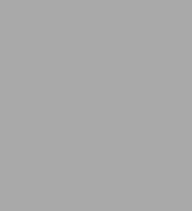
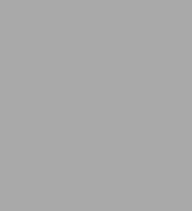
Paperback(3rd ed.)
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
Head First Java is a complete learning experience in Java and object-oriented programming. With this book, you'll learn the Java language with a unique method that goes beyond how-to manuals and helps you become a great programmer. Through puzzles, mysteries, and soul-searching interviews with famous Java objects, you'll quickly get up to speed on Java's fundamentals and advanced topics including lambdas, streams, generics, threading, networking, and the dreaded desktop GUI. If you have experience with another programming language, Head First Java will engage your brain with more modern approaches to codingthe sleeker, faster, and easier to read, write, and maintain Java of today.
What's so special about this book?
If you've read a Head First book, you know what to expecta visually rich format designed for the way your brain works. If you haven't, you're in for a treat. With Head First Java, you'll learn Java through a multisensory experience that engages your mind, rather than by means of a text-heavy approach that puts you to sleep.
Product Details
ISBN-13: | 9781491910771 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 06/21/2022 |
Edition description: | 3rd ed. |
Pages: | 752 |
Sales rank: | 361,161 |
Product dimensions: | 8.00(w) x 9.25(h) x (d) |
About the Author
In 2015, she won the Electronic Frontier Foundation's Pioneer Award for her work creating skillful users and building sustainable communities. Kathy's recent focus has been on cutting-edge, movement science and skill acquisition coaching, known as ecological dynamics or Eco-D. Her work using Eco-D for training horses is ushering in a far, far more humane approach to horsemanship, causing delight for some (and sadly, consternation for others). Those fortunate (autonomous!) horses whose owners are using Kathy's approach are happier, healthier, and more athletic than their fellows who are traditionally trained.You can follow Kathy on Instagram @pantherflows
Before Bert was an author, he was a developer, specializing in old-school AI (mostly expert systems), real-time OSes, and complex scheduling systems. In 2003, Bert and Kathy wrote Head First Java and launched the Head First series. Since then, he's written more Java books and consulted with Sun Microsystems and Oracle on many of their Java certifications. He trains authors and editors to create books that teach well. Of the hundreds of authors he's trained, many have gone on to write best-selling books. Bert's a Go player, and in 2016 he watched in horror and fascination as AlphaGo trounced Lee Sedol. Recently he's been using Eco-D (ecological dynamics) to improve his golf game and to train his parrotlet Bokeh. You can email Bert at CodeRanch.com.
Trisha Gee is a Java Champion, published author, and leader of the Java developer advocacy team at JetBrains. She’s developed Java applications for companies of all sizes in the finance, manufacturing, and nonprofit industries and beyond. Trisha has expertise in Java high-performance systems, dabbles with open source development, and is a leader of the Sevilla Java User Group.
Table of Contents
Intro: Your brain on Java | ||
Who is this book for? | xx | |
What your brain is thinking | xxi | |
Metacognition | xxiii | |
Bend your brain into submission | xxv | |
What you need for this book | xxvi | |
Technical editors | xxviii | |
Acknowledgements | xxix | |
1 | Breaking the Surface: Java takes you to new places | |
The way Java works | 2 | |
Code structure in Java | 5 | |
Anatomy of a class | 6 | |
The main() method | 7 | |
Looping | 8 | |
Conditional branching (if tests) | 11 | |
Coding the "99 bottles of beer" app | 12 | |
Phrase-o-matic | 14 | |
Fireside chat: compiler vs. JVM | 16 | |
Exercises and puzzles | 18 | |
2 | A Trip to Objectville: I was told there would be objects | |
Chair Wars (Brad the OO guy vs. Larry the procedural guy) | 26 | |
Inheritance (an introduction) | 29 | |
Overriding methods (as introduction) | 30 | |
What's in a class? (methods, instance variables) | 32 | |
Making your first object | 34 | |
Using main() | 36 | |
Guessing Game code | 37 | |
Exercises and puzzles | 40 | |
3 | Know Your Variables: Variables come in two flavors: primitive and reference | |
Declaring a variable (Java cares about type) | 48 | |
Primitive types ("I'd like a double with extra foam, please") | 49 | |
Java keywords | 51 | |
Reference variables (remote control to an object) | 52 | |
Object declaration and assignment | 53 | |
Objects on the garbage-collectible heap | 55 | |
Arrays (a first look) | 57 | |
