Testing Vue.js Applications
Summary
Testing Vue.js Applications is a comprehensive guide to testing Vue components, methods, events, and output. Author Edd Yerburgh, creator of the Vue testing utility, explains the best testing practices in Vue along with an evergreen methodology that applies to any web dev process.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Web developers who use the Vue framework love its reliability, speed, small footprint, and versatility. Vue's component-based approach and use of DOM methods require you to adapt your app-testing practices. Learning Vue-specific testing tools and strategies will ensure your apps run like they should.
About the Book
With Testing Vue.js Applications, you'll discover effective testing methods for Vue applications. You'll enjoy author Edd Yerburgh's engaging style and fun real-world examples as you learn to use the Jest framework to run tests for a Hacker News application built with Vue, Vuex, and Vue Router. This comprehensive guide teaches the best testing practices in Vue along with an evergreen methodology that applies to any web dev process.
What's inside
About the Reader
Written for Vue developers at any level.
About the Author
Edd Yerburgh is a JavaScript developer and Vue core team member. He's the main author of the Vue Test Utils library and is passionate about open source tooling for testing component-based applications.
Table of Contents
1127875588
Testing Vue.js Applications is a comprehensive guide to testing Vue components, methods, events, and output. Author Edd Yerburgh, creator of the Vue testing utility, explains the best testing practices in Vue along with an evergreen methodology that applies to any web dev process.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Web developers who use the Vue framework love its reliability, speed, small footprint, and versatility. Vue's component-based approach and use of DOM methods require you to adapt your app-testing practices. Learning Vue-specific testing tools and strategies will ensure your apps run like they should.
About the Book
With Testing Vue.js Applications, you'll discover effective testing methods for Vue applications. You'll enjoy author Edd Yerburgh's engaging style and fun real-world examples as you learn to use the Jest framework to run tests for a Hacker News application built with Vue, Vuex, and Vue Router. This comprehensive guide teaches the best testing practices in Vue along with an evergreen methodology that applies to any web dev process.
What's inside
- Unit tests, snapshot tests, and end-to-end tests
- Writing unit tests for Vue components
- Writing tests for Vue mixins, Vuex, and Vue Router
- Advanced testing techniques, like mocking
About the Reader
Written for Vue developers at any level.
About the Author
Edd Yerburgh is a JavaScript developer and Vue core team member. He's the main author of the Vue Test Utils library and is passionate about open source tooling for testing component-based applications.
Table of Contents
- Introduction to testing Vue applications
- Creating your first test
- Testing rendered component output
- Testing component methods
- Testing events
- Understanding Vuex
- Testing Vuex
- Organizing tests with factory functions
- Understanding Vue Router
- Testing Vue Router
- Testing mixins and filters
- Writing snapshot tests
- Testing server-side rendering
- Writing end-to-end tests
- APPENDIXES
- A - Setting up your environment
- B - Running the production build
- C - Exercise answers
Testing Vue.js Applications
Summary
Testing Vue.js Applications is a comprehensive guide to testing Vue components, methods, events, and output. Author Edd Yerburgh, creator of the Vue testing utility, explains the best testing practices in Vue along with an evergreen methodology that applies to any web dev process.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Web developers who use the Vue framework love its reliability, speed, small footprint, and versatility. Vue's component-based approach and use of DOM methods require you to adapt your app-testing practices. Learning Vue-specific testing tools and strategies will ensure your apps run like they should.
About the Book
With Testing Vue.js Applications, you'll discover effective testing methods for Vue applications. You'll enjoy author Edd Yerburgh's engaging style and fun real-world examples as you learn to use the Jest framework to run tests for a Hacker News application built with Vue, Vuex, and Vue Router. This comprehensive guide teaches the best testing practices in Vue along with an evergreen methodology that applies to any web dev process.
What's inside
About the Reader
Written for Vue developers at any level.
About the Author
Edd Yerburgh is a JavaScript developer and Vue core team member. He's the main author of the Vue Test Utils library and is passionate about open source tooling for testing component-based applications.
Table of Contents
Testing Vue.js Applications is a comprehensive guide to testing Vue components, methods, events, and output. Author Edd Yerburgh, creator of the Vue testing utility, explains the best testing practices in Vue along with an evergreen methodology that applies to any web dev process.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Web developers who use the Vue framework love its reliability, speed, small footprint, and versatility. Vue's component-based approach and use of DOM methods require you to adapt your app-testing practices. Learning Vue-specific testing tools and strategies will ensure your apps run like they should.
About the Book
With Testing Vue.js Applications, you'll discover effective testing methods for Vue applications. You'll enjoy author Edd Yerburgh's engaging style and fun real-world examples as you learn to use the Jest framework to run tests for a Hacker News application built with Vue, Vuex, and Vue Router. This comprehensive guide teaches the best testing practices in Vue along with an evergreen methodology that applies to any web dev process.
What's inside
- Unit tests, snapshot tests, and end-to-end tests
- Writing unit tests for Vue components
- Writing tests for Vue mixins, Vuex, and Vue Router
- Advanced testing techniques, like mocking
About the Reader
Written for Vue developers at any level.
About the Author
Edd Yerburgh is a JavaScript developer and Vue core team member. He's the main author of the Vue Test Utils library and is passionate about open source tooling for testing component-based applications.
Table of Contents
- Introduction to testing Vue applications
- Creating your first test
- Testing rendered component output
- Testing component methods
- Testing events
- Understanding Vuex
- Testing Vuex
- Organizing tests with factory functions
- Understanding Vue Router
- Testing Vue Router
- Testing mixins and filters
- Writing snapshot tests
- Testing server-side rendering
- Writing end-to-end tests
- APPENDIXES
- A - Setting up your environment
- B - Running the production build
- C - Exercise answers
34.99
In Stock
5
1
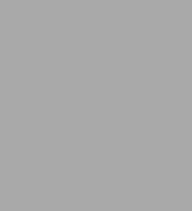
Testing Vue.js Applications
272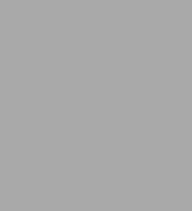
Testing Vue.js Applications
272
34.99
In Stock
From the B&N Reads Blog