Java for Programmers / Edition 2 available in Paperback

Java for Programmers / Edition 2
- ISBN-10:
- 0132821540
- ISBN-13:
- 9780132821544
- Pub. Date:
- 05/02/2011
- Publisher:
- Prentice Hall
- ISBN-10:
- 0132821540
- ISBN-13:
- 9780132821544
- Pub. Date:
- 05/02/2011
- Publisher:
- Prentice Hall

Java for Programmers / Edition 2
Buy New
$59.99Buy Used
$21.63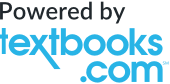
-
SHIP THIS ITEM— This item is available online through Marketplace sellers.
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
This item is available online through Marketplace sellers.
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
This item is available online through Marketplace sellers.
Overview
- Classes, Objects, Encapsulation, Inheritance, Polymorphism, Interfaces, Nested Classes
- Integrated OOP Case Studies: Time, GradeBook, Employee
- Industrial-Strength, 95-Page OOD/UML® 2 ATM Case Study
- JavaServer™ Faces, Ajax-Enabled Web Applications, Web Services, Networking
- JDBC™, SQL, Java DB, MySQL®
- Threads and the Concurrency APIs
- I/O, Types, Control Statements, Methods
- Arrays, Generics, Collections
- Exception Handling, Files
- GUI, Graphics, GroupLayout, JDIC
- Using the Debugger and the API Docs
- And more…
- For information on Deitel’s Dive Into® Series corporate training courses offered at customer sites worldwide (or write to deitel@deitel.com)
- Download code examples
- Check out the growing list of programming, Web 2.0, and software-related Resource Centers
- To receive updates for this book, subscribe to the free DEITEL® BUZZ ONLINE e-mail newsletter at www.deitel.com/newsletter/subscribe.html
- Read archived issues of the DEITEL® BUZZ ONLINE
Written for programmers with a background in high-level language programming, this book applies the Deitel signature live-code approach to teaching programming and explores the Java language and Java APIs in depth. The book presents the concepts in the context of fully tested programs, complete with syntax shading, code highlighting, line-by-line code descriptions and program outputs. The book features 220 Java applications with over 18,000 lines of proven Java code, and hundreds of tips that will help you build robust applications.
Start with an introduction to Java using an early classes and objects approach, then rapidly move on to more advanced topics, including GUI, graphics, exception handling, generics, collections, JDBC™, web-application development with JavaServer™ Faces, web services and more. You’ll enjoy the Deitels’ classic treatment of object-oriented programming and the OOD/UML® ATM case study, including a complete Java implementation. When you’re finished, you’ll have everything you need to build object-oriented Java applications.
The DEITEL® Developer Series is designed for practicing programmers. The series presents focused treatments of emerging technologies, including Java™, C++, .NET, web services, Internet and web development and more.
PRE-PUBLICATION REVIEWER TESTIMONIALS
“Presenting software engineering side by side with core Java concepts is highly refreshing; gives readers insight into how professional software is developed.”—Clark Richey (Java Champion), RABA Technologies, LLC.
“The quality of the design and code examples is second to none!”—Terrell Hull, Enterprise Architect
“The JDBC chapter is very hands on. I like the fact that Java DB/Apache Derby is used in the examples, which makes it really simple to learn and understand JDBC.”—Sandeep Konchady, Sun Microsystems
“Equips you with the latest web application technologies. Examples are impressive and real! Want to develop a simple address locator with Ajax and JSF? Jump to Chapter 22.”—Vadiraj Deshpande, Sun Microsystems
“Covers web services with Java SE 6 and Java EE 5 in a real-life, example-based, friendly approach. The Deitel Web Services Resource Center is really good, even for advanced developers.”—Sanjay Dhamankar, Sun Microsystems
“Mandatory book for any serious Java EE developer looking for improved productivity: JSF development, visual web development and web services development have never been easier.”—Ludovic Chapenois, Sun Microsystems
“I teach Java programming and object-oriented analysis and design. The OOD/UML 2 case study is the best presentation of the ATM example I have seen.”—Craig W. Slinkman, University of Texas–Arlington
“Introduces OOP and UML 2 early. The conceptual level is perfect. No other book comes close to its quality of organization and presentation. The live-code approach to presenting exemplary code makes a big difference in the learning outcome.”—Walt Bunch, Chapman University/
Product Details
ISBN-13: | 9780132821544 |
---|---|
Publisher: | Prentice Hall |
Publication date: | 05/02/2011 |
Edition description: | Second Edition |
Pages: | 1168 |
Product dimensions: | 6.90(w) x 9.00(h) x 1.60(d) |
About the Author
Paul Deitel and Harvey Deitel are the founders of Deitel & Associates, Inc., the internationally recognized programming languages authoring and corporate-training organization. Millions of people worldwide have used Deitel books to master Java, C#, C++, C, iPhone app development, Internet and web programming, JavaScript, XML, Visual Basic®, Visual C++®, Perl, Python and more.
