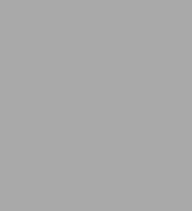
Java Examples in a Nutshell: A Tutorial Companion to Java in a Nutshell
718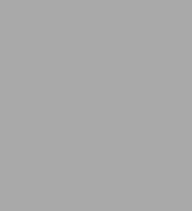
Java Examples in a Nutshell: A Tutorial Companion to Java in a Nutshell
718Paperback(Third Edition)
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
- Core APIs, including I/O, New I/O, threads, networking, security, serialization, and reflection
- Desktop APIs, highlighting Swing GUIs, Java 2D graphics, preferences, printing, drag-and-drop, JavaBeans, applets, and sound
- Enterprise APIs, including JDBC (database access), JAXP (XML parsing and transformation), Servlets 2.4, JSP 2.0 (JavaServer Pages), and RMI
Product Details
ISBN-13: | 9780596006204 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 01/09/2004 |
Series: | In a Nutshell (O'Reilly) |
Edition description: | Third Edition |
Pages: | 718 |
Sales rank: | 729,408 |
Product dimensions: | 5.92(w) x 8.94(h) x 1.24(d) |
About the Author
Read an Excerpt
Chapter 19: XML
Contents:
- Parsing with JAXP and SAX 1
Parsing with SAX 2
Parsing and Manipulating with JAXP and DOM
Traversing a DOM Tree
Traversing a Document with DOM Level 2
The JDOM API
Exercises
XML, or Extensible Markup Language, is a meta-language for marking up text documents with structural tags, similar to those found in HTML and SGML documents. XML has become popular because its structural markup allows documents to describe their own format and contents. XML enables "portable data," and it can be quite powerful when combined with the "portable code" enabled by Java.
Because of the popularity of XML, there are a number of tools for parsing and manipulating XML documents. And because XML documents are becoming more and more common, it is worth your time to learn how to use some of those tools to work with XML. The examples in this chapter introduce you to simple XML parsing and manipulation. If you are familiar with the basic structure of an XML file, you should have no problem understanding them. Note that there are many subtleties to working with XML; this chapter doesn't attempt to explain them all. To learn more about XML, try Java and XML, by Brett McLaughlin, or XML Pocket Reference, by Robert Eckstein, both from O'Reilly & Associates.
The world of XML and its affiliated technologies is moving so fast that it can be hard just keeping up with the acronyms, standards, APIs, and version numbers. I'll try to provide an overview of the state of various technologies in this chapter, but be warned that things may have changed, sometimes radically, by the time you read this material.
Parsing with JAXP and SAX 1
The first thing you want to do with an XML document is parse it. There are two commonly used approaches to XML parsing: they go by the acronyms SAX and DOM. We'll begin with SAX parsing; DOM parsing is covered later in the chapter. At the very end of the chapter, we'll also see a new, but very promising, Java-centric XML API known as JDOM.
SAX is the Simple API for XML. SAX is not a parser, but rather a Java API that describes how a parser operates. When parsing an XML document using the SAX API, you define a class that implements various "event" handling methods. As the parser encounters the various element types of the XML document, it invokes the corresponding event handler methods you've defined. Your methods take whatever actions are required to accomplish the desired task. In the SAX model, the parser converts an XML document into a sequence of Java method calls. The parser doesn't build a parse tree of any kind (although your methods can do this, if you want). SAX parsing is typically quite efficient and is therefore your best choice for most simple XML processing tasks.
The SAX API was created by David Megginson (http://www.megginson.com/SAX/). The Java implementation of the API is in the package org.xml.sax
and its subpackages. SAX is a defacto standard but has not been standardized by any official body. SAX Version 1 has been in use for some time; SAX 2 was finalized in May 2000. There are numerous changes between the SAX 1 and SAX 2 APIs. Many Java-based XML parsers exist that conform to the SAX 1 or SAX 2 APIs.
With the SAX API, you can't completely abstract away the details of the XML parser implementation you are using: at a minimum, your code must supply the classname of the parser to be used. This is where JAXP comes in. JAXP is the Java API for XML Parsing. It is an "optional package" defined by Sun that consists of the javax.xml.parsers
package. JAXP provides a thin layer on top of SAX (and on top of DOM, as we'll see) and standardizes an API for obtaining and using SAX (and DOM) parser objects. The JAXP package ships with default parser implementations but allows other parsers to be easily plugged in and configured using system properties. At this writing, the current version of JAXP is 1.0.1; it supports SAX 1, but not SAX 2. By the time you read this, however, JAXP 1.1, which will include support for SAX 2, may have become available.
Example 19.1 is a listing of ListServlets1.java, a program that uses JAXP and SAX to parse a web application deployment descriptor and list the names of the servlets configured by that file. If you haven't yet read Chapter 18, Servlets and JSP, you should know that servlet-based web applications are configured using an XML file named web.xml. This file contains <servlet>
tags that define mappings between servlet names and the Java classes that implement them. To help you understand the task to be solved by the ListServlets1.java program, here is an excerpt from the web.xml file developed in Chapter 18:
<servlet> </servlet> <servlet> </servlet> <servlet> </servlet>
ListServlets1.java includes a main()
method that uses the JAXP API to obtain a SAX parser instance. It then tells the parser what to parse and starts the parser running. The remaining methods of the class are invoked by the parser. Note that ListServlets1
extends the SAX HandlerBase
class. This superclass provides dummy implementations of all the SAX event handler methods. The example simply overrides the handlers of interest. The parser calls the startElement()
method when it reads an XML tag; it calls endElement()
when it finds a closing tag. characters()
is invoked when the parser reads a string of plain text with no markup. Finally, the parser calls warning()
, error()
, or fatalError()
when something goes wrong in the parsing process. The implementations of these methods are written specifically to extract the desired information from a web.xml file and are based on a knowledge of the structure of this type of file.