Exercises and puzzles | 61 | |
4 | How Objects Behave: State affects behavior, behavior affects state | |
Methods use object state (bark different) | 71 | |
Method arguments and return types | 72 | |
Pass-by-value (the variable is always copied) | 75 | |
Getters and Setters | 77 | |
Encapsulation (do it or risk humiliation) | 78 | |
Using references in an array | 81 | |
Exercises and puzzles | 86 | |
5 | Extra-Strength Methods: Let's put some muscle in our methods | |
Building the Sink a Dot Com game | 94 | |
Starting with the Simple Dot Com game (a simpler version) | 96 | |
Writing prepcode (pseudocode for the game) | 97 | |
Test code for Simple Dot Com | 100 | |
Coding the Simple Dot Com game | 101 | |
Final code for Simple Dot Com | 104 | |
Generating random numbers with Math.random() | 109 | |
Ready-bake code for getting user input from the command-line | 110 | |
Looping with for loops | 112 | |
Casting primitives from a large size to a smaller size | 114 | |
Converting a String to an int with Integer.parseInt() | 114 | |
Exercises and puzzles | 115 | |
6 | Using the Java Library: Java ships with hundreds of pre-built classes | |
Analying the bug in the Simple Dot Com Game | 122 | |
ArrayList (taking advantage of the Java API) | 128 | |
Fixing the DotCom class code | 134 | |
Building the real game (Sink a Dot Com) | 136 | |
Prepcode for the real game | 140 | |
Code for the real game | 142 | |
Boolean expressions | 147 | |
Using the library (Java API) | 150 | |
Using packages (import statements, fully-qualified names) | 151 | |
Using the HTML API docs and reference books | 154 | |
Exercises and puzzles | 157 | |
7 | Better Living in Objectville: Plan your programs with the future in mind | |
Understanding inheritance (superclass and subclass relationships) | 164 | |
Designing an inheritance tree (the Animal simulation) | 166 | |
Avoiding duplicate code (using inheritance) | 167 | |
Overriding methods | 168 | |
IS-A and HAS-A (bathtub girl) | 173 | |
What do you inherit from your superclass? | 176 | |
What does inheritance really buy you? | 178 | |
Polymorphism (using a supertype reference to a subclass object) | 179 | |
Rules for overriding (don't touch those arguments and return types!) | 186 | |
Method overloading (nothing more than method name re-use) | 187 | |
Exercises and puzzles | 188 | |
8 | Serious Polymorphism: Inheritance is just the beginning | |
Some classes just should not be instantiated | 196 | |
Abstract classes (can't be instantiated) | 197 | |
Abstract methods (must be implemented) | 199 | |
Polymorphism in action | 202 | |
Class Object (the ultimate superclass of everything) | 204 | |
Taking objects out of an ArrayList (they come out as type Object) | 207 | |
Compiler checks the reference type (before letting you call a method) | 209 | |
Get in touch with your inner object | 210 | |
Polymorphic references | 211 | |
Casting an object reference (moving lower on the inheritance tree) | 212 | |
Deadly Diamond of Death (multiple inheritance problem) | 219 | |
Using interfaces (the best solution!) | 220 | |
Exercises and puzzles | 225 | |
9 | Life and Death of an Object: Objects are born and objects die | |
The stack and the heap, where objects and variables live | 232 | |
Methods on the stack | 233 | |
Where local variables live | 234 | |
Where instance variables live | 235 | |
The miracle of object creation | 236 | |
Constructors (the code that runs when you say new) | 237 | |
Initializing the state of a new Duck | 239 | |
The compiler can make a default (no-arg) constructor | 241 | |
Overloaded constructors | 243 | |
Superclass constructors (constructor chaining) | 246 | |
Invoking overloaded constructors using this() | 252 | |
Life of an object | 254 | |
Garbage Collection (and making objects eligible) | 256 | |
Exercises and puzzles | 262 | |
10 | Numbers Matter: Do the Math | |
Math class (do you really need an instance of it?) | 270 | |
Static methods | 271 | |
Static variables | 273 | |
Constants (static final variables) | 278 | |
Math methods (random(), round(), abs(), etc.) | 282 | |
Wrapper classes (Integer, Boolean, Character, etc.) | 283 | |
Number formatting | 284 | |
Date formatting | 288 | |
Exercises and puzzles | 291 | |
11 | Risky Behavior: Stuff happens | |
Making a music machine (the BeatBox) | 298 | |
What if you need to call risky code? | 301 | |
Exceptions say "something bad may have happened..." | 302 | |
The compiler guarantees (it checks) that you're aware of the risks | 303 | |
Catching exceptions using a try/catch (skateboarder) | 304 | |
Flow control in try/catch blocks | 308 | |
The finally block (no matter what happens, turn off the oven!) | 309 | |
Catching multiple exceptions (the order matters) | 311 | |