Table of Contents
Preface xxiBefore You Begin xxix
Chapter 1: Introduction 1
1.1 Introduction 2
1.2 Introduction to Object Technology 2
1.3 Open Source Software 5
1.4 Java and a Typical Java Development Environment 7
1.5 Test-Driving a Java Application 11
1.6 Web 2.0: Going Social 15
1.7 Software Technologies 18
1.8 Keeping Up to Date with Information Technologies 20
1.9 Wrap-Up 21
Chapter 2: Introduction to Java Applications 22
2.1 Introduction 23
2.2 Your First Program in Java: Printing a Line of Text 23
2.3 Modifying Your First Java Program 27
2.4 Displaying Text with printf 29
2.5 Another Application: Adding Integers 30
2.6 Arithmetic 34
2.7 Decision Making: Equality and Relational Operators 35
2.8 Wrap-Up 38
Chapter 3: Introduction to Classes, Objects, Methods and Strings 39
3.1 Introduction 40
3.2 Declaring a Class with a Method and Instantiating an Object of a Class 40
3.3 Declaring a Method with a Parameter 44
3.4 Instance Variables, set Methods and get Methods 47
3.5 Primitive Types vs. Reference Types 52
3.6 Initializing Objects with Constructors 53
3.7 Floating-Point Numbers and Type double 56
3.8 Wrap-Up 60
Chapter 4: Control Statements: Part 1 61
4.1 Introduction 62
4.2 Control Structures 62
4.3 if Single-Selection Statement 64
4.4 if…else Double-Selection Statement 65
4.5 while Repetition Statement 68
4.6 Counter-Controlled Repetition 70
4.7 Sentinel-Controlled Repetition 73
4.8 Nested Control Statements 78
4.9 Compound Assignment Operators 81
4.10 Increment and Decrement Operators 82
4.11 Primitive Types 85 4.12 Wrap-Up 85
Chapter 5: Control Statements: Part 2 86
5.1 Introduction 87
5.2 Essentials of Counter-Controlled Repetition 87
5.3 for Repetition Statement 89
5.4 Examples Using the for Statement 92
5.5 do…while Repetition Statement 96
5.6 switch Multiple-Selection Statement 98
5.7 break and continue Statements 105
5.8 Logical Operators 107
5.9 Wrap-Up 113
Chapter 6: Methods: A Deeper Look 114
6.1 Introduction 115
6.2 Program Modules in Java 115
6.3 static Methods, static Fields and Class Math 115
6.4 Declaring Methods with Multiple Parameters 118
6.5 Notes on Declaring and Using Methods 121
6.6 Argument Promotion and Casting 122
6.7 Java API Packages 123
6.8 Case Study: Random-Number Generation 125
6.9 Case Study: A Game of Chance; Introducing Enumerations 130
6.10 Scope of Declarations 134
6.11 Method Overloading 137
6.12 Wrap-Up 139
Chapter 7: Arrays and ArrayLists 140
7.1 Introduction 141
7.2 Arrays 141
7.3 Declaring and Creating Arrays 143
7.4 Examples Using Arrays 144
7.5 Case Study: Card Shuffling and Dealing Simulation 153
7.6 Enhanced for Statement 157
7.7 Passing Arrays to Methods 159
7.8 Case Study: Class GradeBook Using an Array to Store Grades 162
7.9 Multidimensional Arrays 167
7.10 Case Study: Class GradeBook Using a Two-Dimensional Array 171
7.11 Variable-Length Argument Lists 177
7.12 Using Command-Line Arguments 178
7.13 Class Arrays 180
7.14 Introduction to Collections and Class ArrayList 183
7.15 Wrap-Up 186
Chapter 8: Classes and Objects: A Deeper Look 187
8.1 Introduction 188
8.2 Time Class Case Study 188
8.3 Controlling Access to Members 192
8.4 Referring to the Current Object’s Members with the this Reference 193
8.5 Time Class Case Study: Overloaded Constructors 195
8.6 Default and No-Argument Constructors 201
8.7 Notes on Set and Get Methods 202
8.8 Composition 203
8.9 Enumerations 206
8.10 Garbage Collection and Method finalize 209
8.11 static Class Members 210
