As a Java programmer, how can you tackle the disruptive client-server approach to web development? With this comprehensive guide, you’ll learn how today’s client-side technologies and web APIs work with various Java tools. Author Casimir Saternos provides the big picture of client-server development, and then takes you through many practical client-server architectures. You’ll work with hands-on projects in several chapters to get a feel for the topics discussed.
User habits, technologies, and development methods have drastically altered web app design in recent years. But the Web itself hasn’t changed. This book shows you how to build apps that conform to the web’s underlying architecture.
- Learn the advantages of using separate client and server tiers, including code organization and speedy prototyping
- Explore the major tools, frameworks, and starter projects used in JavaScript development
- Dive into web API design and REST style of software architecture
- Understand Java’s alternatives to traditional packaging methods and application server deployment
- Build projects with lightweight servers, using jQuery with Jython, and Sinatra with Angular
- Create client-server web apps with traditional Java web application servers and libraries
As a Java programmer, how can you tackle the disruptive client-server approach to web development? With this comprehensive guide, you’ll learn how today’s client-side technologies and web APIs work with various Java tools. Author Casimir Saternos provides the big picture of client-server development, and then takes you through many practical client-server architectures. You’ll work with hands-on projects in several chapters to get a feel for the topics discussed.
User habits, technologies, and development methods have drastically altered web app design in recent years. But the Web itself hasn’t changed. This book shows you how to build apps that conform to the web’s underlying architecture.
- Learn the advantages of using separate client and server tiers, including code organization and speedy prototyping
- Explore the major tools, frameworks, and starter projects used in JavaScript development
- Dive into web API design and REST style of software architecture
- Understand Java’s alternatives to traditional packaging methods and application server deployment
- Build projects with lightweight servers, using jQuery with Jython, and Sinatra with Angular
- Create client-server web apps with traditional Java web application servers and libraries
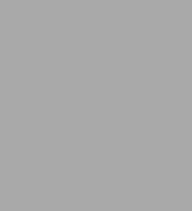
Client-Server Web Apps with JavaScript and Java: Rich, Scalable, and RESTful
260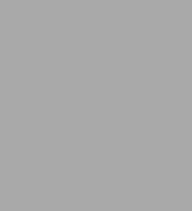
Client-Server Web Apps with JavaScript and Java: Rich, Scalable, and RESTful
260Product Details
ISBN-13: | 9781449369293 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 03/28/2014 |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 260 |
File size: | 4 MB |