Summary
Functional Programming in JavaScript teaches JavaScript developers functional techniques that will improve extensibility, modularity, reusability, testability, and performance. Through concrete examples and jargon-free explanations, this book teaches you how to apply functional programming to real-life development tasks
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
In complex web applications, the low-level details of your JavaScript code can obscure the workings of the system as a whole. As a coding style, functional programming (FP) promotes loosely coupled relationships among the components of your application, making the big picture easier to design, communicate, and maintain.
About the Book
Functional Programming in JavaScript teaches you techniques to improve your web applications - their extensibility, modularity, reusability, and testability, as well as their performance. This easy-to-read book uses concrete examples and clear explanations to show you how to use functional programming in real life. If you're new to functional programming, you'll appreciate this guide's many insightful comparisons to imperative or object-oriented programming that help you understand functional design. By the end, you'll think about application design in a fresh new way, and you may even grow to appreciate monads!
What's Inside
About the Reader
Written for developers with a solid grasp of JavaScript fundamentals and web application design.
About the Author
Luis Atencio is a software engineer and architect building enterprise applications in Java, PHP, and JavaScript.
Table of Contents
1123921923
Functional Programming in JavaScript teaches JavaScript developers functional techniques that will improve extensibility, modularity, reusability, testability, and performance. Through concrete examples and jargon-free explanations, this book teaches you how to apply functional programming to real-life development tasks
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
In complex web applications, the low-level details of your JavaScript code can obscure the workings of the system as a whole. As a coding style, functional programming (FP) promotes loosely coupled relationships among the components of your application, making the big picture easier to design, communicate, and maintain.
About the Book
Functional Programming in JavaScript teaches you techniques to improve your web applications - their extensibility, modularity, reusability, and testability, as well as their performance. This easy-to-read book uses concrete examples and clear explanations to show you how to use functional programming in real life. If you're new to functional programming, you'll appreciate this guide's many insightful comparisons to imperative or object-oriented programming that help you understand functional design. By the end, you'll think about application design in a fresh new way, and you may even grow to appreciate monads!
What's Inside
- High-value FP techniques for real-world uses
- Using FP where it makes the most sense
- Separating the logic of your system from implementation details
- FP-style error handling, testing, and debugging
- All code samples use JavaScript ES6 (ES 2015)
About the Reader
Written for developers with a solid grasp of JavaScript fundamentals and web application design.
About the Author
Luis Atencio is a software engineer and architect building enterprise applications in Java, PHP, and JavaScript.
Table of Contents
- PART 1 THINK FUNCTIONALLY
- Becoming functional
- Higher-order JavaScript PART 2 GET FUNCTIONAL
- Few data structures, many operations
- Toward modular, reusable code
- Design patterns against complexity PART 3 ENHANCING YOUR FUNCTIONAL SKILLS
- Bulletproofing your code
- Functional optimizations
- Managing asynchronous events and data
Functional Programming in JavaScript: How to improve your JavaScript programs using functional techniques
Summary
Functional Programming in JavaScript teaches JavaScript developers functional techniques that will improve extensibility, modularity, reusability, testability, and performance. Through concrete examples and jargon-free explanations, this book teaches you how to apply functional programming to real-life development tasks
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
In complex web applications, the low-level details of your JavaScript code can obscure the workings of the system as a whole. As a coding style, functional programming (FP) promotes loosely coupled relationships among the components of your application, making the big picture easier to design, communicate, and maintain.
About the Book
Functional Programming in JavaScript teaches you techniques to improve your web applications - their extensibility, modularity, reusability, and testability, as well as their performance. This easy-to-read book uses concrete examples and clear explanations to show you how to use functional programming in real life. If you're new to functional programming, you'll appreciate this guide's many insightful comparisons to imperative or object-oriented programming that help you understand functional design. By the end, you'll think about application design in a fresh new way, and you may even grow to appreciate monads!
What's Inside
About the Reader
Written for developers with a solid grasp of JavaScript fundamentals and web application design.
About the Author
Luis Atencio is a software engineer and architect building enterprise applications in Java, PHP, and JavaScript.
Table of Contents
Functional Programming in JavaScript teaches JavaScript developers functional techniques that will improve extensibility, modularity, reusability, testability, and performance. Through concrete examples and jargon-free explanations, this book teaches you how to apply functional programming to real-life development tasks
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
In complex web applications, the low-level details of your JavaScript code can obscure the workings of the system as a whole. As a coding style, functional programming (FP) promotes loosely coupled relationships among the components of your application, making the big picture easier to design, communicate, and maintain.
About the Book
Functional Programming in JavaScript teaches you techniques to improve your web applications - their extensibility, modularity, reusability, and testability, as well as their performance. This easy-to-read book uses concrete examples and clear explanations to show you how to use functional programming in real life. If you're new to functional programming, you'll appreciate this guide's many insightful comparisons to imperative or object-oriented programming that help you understand functional design. By the end, you'll think about application design in a fresh new way, and you may even grow to appreciate monads!
What's Inside
- High-value FP techniques for real-world uses
- Using FP where it makes the most sense
- Separating the logic of your system from implementation details
- FP-style error handling, testing, and debugging
- All code samples use JavaScript ES6 (ES 2015)
About the Reader
Written for developers with a solid grasp of JavaScript fundamentals and web application design.
About the Author
Luis Atencio is a software engineer and architect building enterprise applications in Java, PHP, and JavaScript.
Table of Contents
- PART 1 THINK FUNCTIONALLY
- Becoming functional
- Higher-order JavaScript PART 2 GET FUNCTIONAL
- Few data structures, many operations
- Toward modular, reusable code
- Design patterns against complexity PART 3 ENHANCING YOUR FUNCTIONAL SKILLS
- Bulletproofing your code
- Functional optimizations
- Managing asynchronous events and data
34.99
In Stock
5
1
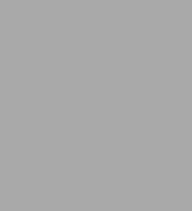
Functional Programming in JavaScript: How to improve your JavaScript programs using functional techniques
272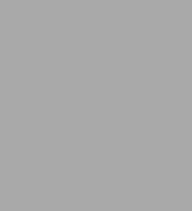
Functional Programming in JavaScript: How to improve your JavaScript programs using functional techniques
272Related collections and offers
34.99
In Stock
From the B&N Reads Blog