5
1
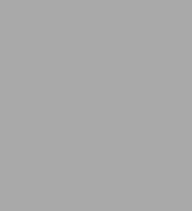
Clojure Programming: Practical Lisp for the Java World
628
by Chas Emerick, Christophe Grand, Brian Carper
Chas Emerick
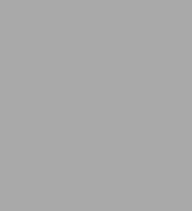
Clojure Programming: Practical Lisp for the Java World
628
by Chas Emerick, Christophe Grand, Brian Carper
Chas Emerick
Paperback
$49.99
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
49.99
In Stock
Overview
Clojure is a practical, general-purpose language that offers expressivity rivaling other dynamic languages like Ruby and Python, while seamlessly taking advantage of Java libraries, services, and all of the resources of the JVM ecosystem. This book helps you learn the fundamentals of Clojure with examples relating it to the languages you know already, in the domains and topics you work with every day. See how this JVM language can help eliminate unnecessary complexity from your programming practice and open up new options for solving the most challenging problems.
Clojure Programming demonstrates the language’s flexibility by showing how it can be used for common tasks like web programming and working with databases, up through more demanding applications that require safe, effective concurrency and parallelism, data analysis, and more. This in-depth look helps tie together the full Clojure development experience, from how to organize your project and an introduction to Clojure build tooling, to a tutorial on how to make the most of Clojure’s REPL during development, and how to deploy your finished application in a cloud environment.
- Learn how to use Clojure while leveraging your investment in the Java platform
- Understand the advantages of Clojure as an efficient Lisp for the JVM
- See how Clojure is used today in several practical domains
- Discover how Clojure eliminates the need for many verbose and complicated design patterns
- Deploy large or small web applications to the cloud with Clojure
Product Details
ISBN-13: | 9781449394707 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 04/19/2012 |
Pages: | 628 |
Product dimensions: | 7.08(w) x 9.02(h) x 1.27(d) |
About the Author
Chas Emerick is the founder of Snowtide Informatics, a small software company in Western Massachusetts. Since 2008, he has helped to develop the core Clojure language and many Clojure open source projects. Chas writes about Clojure, software development practices, entrepreneurship, and other passions at cemerick.com.
Christophe Grand is an independent consultant, based near Lyon, France. He tutors, trains and codes primarily in Clojure. A participant in developing the core Clojure language, he also authored the Enlive and Moustache libaries and is a contributor to Counterclockwise, the Clojure IDE for Eclipse. Christophe writes on Clojure at clj-me.cgrand.net.
Brian Carper is a professional programmer in the field of psychological research. He uses Clojure for data analysis and web development. He's the author of a Clojure-to-CSS compiler and relational database library, and writes about Clojure and other topics at http://briancarper.net.
Christophe Grand is an independent consultant, based near Lyon, France. He tutors, trains and codes primarily in Clojure. A participant in developing the core Clojure language, he also authored the Enlive and Moustache libaries and is a contributor to Counterclockwise, the Clojure IDE for Eclipse. Christophe writes on Clojure at clj-me.cgrand.net.
Brian Carper is a professional programmer in the field of psychological research. He uses Clojure for data analysis and web development. He's the author of a Clojure-to-CSS compiler and relational database library, and writes about Clojure and other topics at http://briancarper.net.
