Vue.js in Action
Summary
Web pages are rich with data and graphics, and it's challenging to maintain a smooth and quick user experience. Vue.js in Action teaches you how to build a fast, flowing web UI with the Vue.js framework. As you move through the book, you'll put your skills to practice by building a complete web store application with product listings, a checkout process, and an administrative interface.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Vue.js is a lightweight frontend framework, offering easy two-way data binding, a reactive UI, and a common-sense project structure. It uses UI patterns and modern HTML to deliver impossibly fast page loads and silky smooth transitions—all from a tiny code footprint. It’s a delight to develop in Vue using ordinary JavaScript and its integrated Vuex state management tool.
About the book
Vue.js in Action is your guide to building modern web apps. You’ll start by exploring the reactive UI model while you get comfortable with Vue’s unique features. Then, you’ll go deeper as you build a shopping cart with an admin interface and the ability to manage stock! Finally, you’ll extend your app, adding transitions, tests, and other key features until it’s production ready.
What's inside
Clearly annotated code and illustrations
Modeling data and consuming APIs
Easy state management with Vuex
Creating custom directives
About the reader
Written for web developers with some experience in JavaScript, HTML, and CSS.
About the author
Erik Hanchett and Benjamin Listwon are experienced web engineers and fearless explorers of new ideas.
1127522923
Web pages are rich with data and graphics, and it's challenging to maintain a smooth and quick user experience. Vue.js in Action teaches you how to build a fast, flowing web UI with the Vue.js framework. As you move through the book, you'll put your skills to practice by building a complete web store application with product listings, a checkout process, and an administrative interface.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Vue.js is a lightweight frontend framework, offering easy two-way data binding, a reactive UI, and a common-sense project structure. It uses UI patterns and modern HTML to deliver impossibly fast page loads and silky smooth transitions—all from a tiny code footprint. It’s a delight to develop in Vue using ordinary JavaScript and its integrated Vuex state management tool.
About the book
Vue.js in Action is your guide to building modern web apps. You’ll start by exploring the reactive UI model while you get comfortable with Vue’s unique features. Then, you’ll go deeper as you build a shopping cart with an admin interface and the ability to manage stock! Finally, you’ll extend your app, adding transitions, tests, and other key features until it’s production ready.
What's inside
Clearly annotated code and illustrations
Modeling data and consuming APIs
Easy state management with Vuex
Creating custom directives
About the reader
Written for web developers with some experience in JavaScript, HTML, and CSS.
About the author
Erik Hanchett and Benjamin Listwon are experienced web engineers and fearless explorers of new ideas.
Vue.js in Action
Summary
Web pages are rich with data and graphics, and it's challenging to maintain a smooth and quick user experience. Vue.js in Action teaches you how to build a fast, flowing web UI with the Vue.js framework. As you move through the book, you'll put your skills to practice by building a complete web store application with product listings, a checkout process, and an administrative interface.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Vue.js is a lightweight frontend framework, offering easy two-way data binding, a reactive UI, and a common-sense project structure. It uses UI patterns and modern HTML to deliver impossibly fast page loads and silky smooth transitions—all from a tiny code footprint. It’s a delight to develop in Vue using ordinary JavaScript and its integrated Vuex state management tool.
About the book
Vue.js in Action is your guide to building modern web apps. You’ll start by exploring the reactive UI model while you get comfortable with Vue’s unique features. Then, you’ll go deeper as you build a shopping cart with an admin interface and the ability to manage stock! Finally, you’ll extend your app, adding transitions, tests, and other key features until it’s production ready.
What's inside
Clearly annotated code and illustrations
Modeling data and consuming APIs
Easy state management with Vuex
Creating custom directives
About the reader
Written for web developers with some experience in JavaScript, HTML, and CSS.
About the author
Erik Hanchett and Benjamin Listwon are experienced web engineers and fearless explorers of new ideas.
Web pages are rich with data and graphics, and it's challenging to maintain a smooth and quick user experience. Vue.js in Action teaches you how to build a fast, flowing web UI with the Vue.js framework. As you move through the book, you'll put your skills to practice by building a complete web store application with product listings, a checkout process, and an administrative interface.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Vue.js is a lightweight frontend framework, offering easy two-way data binding, a reactive UI, and a common-sense project structure. It uses UI patterns and modern HTML to deliver impossibly fast page loads and silky smooth transitions—all from a tiny code footprint. It’s a delight to develop in Vue using ordinary JavaScript and its integrated Vuex state management tool.
About the book
Vue.js in Action is your guide to building modern web apps. You’ll start by exploring the reactive UI model while you get comfortable with Vue’s unique features. Then, you’ll go deeper as you build a shopping cart with an admin interface and the ability to manage stock! Finally, you’ll extend your app, adding transitions, tests, and other key features until it’s production ready.
What's inside
Clearly annotated code and illustrations
Modeling data and consuming APIs
Easy state management with Vuex
Creating custom directives
About the reader
Written for web developers with some experience in JavaScript, HTML, and CSS.
About the author
Erik Hanchett and Benjamin Listwon are experienced web engineers and fearless explorers of new ideas.
44.99
Out Of Stock
5
1
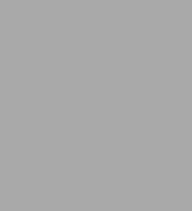
Vue.js in Action
375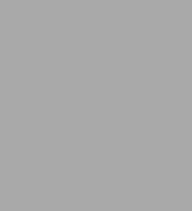
Vue.js in Action
375Paperback(1st Edition)
$44.99
Related collections and offers
44.99
Out Of Stock
From the B&N Reads Blog