8.12 static Import 213
8.13 final Instance Variables 214
8.14 Time Class Case Study: Creating Packages 215
8.15 Package Access 221
8.16 Wrap-Up 222
Chapter 9: Object-Oriented Programming: Inheritance 224
9.1 Introduction 225
9.2 Superclasses and Subclasses 226
9.3 protected Members 228
9.4 Relationship between Superclasses and Subclasses 228
9.5 Constructors in Subclasses 250
9.6 Software Engineering with Inheritance 251
9.7 Class Object 252
9.8 Wrap-Up 253
Chapter 10: Object-Oriented Programming: Polymorphism 254
10.1 Introduction 255
10.2 Polymorphism Examples 257
10.3 Demonstrating Polymorphic Behavior 258
10.4 Abstract Classes and Methods 260
10.5 Case Study: Payroll System Using Polymorphism 262
10.6 final Methods and Classes 278
10.7 Case Study: Creating and Using Interfaces 279
10.8 Wrap-Up 290
Chapter 11: Exception Handling: A Deeper Look 292
11.1 Introduction 293
11.2 Example: Divide by Zero without Exception Handling 293
11.3 Example: Handling ArithmeticExceptions and InputMismatchExceptions 296
11.4 When to Use Exception Handling 301
11.5 Java Exception Hierarchy 301
11.6 finally Block 304
11.7 Stack Unwinding and Obtaining Information from an Exception Object 308
11.8 Chained Exceptions 311
11.9 Declaring New Exception Types 313
11.10 Preconditions and Postconditions 314
11.11 Assertions 315
11.12 (New in Java SE 7) Multi-catch: Handling Multiple Exceptions in One catch 316
11.13 (New in Java SE 7) try-with-Resources: Automatic Resource Deallocation 316
11.14 Wrap-Up 317
Chapter 12: ATM Case Study, Part 1: Object-Oriented Design with the UML 318
12.1 Case Study Introduction 319
12.2 Examining the Requirements Document 319
12.3 Identifying the Classes in a Requirements Document 327
12.4 Identifying Class Attributes 333
12.5 Identifying Objects’ States and Activities 338
12.6 Identifying Class Operations 342
12.7 Indicating Collaboration Among Objects 348
12.8 Wrap-Up 355
Chapter 13: ATM Case Study Part 2: Implementing an Object-Oriented Design 359
13.1 Introduction 3 60
13.2 Starting to Program the Classes of the ATM System 360
13.3 Incorporating Inheritance and Polymorphism into the ATM System 365
13.4 ATM Case Study Implementation 371
13.5 Wrap-Up 395
Chapter 14: GUI Components: Part 1 398
14.1 Introduction 399
14.2 Java’s New Nimbus Look-and-Feel 400
14.3 Simple GUI-Based Input/Output with JOptionPane 401
14.4 Overview of Swing Components 404
14.5 Displaying Text and Images in a Window 406
14.6 Text Fields and an Introduction to Event Handling with Nested Classes 410
14.7 Common GUI Event Types and Listener Interfaces 416
14.8 How Event Handling Works 418
14.9 JButton 420
14.10 Buttons That Maintain State 423
14.11 JComboBox; Using an Anonymous Inner Class for Event Handling 429
14.12 JList 433
14.13 Multiple-Selection Lists 435
14.14 Mouse Event Handling 438
14.15 Adapter Classes 443
14.16 JPanel Subclass for Drawing with the Mouse 446
14.17 Key Event Handling 450
14.18 Introduction to Layout Managers 453
14.19 Using Panels to Manage More Complex Layouts 462
14.20 JTextArea 464
14.21 Wrap-Up 467
Chapter 15: Graphics and Java 2D 468
15.1 Introduction 469
15.2 Graphics Contexts and Graphics Objects 471
15.3 Color Control 472
15.4 Manipulating Fonts 479
15.5 Drawing Lines, Rectangles and Ovals 484
15.6 Drawing Arcs 488
15.7 Drawing Polygons and Polylines 491
15.8 Java 2D API 494
15.9 Wrap-Up 501
Chapter 16: Strings, Characters and Regular Expressions 502
16.1 Introduction 503
16.2 Fundamentals of Characters and Strings 503
