Author and independent consultant Joe Mayo shares some of the most important practices you'll need to be successful as a C# developer. Each section of this cookbook describes some useful facet of the C# programming language. These recipesthe result of many years of experienceare proven concepts for solving real-world problems with C#.
Recipes in this book will help you:
- Set up your project, manage object lifetime, and establish patterns
- Improve code quality through maintainability, error prevention, and correct syntax
- Use LINQ to Objects for in-memory data manipulation and querying
- Understand the differences between dynamic programming and reflection
- Apply several async programming features you may not be aware of
- Work with data using newer libraries and algorithms
- Learn different ways to use new C# features, such as pattern matching and records
Author and independent consultant Joe Mayo shares some of the most important practices you'll need to be successful as a C# developer. Each section of this cookbook describes some useful facet of the C# programming language. These recipesthe result of many years of experienceare proven concepts for solving real-world problems with C#.
Recipes in this book will help you:
- Set up your project, manage object lifetime, and establish patterns
- Improve code quality through maintainability, error prevention, and correct syntax
- Use LINQ to Objects for in-memory data manipulation and querying
- Understand the differences between dynamic programming and reflection
- Apply several async programming features you may not be aware of
- Work with data using newer libraries and algorithms
- Learn different ways to use new C# features, such as pattern matching and records
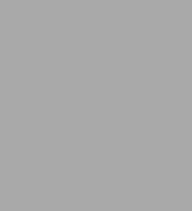
C# Cookbook: Modern Recipes for Professional Developers
325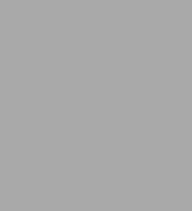
C# Cookbook: Modern Recipes for Professional Developers
325Product Details
ISBN-13: | 9781492093695 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 11/02/2021 |
Pages: | 325 |
Product dimensions: | 7.00(w) x 9.19(h) x 0.69(d) |