The Ruby Programming Language: Everything You Need to Know
The book also includes a long and thorough introduction to the rich API of the Ruby platform, demonstrating -- with heavily-commented example code -- Ruby's facilities for text processing, numeric manipulation, collections, input/output, networking, and concurrency. An entire chapter is devoted to Ruby's metaprogramming capabilities.
The Ruby Programming Language documents the Ruby language definitively but without the formality of a language specification. It is written for experienced programmers who are new to Ruby, and for current Ruby programmers who want to challenge their understanding and increase their mastery of the language.
1100157511
The Ruby Programming Language is the authoritative guide to Ruby and provides comprehensive coverage of versions 1.8 and 1.9 of the language. It was written (and illustrated!) by an all-star team:
- David Flanagan, bestselling author of programming language "bibles" (including JavaScript: The Definitive Guide and Java in a Nutshell) and committer to the Ruby Subversion repository.
- Yukihiro "Matz" Matsumoto, creator, designer and lead developer of Ruby and author of Ruby in a Nutshell, which has been expanded and revised to become this book.
- why the lucky stiff, artist and Ruby programmer extraordinaire.
The book also includes a long and thorough introduction to the rich API of the Ruby platform, demonstrating -- with heavily-commented example code -- Ruby's facilities for text processing, numeric manipulation, collections, input/output, networking, and concurrency. An entire chapter is devoted to Ruby's metaprogramming capabilities.
The Ruby Programming Language documents the Ruby language definitively but without the formality of a language specification. It is written for experienced programmers who are new to Ruby, and for current Ruby programmers who want to challenge their understanding and increase their mastery of the language.
The Ruby Programming Language: Everything You Need to Know
The book also includes a long and thorough introduction to the rich API of the Ruby platform, demonstrating -- with heavily-commented example code -- Ruby's facilities for text processing, numeric manipulation, collections, input/output, networking, and concurrency. An entire chapter is devoted to Ruby's metaprogramming capabilities.
The Ruby Programming Language documents the Ruby language definitively but without the formality of a language specification. It is written for experienced programmers who are new to Ruby, and for current Ruby programmers who want to challenge their understanding and increase their mastery of the language.
The Ruby Programming Language is the authoritative guide to Ruby and provides comprehensive coverage of versions 1.8 and 1.9 of the language. It was written (and illustrated!) by an all-star team:
- David Flanagan, bestselling author of programming language "bibles" (including JavaScript: The Definitive Guide and Java in a Nutshell) and committer to the Ruby Subversion repository.
- Yukihiro "Matz" Matsumoto, creator, designer and lead developer of Ruby and author of Ruby in a Nutshell, which has been expanded and revised to become this book.
- why the lucky stiff, artist and Ruby programmer extraordinaire.
The book also includes a long and thorough introduction to the rich API of the Ruby platform, demonstrating -- with heavily-commented example code -- Ruby's facilities for text processing, numeric manipulation, collections, input/output, networking, and concurrency. An entire chapter is devoted to Ruby's metaprogramming capabilities.
The Ruby Programming Language documents the Ruby language definitively but without the formality of a language specification. It is written for experienced programmers who are new to Ruby, and for current Ruby programmers who want to challenge their understanding and increase their mastery of the language.
42.99
In Stock
5
1
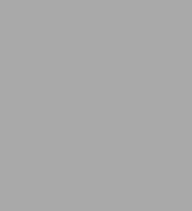
The Ruby Programming Language: Everything You Need to Know
448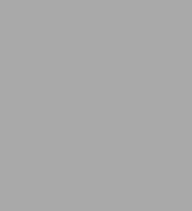
The Ruby Programming Language: Everything You Need to Know
448
42.99
In Stock
Product Details
ISBN-13: | 9780596554651 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 01/25/2008 |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 448 |
File size: | 3 MB |
About the Author
From the B&N Reads Blog