Written by JavaScript expert Stoyan Stefanov—Senior Yahoo! Technical and architect of YSlow 2.0, the web page performance optimization tool—JavaScript Patterns includes practical advice for implementing each pattern discussed, along with several hands-on examples. You'll also learn about anti-patterns: common programming approaches that cause more problems than they solve.
- Explore useful habits for writing high-quality JavaScript code, such as avoiding globals, using single var declarations, and more
- Learn why literal notation patterns are simpler alternatives to constructor functions
- Discover different ways to define a function in JavaScript
- Create objects that go beyond the basic patterns of using object literals and constructor functions
- Learn the options available for code reuse and inheritance in JavaScript
- Study sample JavaScript approaches to common design patterns such as Singleton, Factory, Decorator, and more
- Examine patterns that apply specifically to the client-side browser environment
Written by JavaScript expert Stoyan Stefanov—Senior Yahoo! Technical and architect of YSlow 2.0, the web page performance optimization tool—JavaScript Patterns includes practical advice for implementing each pattern discussed, along with several hands-on examples. You'll also learn about anti-patterns: common programming approaches that cause more problems than they solve.
- Explore useful habits for writing high-quality JavaScript code, such as avoiding globals, using single var declarations, and more
- Learn why literal notation patterns are simpler alternatives to constructor functions
- Discover different ways to define a function in JavaScript
- Create objects that go beyond the basic patterns of using object literals and constructor functions
- Learn the options available for code reuse and inheritance in JavaScript
- Study sample JavaScript approaches to common design patterns such as Singleton, Factory, Decorator, and more
- Examine patterns that apply specifically to the client-side browser environment
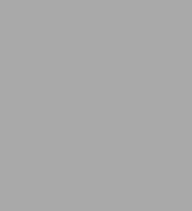
JavaScript Patterns: Build Better Applications with Coding and Design Patterns
232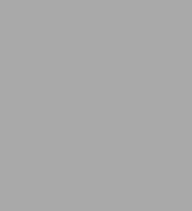
JavaScript Patterns: Build Better Applications with Coding and Design Patterns
232Product Details
ISBN-13: | 9780596806750 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 11/15/2010 |
Pages: | 232 |
Product dimensions: | 6.80(w) x 9.10(h) x 0.80(d) |