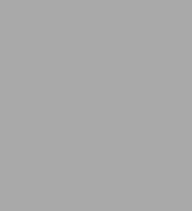
Object-Oriented Python: Master OOP by Building Games and GUIs
416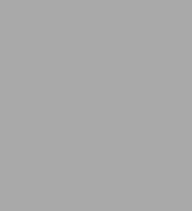
Object-Oriented Python: Master OOP by Building Games and GUIs
416Paperback
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
Object-Oriented Python is an intuitive and thorough guide to mastering object-oriented programming from the ground up. You’ll cover the basics of building classes and creating objects, and put theory into practice using the pygame package with clear examples that help visualize the object-oriented style. You’ll explore the key concepts of object-oriented programming — encapsulation, polymorphism, and inheritance — and learn not just how to code with objects, but the absolute best practices for doing so. Finally, you’ll bring it all together by building a complex video game, complete with full animations and sounds. The book covers two fully functional Python code packages that will speed up development of graphical user interface (GUI) programs in Python.
Product Details
ISBN-13: | 9781718502062 |
---|---|
Publisher: | No Starch Press |
Publication date: | 01/25/2022 |
Pages: | 416 |
Sales rank: | 656,510 |
Product dimensions: | 6.90(w) x 9.40(h) x 0.90(d) |
About the Author
Table of Contents
Acknowledgments xix
Introduction xxi
Who Is This Book For? xxii
Python Version(s) and Installation xxii
How Will I Explain OOP? xxiii
What's in the Book xxiv
Development Environments xxvi
Widgets and Example Games xxvi
Part I Introducing Object-Oriented Programming
1 Procedural Python Examples 3
Higher or Lower Card Game 4
Representing the Data 4
Implementation 4
Reusable Code 7
Bank Account Simulations 7
Analysis of Required Operations and Data 7
Implementation 1-Single Account Without Functions 8
Implementation 2-Single Account with Functions 10
Implementation 3-Two Accounts 12
Implementation 4-Multiple Accounts Using Lists 13
Implementation 5-List of Account Dictionaries 16
Common Problems with Procedural Implementation 18
Object-Oriented Solution -First Look at a Class 19
Summary 20
2 Modeling Physical Objects with Object-Oriented Programming 21
Building Software Models of Physical Objects 22
State and Behavior: Light Switch Example 22
Introduction to Classes and Objects 23
Classes, Objects, and instantiation 25
Writing a Class in Python 26
Scope and Instance Variables 27
Differences Between Functions and Methods 28
Creating an Object from a Class 28
Calling Methods of an Object 30
Creating Multiple Instances from the Same Class 31
Python Data Types Are Implemented as Classes 32
Definition of an Object 33
Building a Slightly More Complicated Class 33
Representing a More Complicated Physical Object as a Class 35
Passing Arguments to a Method 40
Multiple Instances 41
Initialization Parameters 43
Classes in Use 45
OOP as a Solution 45
Summary 46
3 Mental Models of Objects and the Meaning of "Self" 47
Revisiting the DimmerSwitch Class 48
High-Level Mental Model #1 49
A Deeper Mental Model #2 49
What Is the Meaning of "self"? 52
Summary 55
4 Managing Multiple Objects 57
Bank Account Class 58
Importing Class Code 60
Creating Some Test Code 61
Creating Multiple Accounts 62
Multiple Account Objects in a List 64
Multiple Objects with Unique Identifiers 66
Building an Interactive Menu 68
Creating an Object Manager Object 70
Building the Object Manager Object 72
Main Code That Creates an Object Manager Object 74
Better Error Handling with Exceptions 76
Try and except 76
The raise Statement and Custom Exceptions 77
Using Exceptions in Our Bank Program 78
Account Class with Exceptions 78
Optimized Bank Class 79
Main Code That Handles Exceptions 81
Calling the Same Method on a List of Objects 83
Interface vs. Implementation 84
Summary 85
Part II Graphical User Interfaces with Pygame
5 Introduction to Pygame 89
Installing Pygame 90
Window Details 91
The Window Coordinate System 91
Pixel Colors 94
Event-Driven Programs 95
Using Pygame 96
Bringing Up a Blank Window 97
Drawing an Image 100
Detecting a Mouse Click 102
Handling the Keyboard 105
Creating a Location-Based Animation 109
Using Pygame rects 111
Playing Sounds 114
Playing Sound Effects 114
Playing Background Music 115
Drawing Shapes 116
Reference for Primitive Shapes 118
Summary 120
6 Object-Oriented Pygame 121
Building the Screensaver Ball with OOP Pygame 121
Creating a Ball Class 122
Using the Ball Class 124
Creating Many Ball Objects 125
Creating Many, Many Ball Objects 126
Building a Reusable Object-Oriented Button 127
Building a Burton Class 128
Main Code Using a SimpleButton 130
Creating a Program with Multiple Buttons 131
Building a Reusable Object-Oriented Text Display 133
