Multithreading is essential if you want to create an Android app with a great user experience, but how do you know which techniques can help solve your problem? This practical book describes many asynchronous mechanisms available in the Android SDK, and provides guidelines for selecting the ones most appropriate for the app you’re building.
Author Anders Goransson demonstrates the advantages and disadvantages of each technique, with sample code and detailed explanations for using it efficiently. The first part of the book describes the building blocks of asynchronous processing, and the second part covers Android libraries and constructs for developing fast, responsive, and well-structured apps.
- Understand multithreading basics in Java and on the Android platform
- Learn how threads communicate within and between processes
- Use strategies to reduce the risk of memory leaks
- Manage the lifecycle of a basic thread
- Run tasks sequentially in the background with HandlerThread
- Use Java’s Executor Framework to control or cancel threads
- Handle background task execution with AsyncTask and IntentService
- Access content providers with AsyncQueryHandler
- Use loaders to update the UI with new data
Multithreading is essential if you want to create an Android app with a great user experience, but how do you know which techniques can help solve your problem? This practical book describes many asynchronous mechanisms available in the Android SDK, and provides guidelines for selecting the ones most appropriate for the app you’re building.
Author Anders Goransson demonstrates the advantages and disadvantages of each technique, with sample code and detailed explanations for using it efficiently. The first part of the book describes the building blocks of asynchronous processing, and the second part covers Android libraries and constructs for developing fast, responsive, and well-structured apps.
- Understand multithreading basics in Java and on the Android platform
- Learn how threads communicate within and between processes
- Use strategies to reduce the risk of memory leaks
- Manage the lifecycle of a basic thread
- Run tasks sequentially in the background with HandlerThread
- Use Java’s Executor Framework to control or cancel threads
- Handle background task execution with AsyncTask and IntentService
- Access content providers with AsyncQueryHandler
- Use loaders to update the UI with new data
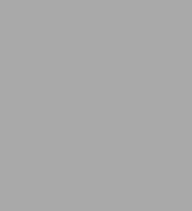
Efficient Android Threading: Asynchronous Processing Techniques for Android Applications
280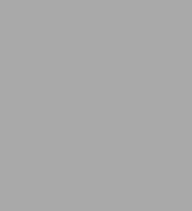
Efficient Android Threading: Asynchronous Processing Techniques for Android Applications
280Product Details
ISBN-13: | 9781449364090 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 05/22/2014 |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 280 |
File size: | 4 MB |