In this text, readers are able to look at specific problems and see how careful implementations can reduce the time constraint for large amounts of data from several years to less than a second.
This new edition contains all the enhancements of the new Java 5.0 code including detailed examples and an implementation of a large subset of the Java 5.0 Collections API.
This text is for readers who want to learn good programming and algorithm analysis skills simultaneously so that they can develop such programs with the maximum amount of efficiency. Readers should have some knowledge of intermediate programming, including topics as object-based programming and recursion, and some background in discrete math.
In this text, readers are able to look at specific problems and see how careful implementations can reduce the time constraint for large amounts of data from several years to less than a second.
This new edition contains all the enhancements of the new Java 5.0 code including detailed examples and an implementation of a large subset of the Java 5.0 Collections API.
This text is for readers who want to learn good programming and algorithm analysis skills simultaneously so that they can develop such programs with the maximum amount of efficiency. Readers should have some knowledge of intermediate programming, including topics as object-based programming and recursion, and some background in discrete math.
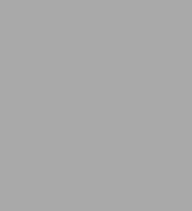
Data Structures and Algorithm Analysis in Java
576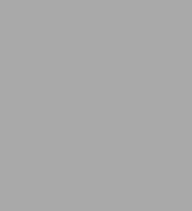
Data Structures and Algorithm Analysis in Java
576Paperback(REV)
Product Details
ISBN-13: | 9780321370136 |
---|---|
Publisher: | Addison Wesley |
Publication date: | 03/03/2006 |
Edition description: | REV |
Pages: | 576 |
Product dimensions: | 7.73(w) x 9.55(h) x 0.99(d) |