Note that web.xml files are somewhat unusual in that they don't rely on attributes for any of the XML tags. That is, servlet names are defined by a <servlet-name>
tag nested within a <servlet>
tag, instead of simply using a name
attribute of the <servlet>
tag itself. This fact makes the example program slightly more complex than it would otherwise be. The web.xml file does allow id
attributes for all its tags. Although servlet engines are not expected to use these attributes, they may be useful to a configuration tool that parses and automatically generates web.xml files. For completeness, the startElement()
method in Example 19.1 looks for an id
attribute of the <servlet>
tag. The value of that attribute, if it exists, is reported in the program's output.
Example 19.1: ListServlets1.java
package com.davidflanagan.examples.xml; import javax.xml.parsers.*; // The JAXP package import org.xml.sax.*; // The main SAX package import java.io.*; /** * Parse a web.xml file using JAXP and SAX1. Print out the names * and class names of all servlets listed in the file. * * This class implements the HandlerBase helper class, which means * that it defines all the "callback" methods that the SAX parser will * invoke to notify the application. In this example we override the * methods that we require. * * This example uses full package names in places to help keep the JAXP * and SAX APIs distinct. **/ public class ListServlets1 extends org.xml.sax.HandlerBase {
Compiling and Running the Example
To run the previous example, you need the JAXP package from Sun. You can download it by following the download links from http://java.sun.com/xml/. Once you've downloaded the package, uncompress the archive it is packaged in and install it somewhere convenient on your system. In Version 1.0.1 of JAXP, the download bundle contains two JAR files: jaxp.jar, the JAXP API classes, and parser.jar, the SAX and DOM APIs and default parser implementations. To compile and run this example, you need both JAR files in your classpath. If you have any other XML parsers, such as the Xerces parser, in your classpath, remove them or make sure that the JAXP files are listed first; otherwise you may run into version-skew problems between the different parsers. Note that you probably don't want to permanently alter your classpath, since you'll have to change it again for the next example. One simple solution with Java 1.2 and later is to temporarily drop copies of the JAXP JAR files into the jre/lib/ext/ directory of your Java installation.
With the two JAXP JAR files temporarily in your classpath, you can compile and run ListServlets1.java as usual. When you run it, specify the name of a web.xml file on the command line. You can use the sample file included with the downloadable examples for this book or specify one from your own servlet engine.
There is one complication to this example. Most web.xml files contain a <!DOCTYPE>
tag that specifies the document type (or DTD). Despite the fact that Example 19.1 specifies that the parser should not validate the document, a conforming XML parser must still read the DTD for any document that has a <!DOCTYPE>
declaration. Most web.xml have a declaration like this:
<!DOCTYPE web-appParsing with SAX 2
Example 19.1 showed how you can parse an XML document using the SAX 1 API, which is what is supported by the current version of JAXP (at this writing). The SAX 1 API is out of date, however. So Example 19.2 shows how you can accomplish a similar parsing task using the SAX 2 API and the open-source Xerces parser available from the Apache Software Foundation.
Example 19.2 is a listing of the program ListServlets2.java. Like the ListServlets1.java example, this program reads a specified web.xml file and looks for
<servlet>
tags, so it can print out the servlet name-to-servlet class mappings. This example goes a little further than the last, however, and also looks for<servlet-mapping>
tags, so it can also output the URL patterns that are mapped to named servlets. The example uses two hashtables to store the information as it accumulates it, then prints out all the information when parsing is complete.The SAX 2 API is functionally similar to the SAX 1 API, but a number of classes and interfaces have new names and some methods have new signatures. Many of the changes were required for the addition of XML namespace support in SAX 2. As you read through Example 19.2, pay attention to the API differences from Example 19.1.
Example 19.2: ListServlets2.java
package com.davidflanagan.examples.xml; import org.xml.sax.*; // The main SAX package import org.xml.sax.helpers.*; // SAX helper classes import java.io.*; // For reading the input file import java.util.*; // Hashtable, lists, and so on /** * Parse a web.xml file using the SAX2 API and the Xerces parser from the * Apache project. * * This class extends DefaultHandler so that instances can serve as SAX2 * event handlers, and can be notified by the parser of parsing events. * We simply override the methods that receive events we're interested in **/ public class ListServlets2 extends org.xml.sax.helpers.DefaultHandler {Compiling and Running the Example
The
ListServlets2
example uses the Xerces-J parser from the Apache XML Project. You can download this open-source parser by following the download links from http://xml.apache.org/. Once you have downloaded Xerces-J, unpack the distribution in a convenient location on your system. In that distribution, you should find a xerces.jar file. This file must be in your classpath to compile and run the ListServlets2.java example. Note that the xerces.jar file and the parsers.jar file from the JAXP distribution both contain versions of the SAX and DOM classes; you should avoid having both files in your classpath at the same time....