16.3 Class String 504
16.4 Class StringBuilder 517
16.5 Class Character 524
16.6 Tokenizing Strings 529
16.7 Regular Expressions, Class Pattern and Class Matcher 530
16.8 Wrap-Up 538
Chapter 17: Files, Streams and Object Serialization 539
17.1 Introduction 540
17.2 Files and Streams 540
17.3 Class File 542
17.4 Sequential-Access Text Files 546
17.5 Object Serialization 562
17.6 Additional java.io Classes 57117.7 Opening Files with JFileChooser 574
17.8 Wrap-Up 577
Chapter 18: Generic Collections 578
18.1 Introduction 579
18.2 Collections Overview 579
18.3 Type-Wrapper Classes for Primitive Types 580
18.4 Autoboxing and Auto-Unboxing 581
18.5 Interface Collection and Class Collections 581
18.6 Lists 582
18.7 Collections Methods 590
18.8 Stack Class of Package java.util 602
18.9 Class PriorityQueue and Interface Queue 604
18.10 Sets 605
18.11 Maps 608
18.12 Properties Class 612
18.13 Synchronized Collections 615
18.14 Unmodifiable Collections 615
18.15 Abstract Implementations 616
18.16 Wrap-Up 616
Chapter 19: Generic Classes and Methods 618
19.1 Introduction 619
19.2 Motivation for Generic Methods 619
19.3 Generic Methods: Implementation and Compile-Time Translation 622
19.4 Additional Compile-Time Translation Issues: Methods That Use a Type Parameter as the Return Type 625
19.5 Overloading Generic Methods 628
19.6 Generic Classes 628
19.7 Raw Types 636
19.8 Wildcards in Methods That Accept Type Parameters 640
19.9 Generics and Inheritance: Notes 644
19.10 Wrap-Up 645
Chapter 20: Applets and Java Web Start 646
20.1 Introduction 647
20.2 Sample Applets Provided with the JDK 648
20.3 Simple Java Applet: Drawing a String 652
20.4 Applet Life-Cycle Methods 656
20.5 Initialization with Method init 657
20.6 Sandbox Security Model 659
20.7 Java Web Start and the Java Network Launch Protocol (JNLP) 661
20.8 Wrap-Up 666
Chapter 21: Multimedia: Applets and Applications 667
21.1 Introduction 668
21.2 Loading, Displaying and Scaling Images 669
21.3 Animating a Series of Images 675
21.4 Image Maps 682
21.5 Loading and Playing Audio Clips 685
21.6 Playing Video and Other Media with Java Media Framework 688
21.7 Wrap-Up 692
21.8 Web Resources 692
Chapter 22: GUI Components: Part 2 694
22.1 Introduction 695
22.2 JSlider 695
22.3 Windows: Additional Notes 699
22.4 Using Menus with Frames 700
22.5 JPopupMenu 708
22.6 Pluggable Look-and-Feel 711
22.7 JDesktopPane and JInternalFrame 716
22.8 JTabbedPane 720
22.9 Layout Managers: BoxLayout and GridBagLayout 722
22.10 Wrap-Up 734
Chapter 23: Multithreading 735
23.1 Introduction 736
23.2 Thread States: Life Cycle of a Thread 738
23.3 Creating and Executing Threads with Executor Framework 741
23.4 Thread Synchronization 744
23.5 Producer/Consumer Relationship without Synchronization 752
23.6 Producer/Consumer Relationship: ArrayBlockingQueue 760
23.7 Producer/Consumer Relationship with Synchronization 763
23.8 Producer/Consumer Relationship: Bounded Buffers 769
23.9 Producer/Consumer Relationship: The Lock and Condition Interfaces 776
23.10 Concurrent Collections Overview 783
23.11 Multithreading with GUI 785
23.12 Interfaces Callable and Future 799
23.13 Java SE 7: Fork/Join Framework 799
23.14 Wrap-Up 800
Chapter 24: Networking 801
24.1 Introduction 802
24.2 Manipulating URLs 803
24.3 Reading a File on a Web Server 808
24.4 Establishing a Simple Server Using Stream Sockets 811
24.5 Establishing a Simple Client Using Stream Sockets 813
24.6 Client/Server Interaction with Stream Socket Connections 813