Steps to Display Text 133
Creating a SimpleText Class 133
Demo Ball with SimpleText and SimpleButton 135
Interface vs. Implementation 137
Callbacks 137
Creating a Callback 138
Using a Callback with SimpleButton 139
Summary 141
7 Pygame Gui Widgets 143
Passing Arguments into a Function or Method 144
Positional and Keyword Parameters 145
Additional Notes on Keyword Parameters 146
Using None as a Default Value 146
Choosing Keywords and Default Values 148
Default Values in GUI Widgets 148
The pygwidgets Package 148
Setting Up 149
Overall Design Approach 150
Adding an Image 151
Adding Buttons, Checkboxes, and Radio Buttons 152
Text Output and Input 154
Other pygwidgets Classes 157
Pygwidgets Example Program 157
The Importance of a Consistent API 158
Summary 158
Part III Encapsulation, Polymorphism, and Inheritance
8 Encapsulation 163
Encapsulation with Functions 164
Encapsulation with Objects 164
Objects Own Their Data 165
Interpretations of Encapsulation 165
Direct Access and Why You Should Avoid It 166
Strict Interpretation with Getters and Setters 170
Safe Direct Access 172
Making Instance Variables More Private 172
Implicitly Private 172
More Explicitly Private 173
Decorators and @property 174
Encapsulation in pygwidgets Classes 177
A Story from the Real World 178
Abstraction 179
Summary 182
9 Polymorphism 183
Sending Messages to Real-World Objects 184
A Classic Example of Polymorphism in Programming 184
Example Using Pygame Shapes 185
The Square Shape Class 186
The Circle and Triangle Shape Classes 187
The Main Program Creating Shapes 190
Extending a Pattern 192
Pygwidgets Exhibits Polymorphism 192
Polymorphism for Operators 193
Magic Methods 194
Comparison Operator Magic Methods 195
A Rectangle Class with Magic Methods 196
Main Program Using Magic Methods 198
Math Operator Magic Methods 200
Vector Example 201
Creating a String Representation of Values in an Object 203
A Fraction Class with Magic Methods 205
Summary 208
10 Inheritance 211
Inheritance in Object-Oriented Programming 212
Implementing Inheritance 213
Employee and Manager Example 214
Base Class: Employee 214
Subclass: Manager 215
Test Code 217
The Client's View of a Subclass 218
Real-World Examples of Inheritance 219
InputNumber 219
DisplayMoney 222
Example Usage 224
Multiple Classes Inheriting from the Same Base Class 227
Abstract Classes and Methods 231
How pygwidgets Uses Inheritance 234
Class Hierarchy 236
The Difficulty of Programming with Inheritance 238
Summary 239
11 Managing Memory Used by Objects 241
Object Lifetime 242
Reference Count 242
Garbage Collection 248
Class Variables 248
Class Variable Constants 249
Class Variables for Counting 250
Putting It All Together: Balloon Sample Program 251
Module of Constants 253
Main Program Code 254
Balloon Manager 256
Balloon Class and Objects 258
Managing Memory: Slots 261
Summary 263
Part IV Using Oop in Game Development
12 Card Games 267
The Cord Class 268
The Deck Class 270
The Higher or Lower Game 272
Main Program 272
Game Object 274
Testing with name_ 276
Other Card Games 278
Blackjack Deck 278
Games with Unusual Card Decks 279
Summary 279
13 Timers 281
Timer Demonstration Program 282
Three Approaches for implementing Timers 283
Counting Frames 283
Timer Event 284
Building a Timer by Calculating Elapsed Time 285
Installing pyghelpers 287
The Timer Class 287
Displaying Time 290
CountUpTimer 291
CountDownTimer 293
Summary 294
14 Animation 295
Building Animation Classes 296
SimpleAnimation Class 296
SimpleSpriteSheetAnimation Class 300
Merging Two Classes 304
Animation Classes in pygwidgets 304
Animation Class 305
SpriteSheetAnimation Class 306
Common Base Class: PygAnimation 307
Example Animation Program 308
Summary 310
15 Scenes 311
The State Machine Approach 312
A pygame Example with a State Machine 314
A Scene Manager for Managing Many Scenes 319
A Demo Program Using a Scene Manager 320
The Main Program 322
Building the Scenes 323
A Typical Scene 326
Rock, Paper, Scissors Using Scenes 328
Communication Between Scenes 332
Requesting Information from a Target Scene 333
Sending Information to a Target Scene 333
Sending Information to All Scenes 334
Testing Communications Among Scenes 334
Implementation of the Scene Manager 334
Run() Method 336
Main Methods 337
Communication Between Scenes 338
Summary 340
16 Full Game: Dodger 341
Modal Dialogs 342
Yes/No and Alert Dialogs 342
Answer Dialogs 345
Building a Full Game: Dodger 347
Game Overview 347
Implementation 348
Extensions to the Game 366
Summary 366
17 Design Patterns and Wrap-Up 367
Model View Controller 367
File Display Example 368
Statistical Display Example 368
Advantages of the MVC Pattern 373
Wrap-Up 374
Index 377