Summary
With Redux in Action, you'll discover how to integrate Redux into your React application and development environment. With the insights you glean from the experience of authors Marc Garreau and Will Faurot, you'll be more than confident in your ability to solve your state management woes with Redux and focus on developing the apps you need!
Foreword by Mark Erikson, Redux co-maintainer.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
With Redux, you manage the state of a web application in a single, simple object, practically eliminating most state-related bugs. Centralizing state with Redux makes it possible to quickly start saved user sessions, maintain a reliable state history, and smoothly transfer state between UIs. Plus, the Redux state container is fully programmable and integrates cleanly with React and other popular frameworks.
About the Book
Redux in Action is an accessible guide to effectively managing state in web applications. Built around common use cases, this practical book starts with a simple task-management application built in React. You'll use the app to learn the Redux workflow, handle asynchronous actions, and get your hands on the Redux developer tools. With each step, you'll discover more about Redux and the benefits of centralized state management. The book progresses to more-complex examples, including writing middleware for analytics, time travel debugging, and an overview of how Redux works with other frameworks such as Angular and Electron.
What's Inside
About the Reader
For web developers comfortable with JavaScript and React.
About the Author
Marc Garreau has architected and executed half a dozen unique client-side applications using Redux. Will Faurot is a mentor for Redux developers of all skill levels.
Table of Contents
1126999341
With Redux in Action, you'll discover how to integrate Redux into your React application and development environment. With the insights you glean from the experience of authors Marc Garreau and Will Faurot, you'll be more than confident in your ability to solve your state management woes with Redux and focus on developing the apps you need!
Foreword by Mark Erikson, Redux co-maintainer.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
With Redux, you manage the state of a web application in a single, simple object, practically eliminating most state-related bugs. Centralizing state with Redux makes it possible to quickly start saved user sessions, maintain a reliable state history, and smoothly transfer state between UIs. Plus, the Redux state container is fully programmable and integrates cleanly with React and other popular frameworks.
About the Book
Redux in Action is an accessible guide to effectively managing state in web applications. Built around common use cases, this practical book starts with a simple task-management application built in React. You'll use the app to learn the Redux workflow, handle asynchronous actions, and get your hands on the Redux developer tools. With each step, you'll discover more about Redux and the benefits of centralized state management. The book progresses to more-complex examples, including writing middleware for analytics, time travel debugging, and an overview of how Redux works with other frameworks such as Angular and Electron.
What's Inside
- Using Redux in an existing React application
- Handling side effects with the redux-saga library
- Consuming APIs with asynchronous actions
- Unit testing a React and Redux application
About the Reader
For web developers comfortable with JavaScript and React.
About the Author
Marc Garreau has architected and executed half a dozen unique client-side applications using Redux. Will Faurot is a mentor for Redux developers of all skill levels.
Table of Contents
- Introducing Redux
- Your first Redux application
- Debugging Redux applications
- Consuming an API
- Middleware
- Handling complex side effects
- Preparing data for components
- Structuring a Redux store
- Testing Redux applications
- Performance
- Structuring Redux code
- Redux beyond React
Redux in Action
Summary
With Redux in Action, you'll discover how to integrate Redux into your React application and development environment. With the insights you glean from the experience of authors Marc Garreau and Will Faurot, you'll be more than confident in your ability to solve your state management woes with Redux and focus on developing the apps you need!
Foreword by Mark Erikson, Redux co-maintainer.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
With Redux, you manage the state of a web application in a single, simple object, practically eliminating most state-related bugs. Centralizing state with Redux makes it possible to quickly start saved user sessions, maintain a reliable state history, and smoothly transfer state between UIs. Plus, the Redux state container is fully programmable and integrates cleanly with React and other popular frameworks.
About the Book
Redux in Action is an accessible guide to effectively managing state in web applications. Built around common use cases, this practical book starts with a simple task-management application built in React. You'll use the app to learn the Redux workflow, handle asynchronous actions, and get your hands on the Redux developer tools. With each step, you'll discover more about Redux and the benefits of centralized state management. The book progresses to more-complex examples, including writing middleware for analytics, time travel debugging, and an overview of how Redux works with other frameworks such as Angular and Electron.
What's Inside
About the Reader
For web developers comfortable with JavaScript and React.
About the Author
Marc Garreau has architected and executed half a dozen unique client-side applications using Redux. Will Faurot is a mentor for Redux developers of all skill levels.
Table of Contents
With Redux in Action, you'll discover how to integrate Redux into your React application and development environment. With the insights you glean from the experience of authors Marc Garreau and Will Faurot, you'll be more than confident in your ability to solve your state management woes with Redux and focus on developing the apps you need!
Foreword by Mark Erikson, Redux co-maintainer.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
With Redux, you manage the state of a web application in a single, simple object, practically eliminating most state-related bugs. Centralizing state with Redux makes it possible to quickly start saved user sessions, maintain a reliable state history, and smoothly transfer state between UIs. Plus, the Redux state container is fully programmable and integrates cleanly with React and other popular frameworks.
About the Book
Redux in Action is an accessible guide to effectively managing state in web applications. Built around common use cases, this practical book starts with a simple task-management application built in React. You'll use the app to learn the Redux workflow, handle asynchronous actions, and get your hands on the Redux developer tools. With each step, you'll discover more about Redux and the benefits of centralized state management. The book progresses to more-complex examples, including writing middleware for analytics, time travel debugging, and an overview of how Redux works with other frameworks such as Angular and Electron.
What's Inside
- Using Redux in an existing React application
- Handling side effects with the redux-saga library
- Consuming APIs with asynchronous actions
- Unit testing a React and Redux application
About the Reader
For web developers comfortable with JavaScript and React.
About the Author
Marc Garreau has architected and executed half a dozen unique client-side applications using Redux. Will Faurot is a mentor for Redux developers of all skill levels.
Table of Contents
- Introducing Redux
- Your first Redux application
- Debugging Redux applications
- Consuming an API
- Middleware
- Handling complex side effects
- Preparing data for components
- Structuring a Redux store
- Testing Redux applications
- Performance
- Structuring Redux code
- Redux beyond React
34.99
In Stock
5
1
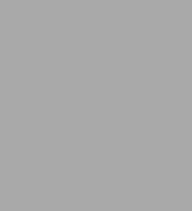
Redux in Action
312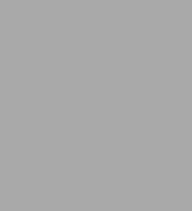
Redux in Action
312Related collections and offers
34.99
In Stock
From the B&N Reads Blog