Declaring an exception (just duck it) | 317 | |
Handle or declare law | 319 | |
Code Kitchen (making sounds) | 321 | |
Exercises and puzzles | 330 | |
12 | A Very Graphic Story: Face it, you need to make GUIs | |
Your first GUI | 337 | |
Getting a user event | 339 | |
Implement a listener interface | 340 | |
Getting a button's ActionEvent | 342 | |
Putting graphics on a GUI | 345 | |
Fun with paintComponent() | 347 | |
The Graphics2D object | 348 | |
Putting more than one button on a screen | 352 | |
Inner classes to the rescue (make your listener an inner class) | 358 | |
Animation (move it, paint it, move it, paint it, move it, paint it...) | 364 | |
Code Kitchen (painting graphics with the beat of the music) | 368 | |
Exercises and puzzles | 376 | |
13 | Work on your Swing: Swing is easy | |
Swing Components | 382 | |
Layout Managers (they control size and placement) | 383 | |
Three Layout Managers (border, flow, box) | 385 | |
BorderLayout (cares about five regions) | 386 | |
FlowLayout (cares about the order and preferred size) | 390 | |
BoxLayout (like flow, but can stack components vertically) | 393 | |
JTextField (for single-line user input) | 395 | |
JTextArea (for multi-line, scrolling text) | 396 | |
JCheckBox (is it selected?) | 398 | |
JList (a scrollable, selectable list) | 399 | |
Code Kitchen (The Big One - building the BeatBox chat client) | 400 | |
Exercises and puzzles | 406 | |
14 | Saving Objects: Objects can be flattened and inflated | |
Saving object state | 413 | |
Writing a serialized object to a file | 414 | |
Java input and output streams (connections and chains) | 415 | |
Object serialization | 416 | |
Implementing the Serializable interface | 419 | |
Using transient variables | 421 | |
Deserializing an object | 423 | |
Writing to a text file | 427 | |
java.io.File | 432 | |
Reading from a text file | 434 | |
StringTokenizer | 438 | |
CodeKitchen | 442 | |
Exercises and puzzles | 446 | |
15 | Make a Connection: Connect with the outside world | |
Chat program overview | 453 | |
Connecting, sending, and receiving | 454 | |
Network sockets | 455 | |
TCP ports | 456 | |
Reading data from a socket (using BufferedReader) | 458 | |
Writing data to a socket (using PrintWriter) | 459 | |
Writing the Daily Advice Client program | 460 | |
Writing a simple server | 463 | |
Daily Advice Server code | 464 | |
Writing a chat client | 466 | |
Multiple call stacks | 470 | |
Launching a new thread (make it, start it) | 472 | |
The Runnable interface (the thread's job) | 473 | |
Three states of a new Thread object (new, runnable, running) | 475 | |
The runnable-running loop | 476 | |
Thread scheduler (it's his decision, not yours) | 477 | |
Putting a thread to sleep | 481 | |
Making and starting two threads | 483 | |
Concurrency issues: can this couple be saved? | 485 | |
The Ryan and Monica concurrency problem, in code | 486 | |
Locking to make things atomic | 490 | |
Every object has a lock | 491 | |
The dreaded "Lost Update" problem | 492 | |
Synchronized methods (using a lock) | 494 | |
Deadlock! | 496 | |
Multithreaded ChatClient code | 498 | |
Ready-bake SimpleChatServer | 500 | |
Exercises and puzzles | 504 | |
16 | Release Your Code: It's time to let go | |
Deployment options | 510 | |
Keep your source code and class files separate | 512 | |
Making an executable JAR (Java ARchives) | 513 | |
Running an executable JAR | 514 | |
Put your classes in a package! | 515 | |
Preventing package name conflicts | 516 | |
Packages must have a matching directory structure | 517 | |
Compiling and running with packages | 518 | |
Compiling with -d | 519 | |
Making an executable JAR (with packages) | 520 | |
Java Web Start (JWS) for deployment from the web | 525 | |
The jnlp file | 527 | |
How to make and deploy a JWS application | 528 | |
Exercises and puzzles | 529 | |
17 | Distributed Computing: Being remote doesn't have to be a bad thing | |
Java Remote Method Invocation (RMI), hands-on, very detailed | 552 | |
Servlets (a quick look) | 553 | |
Enterprise JavaBeans (EJB), a very quick look | 559 | |
Jini, the best trick of all | 560 | |
Building the really cool universal service browser | 564 | |
The End | 576 | |
A | Appendix A: The final Code Kitchen project | |
BeatBoxFinal (client code) | 578 | |
MusicServer (server code) | 585 | |
B | Appendix B: The Top Ten Things that didn't make it into the book | |
Bit manipulation | 588 | |
Immutability | 589 | |
Assertions | 590 | |
Block scope | 591 | |
Linked invocations | 592 | |
Overriding equals() | 593 | |
Access levels and access modifiers (who sees what) | 594 | |
String and StringBuffer methods | 596 | |
Multidimensional arrays | 597 | |
Collections | 598 | |
Index | 607 |