Table of Contents
Preface; Who Is This Book For?; How to Read This Book; Who’s “We”?; Acknowledgments; Conventions Used in This Book; Using Code Examples; Safari® Books Online; How to Contact Us; Chapter 1: Down the Rabbit Hole; 1.1 Why Clojure?; 1.2 Obtaining Clojure; 1.3 The Clojure REPL; 1.4 No, Parentheses Actually Won’t Make You Go Blind; 1.5 Expressions, Operators, Syntax, and Precedence; 1.6 Homoiconicity; 1.7 The Reader; 1.8 Namespaces; 1.9 Symbol Evaluation; 1.10 Special Forms; 1.11 Putting It All Together; 1.12 This Is Just the Beginning; Functional Programming and Concurrency; Chapter 2: Functional Programming; 2.1 What Does Functional Programming Mean?; 2.2 On the Importance of Values; 2.3 First-Class and Higher-Order Functions; 2.4 Composition of Function(ality); 2.5 Pure Functions; 2.6 Functional Programming in the Real World; Chapter 3: Collections and Data Structures; 3.1 Abstractions over Implementations; 3.2 Concise Collection Access; 3.3 Data Structure Types; 3.4 Immutability and Persistence; 3.5 Metadata; 3.6 Putting Clojure’s Collections to Work; 3.7 In Summary; Chapter 4: Concurrency and Parallelism; 4.1 Shifting Computation Through Time and Space; 4.2 Parallelism on the Cheap; 4.3 State and Identity; 4.4 Clojure Reference Types; 4.5 Classifying Concurrent Operations; 4.6 Atoms; 4.7 Notifications and Constraints; 4.8 Refs; 4.9 Vars; 4.10 Agents; 4.11 Using Java’s Concurrency Primitives; 4.12 Final Thoughts; Building Abstractions; Chapter 5: Macros; 5.1 What Is a Macro?; 5.2 Writing Your First Macro; 5.3 Debugging Macros; 5.4 Syntax; 5.5 When to Use Macros; 5.6 Hygiene; 5.7 Common Macro Idioms and Patterns; 5.8 The Implicit Arguments: &env and &form; 5.9 In Detail(:|:) -> and ->>; 5.10 Final Thoughts; Chapter 6: Datatypes and Protocols; 6.1 Protocols; 6.2 Extending to Existing Types; 6.3 Defining Your Own Types; 6.4 Implementing Protocols; 6.5 Protocol Introspection; 6.6 Protocol Dispatch Edge Cases; 6.7 Participating in Clojure’s Collection Abstractions; 6.8 Final Thoughts; Chapter 7: Multimethods; 7.1 Multimethods Basics; 7.2 Toward Hierarchies; 7.3 Hierarchies; 7.4 Making It Really Multiple!; 7.5 A Few More Things; 7.6 Final Thoughts; Tools, Platform, and Projects; Chapter 8: Organizing and Building Clojure Projects; 8.1 Project Geography; 8.2 Build; 8.3 Final Thoughts; Chapter 9: Java and JVM Interoperability; 9.1 The JVM Is Clojure’s Foundation; 9.2 Using Java Classes, Methods, and Fields; 9.3 Handy Interop Utilities; 9.4 Exceptions and Error Handling; 9.5 Type Hinting for Performance; 9.6 Arrays; 9.7 Defining Classes and Implementing Interfaces; 9.8 Using Clojure from Java; 9.9 Collaborating Partners; Chapter 10: REPL-Oriented Programming; 10.1 Interactive Development; 10.2 Tooling; 10.3 Debugging, Monitoring, and Patching Production in the REPL; 10.4 Limitations to Redefining Constructs; 10.5 In Summary; Practicums; Chapter 11: Numerics and Mathematics; 11.1 Clojure Numerics; 11.2 Clojure Mathematics; 11.3 Equality and Equivalence; 11.4 Optimizing Numeric Performance; 11.5 Visualizing the Mandelbrot Set in Clojure; Chapter 12: Design Patterns; 12.1 Dependency Injection; 12.2 Strategy Pattern; 12.3 Chain of Responsibility; 12.4 Aspect-Oriented Programming; 12.5 Final Thoughts; Chapter 13: Testing; 13.1 Immutable Values and Pure Functions; 13.2 clojure.test; 13.3 Growing an HTML DSL; 13.4 Relying upon Assertions; Chapter 14: Using Relational Databases; 14.1 clojure.java.jdbc; 14.2 Korma; 14.3 Hibernate; 14.4 Final Thoughts; Chapter 15: Using Nonrelational Databases; 15.1 Getting Set Up with CouchDB and Clutch; 15.2 Basic CRUD Operations; 15.3 Views; 15.4 _changes: Abusing CouchDB as a Message Queue; 15.5 À la Carte Message Queues; 15.6 Final Thoughts; Chapter 16: Clojure and the Web; 16.1 The “Clojure Stack”; 16.2 The Foundation: Ring; 16.3 Routing Requests with Compojure; 16.4 Templating; 16.5 Final Thoughts; Chapter 17: Deploying Clojure Web Applications; 17.1 Java and Clojure Web Architecture; 17.2 Running Web Apps Locally; 17.3 Web Application Deployment; 17.4 Going Beyond Simple Web Application Deployment; Miscellanea; Chapter 18: Choosing Clojure Type Definition Forms Wisely; Chapter 19: Introducing Clojure into Your Workplace; 19.1 Just the Facts…; 19.2 Emphasize Productivity; 19.3 Emphasize Community; 19.4 Be Prudent; Chapter 20: What’s Next?; 20.1 (dissoc Clojure 'JVM); 20.2 4Clojure; 20.3 Overtone; 20.4 core.logic; 20.5 Pallet; 20.6 Avout; 20.7 Clojure on Heroku; Colophon;From the B&N Reads Blog
Page 1 of