Hash Functions.
Separate Chaining.
Linear Probing.
Double Hashing.
Dynamic Hash Tables.
Perspective. 15. Radix Search.
Digital Search Trees.
Tries.
Patricia Tries.
Multiway Tries and TSTs.
Text-String-Index Applications. 16. External Searching.
Rules of the Game.
Indexed Sequential Access.
B Trees.
Extendible Hashing.
Perspective. Appendix.
Index. 0201361205T06262002
Preface
This book is the first of three volumes that are intended to survey the most important computer algorithms in use today. This first volume (Parts 1-4) covers fundamental concepts (Part 1), data structures (Part 2), sorting algorithms (Part 3), and searching algorithms (Part 4); the second volume (Part 5) covers graphs and graph algorithms; and the (yet to be published) third volume (Parts 6-8) covers strings (Part 6), computational geometry (Part 7), and advanced algorithms and applications (Part 8).
The books are useful as texts early in the computer science curriculum, after students have acquired basic programming skills and familiarity with computer systems, but before they have taken specialized courses in advanced areas of computer science or computer applications. The books also are useful for self-study or as a reference for people engaged in the development of computer systems or applications programs because they contain implementations of useful algorithms and detailed information on these algorithms' performance characteristics. The broad perspective taken makes the series an appropriate introduction to the field.
Together the three volumes comprise the Third Edition of a book that has been widely used by students and programmers around the world for many years. I have completely rewritten the text for this edition, and I have added thousands of new exercises, hundreds of new figures, dozens of new programs, and detailed commentary on all the figures and programs. This new material provides both coverage of new topics and fuller explanations of many of the classic algorithms. A new emphasis on abstract data types throughout the books makes the programs more broadly useful and relevant in modern object-oriented programming environments. People who have read previous editions will find a wealth of new information throughout; all readers will find a wealth of pedagogical material that provides effective access to essential concepts.
These books are not just for programmers and computer science students. Everyone who uses a computer wants it to run faster or to solve larger problems. The algorithms that we consider represent a body of knowledge developed during the last 50 years that is the basis for the efficient use of the computer for a broad variety of applications. From N-body simulation problems in physics to genetic-sequencing problems in molecular biology, the basic methods described here have become essential in scientific research; and from database systems to Internet search engines, they have become essential parts of modern software systems. As the scope of computer applications becomes more widespread, so grows the impact of basic algorithms. The goal of this book is to serve as a resource so that students and professionals can know and make intelligent use of these fundamental algorithms as the need arises in whatever computer application they might undertake.
ScopeThis book, Algorithms in Java, Third Edition, Parts 1-4 , contains 16 chapters grouped into four major parts: fundamentals, data structures, sorting, and searching. The descriptions here are intended to give readers an understanding of the basic properties of as broad a range of fundamental algorithms as possible. The algorithms described here have found widespread use for years, and represent an essential body of knowledge for both the practicing programmer and the computerscience student. The second volume is devoted to graph algorithms, and the third consists of four additional parts that cover strings, geometry, and advanced topics. My primary goal in developing these books has been to bring together fundamental methods from these areas, to provide access to the best methods known for solving problems by computer.
You will most appreciate the material here if you have had one or two previous courses in computer science or have had equivalent programming experience: one course in programming in a high-level language such as Java, C, or C++, and perhaps another course that teaches fundamental concepts of programming systems. This book is thus intended for anyone conversant with a modern programming language and with the basic features of modern computer systems. References that might help to fill in gaps in your background are suggested in the text.
Most of the mathematical material supporting the analytic results is self-contained (or is labeled as beyond the scope of this book), so little specific preparation in mathematics is required for the bulk of the book, although mathematical maturity is definitely helpful.
Use in the CurriculumThere is a great deal of flexibility in how the material here can be taught, depending on the taste of the instructor and the preparation of the students. There is sufficient coverage of basic material for the book to be used to teach data structures to beginners, and there is sufficient detail and coverage of advanced material for the book to be used to teach the design and analysis of algorithms to upper-level students. Some instructors may wish to emphasize implementations and practical concerns; others may wish to emphasize analysis and theoretical concepts.
An elementary course on data structures and algorithms might emphasize the basic data structures in Part 2 and their use in the implementations in Parts 3 and 4. A course on design and analysis of algorithms might emphasize the fundamental material in Part 1 and Chapter 5, then study the ways in which the algorithms in Parts 3 and 4 achieve good asymptotic performance. A course on software engineering might omit the mathematical and advanced algorithmic material, and emphasize how to integrate the implementations given here into large programs or systems. A course on algorithms might take a survey approach and introduce concepts from all these areas.