24.7 Datagrams: Connectionless Client/Server Interaction 825
24.8 Client/Server Tic-Tac-Toe Using a Multithreaded Server 833
24.9 [Web Bonus] Case Study: DeitelMessenger 848
24.10 Wrap-Up 848
Chapter 25: Accessing Databases with JDBC 849
25.1 Introduction 850
25.2 Relational Databases 851
25.3 Relational Database Overview: The books Database 852
25.4 SQL 855
25.5 Instructions for Installing MySQL and MySQL Connector/J 864
25.6 Instructions for Setting Up a MySQL User Account 865
25.7 Creating Database books in MySQL 866
25.8 Manipulating Databases with JDBC 867
25.9 RowSet Interface 885
25.10 Java DB/Apache Derby 887
25.11 PreparedStatements 889
25.12 Stored Procedures 904
25.13 Transaction Processing 905
25.14 Wrap-Up 905
25.15 Web Resources 906
Chapter 26: JavaServer™ Faces Web Apps: Part 1 907
26.1 Introduction 908
26.2 HyperText Transfer Protocol (HTTP) Transactions 909
26.3 Multitier Application Architecture 912
26.4 Your First JSF Web App 913
26.5 Model-View-Controller Architecture of JSF Apps 922
26.6 Common JSF Components 922
26.7 Validation Using JSF Standard Validators 926
26.8 Session Tracking 933
26.9 Wrap-Up 941
Chapter 27: JavaServer™ Faces Web Apps: Part 2 942
27.1 Introduction 943
27.2 Accessing Databases in Web Apps 943
27.3 Ajax 956
27.4 Adding Ajax Functionality to the Validation App 958
27.5 Wrap-Up 961
Chapter 28: Web Services 962
28.1 Introduction 963
28.2 Web Service Basics 965
28.3 Simple Object Access Protocol (SOAP) 965
28.4 Representational State Transfer (REST) 965
28.5 JavaScript Object Notation (JSON) 966
28.6 Publishing and Consuming SOAP-Based Web Services 966
28.7 Publishing and Consuming REST-Based XML Web Services 978
28.8 Publishing and Consuming REST-Based JSON Web Services 983
28.9 Session Tracking in a SOAP Web Service 987
28.10 Consuming a Database-Driven SOAP Web Service 1002
28.11 Equation Generator: Returning User-Defined Types 1009
28.12 Wrap-Up 1020
Appendix A: Operator Precedence Chart 1022
Appendix B: ASCII Character Set 1024
Appendix C: Keywords and Reserved Words 1025
Appendix D: Primitive Types 1026
Appendix E: Using the Java API Documentation 1027
E.1 Introduction 1027
E.2 Navigating the Java API 1028
Appendix F: Using the Debugger 1036
F.1 Introduction 1037
F.2 Breakpoints and the run, stop, cont and print Commands 1037
F.3 The print and set Commands 1041
F.4 Controlling Execution Using the step, step up and next Commands 1043
F.5 The watch Command 1046
F.6 The clear Command 1049
F.7 Wrap-Up 1051
Appendix G: Formatted Output 1052
G.1 Introduction 1053
G.2 Streams 1053
G.3 Formatting Output with printf 1053
G.4 Printing Integers 1054
G.5 Printing Floating-Point Numbers 1055
G.6 Printing Strings and Characters 1057
G.7 Printing Dates and Times 1058
G.8 Other Conversion Characters 1060
G.9 Printing with Field Widths and Precisions 1062
G.10 Using Flags in the printf Format String 1064
G.11 Printing with Argument Indices 1068
G.12 Printing Literals and Escape Sequences 1068
G.13 Formatting Output with Class Formatter 1069
G.14 Wrap-Up 1070
Appendix H: GroupLayout 1071
H.1 Introduction 1071
H.2 GroupLayout Basics 1071
H.3 Building a ColorChooser 1072
H.4 GroupLayout Web Resources 1082
Appendix I: Java Desktop Integration Components 1083
I.1 Introduction 1083
I.2 Splash Screens 1083
I.3 Desktop Class 1085
I.4 Tray Icons 1087
Appendix J: UML 2: Additional Diagram Types 1089
J.1 Introduction 1089
J.2 Additional Diagram Types 1089
Index 1091