Earlier editions of this book that are based on other programming languages have been used at scores of colleges and universities as a text for the second or third course in computer science and as supplemental reading for other courses. At Princeton, our experience has been that the breadth of coverage of material in this book provides our majors with an introduction to computer science that can be expanded on in later courses on analysis of algorithms, systems programming, and theoretical computer science, while providing the growing group of students from other disciplines with a large set of techniques that these people can put to good use immediately.
The exercisesnearly all of which are new to this third editionfall into several types. Some are intended to test understanding of material in the text, and simply ask readers to work through an example or to apply concepts described in the text. Others involve implementing and putting together the algorithms, or running empirical studies to compare variants of the algorithms and to learn their properties. Still others are a repository for important information at a level of detail that is not appropriate for the text. Reading and thinking about the exercises will pay dividends for every reader.
Algorithms of Practical UseAnyone wanting to use a computer more effectively can use this book for reference or for self-study. People with programming experience can find information on specific topics throughout the book. To a large extent, you can read the individual chapters in the book independently of the others, although, in some cases, algorithms in one chapter make use of methods from a previous chapter.
The orientation of the book is to study algorithms likely to be of practical use. The book provides information about the tools of the trade to the point that readers can confidently implement, debug, and put algorithms to work to solve a problem or to provide functionality in an application. Full implementations of the methods discussed are included, as are descriptions of the operations of these programs on a consistent set of examples.
Because we work with real code, rather than write pseudo-code, you can put the programs to practical use quickly. Program listings are available from the book's home page. You can use these working programs in many ways to help you study algorithms. Read them to check your understanding of the details of an algorithm, or to see one way to handle initializations, boundary conditions, and other awkward situations that often pose programming challenges. Run them to see the algorithms in action, to study performance empirically and check your results against the tables in the book, or to try your own modifications.
Characteristics of the algorithms and of the situations in which they might be useful are discussed in detail. Connections to the analysis of algorithms and theoretical computer science are developed in convi text. When appropriate, empirical and analytic results are presented to illustrate why certain algorithms are preferred. When interesting, the relationship of the practical algorithms being discussed to purely theoretical results is described. Specific information on performance characteristics of algorithms and implementations is synthesized, encapsulated, and discussed throughout the book.
Programming LanguageThe programming language used for all of the implementations is Java. The programs use a wide range of standard Java idioms, and the text includes concise descriptions of each construct.
Mike Schidlowsky and I developed a style of Java programming based on abstract data types that we feel is an effective way to present the algorithms and data structures as real programs. We have striven for elegant, compact, efficient, and portable implementations. The style is consistent whenever possible, so programs that are similar look similar.
For many of the algorithms in this book, the similarities hold regardless of the language: Quicksort is quicksort (to pick one prominent example), whether expressed in Ada, Algol-60, Basic, C, C++, Fortran, Java, Mesa, Modula-3, Pascal, PostScript, Smalltalk, or countless other programming languages and environments where it has proved to be an effective sorting method. On the one hand, our code is informed by experience with implementing algorithms in these and numerous other languages (C and C++ versions of this book are also available); on the other hand, some of the properties of some of these languages are informed by their designers' experience with some of the algorithms and data structures that we consider in this book.
Chapter 1 constitutes a detailed example of this approach to developing efficient Java implementations of our algorithms, and Chapter 2 describes our approach to analyzing them. Chapters 3 and 4 are devoted to describing and justifying the basic mechanisms that we use for data type and ADT implementations. These four chapters set the stage for the rest of the book.
Robert Sedgewick
Princeton, New Jersey, 2002
In the past decade, Java has become the language of choice for a variety of applications. But Java developers have found themselves repeatedly referring to references such as Sedgewick's Algorithms in C for solutions to common programming problems. There has long been an empty space on the bookshelf for a comparable reference work for Java; this book is here to fill that space.
We wrote the sample programs as utility methods to be used in a variety of contexts. To that end, we did not use the Java package mechanism. To focus on the algorithms at hand (and to expose the algorithmic basis of many fundamental library classes), we avoided the standard Java library in favor of more fundamental types. Proper error checking and other defensive practices would both substantially increase the amount of code and distract the reader from the core algorithms. Developers should introduce such code when using the programs in larger applications.
Although the algorithms we present are language independent, we have paid close attention to Java-specific performance issues. The timings throughout the book are provided as one context for comparing algorithms, and will vary depending on the virtual machine. As Java environments evolve, programs will perform as fast as natively compiled code, but such optimizations will not change the performance of algorithms relative to one another. We provide the timings as a useful reference for such comparisons.
I would like to thank Mike Zamansky, for his mentorship and devotion to the teaching of computer science, and Daniel Chaskes, Jason Sanders, and James Percy, for their unwavering support. I would also like to thank my family for their support and for the computer that bore my first programs. Bringing together Java with the classic algorithms of computer science was an exciting endeavor for which I am very grateful. Thank you, Bob, for the opportunity to do so.
Michael Schidlowsky
Oakland Gardens, New York, 2002