AOP in .NET: Practical Aspect-Oriented Programming
Summary
AOP in .NET introduces aspect-oriented programming to .NET developers and provides practical guidance on how to get the most benefit from this technique in your everyday coding. The book's many examples concentrate on modularizing non-functional requirements that often sprawl throughout object-oriented projects. Even if you've never tried AOP before, you'll appreciate the straightforward introduction using familiar C#-based examples. AOP tools for .NET have now reached the level of practical maturity Java developers have relied on for many years, and you'll explore the leading options, PostSharp, and Castle DynamicProxy.
About the Technology
Core concerns that cut across all parts of your application, such as logging or authorization, are difficult to maintain independently. In aspect-oriented programming (AOP) you isolate these cross-cutting concerns into their own classes, disentangling them from business logic. Mature AOP tools like PostSharp and Castle DynamicProxy now offer .NET developers the level of support Java coders have relied on for years.
About this Book
AOP in .NET introduces aspect-oriented programming and provides guidance on how to get the most practical benefit from this technique. The book's many examples concentrate on modularizing non-functional requirements that often sprawl throughout object-oriented projects. You'll appreciate its straightforward introduction using familiar C#-based examples.
This book requires no prior experience with AOP. Readers should know C# or another OO language.
What's Inside
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Author
Matthew D. Groves is a developer with over ten years of professional experience working with C#, ASP.NET, JavaScript, and PHP.
Table of Contents
1114672154
AOP in .NET introduces aspect-oriented programming to .NET developers and provides practical guidance on how to get the most benefit from this technique in your everyday coding. The book's many examples concentrate on modularizing non-functional requirements that often sprawl throughout object-oriented projects. Even if you've never tried AOP before, you'll appreciate the straightforward introduction using familiar C#-based examples. AOP tools for .NET have now reached the level of practical maturity Java developers have relied on for many years, and you'll explore the leading options, PostSharp, and Castle DynamicProxy.
About the Technology
Core concerns that cut across all parts of your application, such as logging or authorization, are difficult to maintain independently. In aspect-oriented programming (AOP) you isolate these cross-cutting concerns into their own classes, disentangling them from business logic. Mature AOP tools like PostSharp and Castle DynamicProxy now offer .NET developers the level of support Java coders have relied on for years.
About this Book
AOP in .NET introduces aspect-oriented programming and provides guidance on how to get the most practical benefit from this technique. The book's many examples concentrate on modularizing non-functional requirements that often sprawl throughout object-oriented projects. You'll appreciate its straightforward introduction using familiar C#-based examples.
This book requires no prior experience with AOP. Readers should know C# or another OO language.
What's Inside
- Clear and simple introduction to AOP
- Maximum benefit with minimal theory
- PostSharp and Castle DynamicProxy
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Author
Matthew D. Groves is a developer with over ten years of professional experience working with C#, ASP.NET, JavaScript, and PHP.
Table of Contents
- Introducing AOP
- Acme Car Rental Call this instead: intercepting methods
- Before and after: boundary aspects
- Get this instead: intercepting locations
- Unit testing aspects AOP implementation types
- Using AOP as an architectural tool
- Aspect composition: example and execution
AOP in .NET: Practical Aspect-Oriented Programming
Summary
AOP in .NET introduces aspect-oriented programming to .NET developers and provides practical guidance on how to get the most benefit from this technique in your everyday coding. The book's many examples concentrate on modularizing non-functional requirements that often sprawl throughout object-oriented projects. Even if you've never tried AOP before, you'll appreciate the straightforward introduction using familiar C#-based examples. AOP tools for .NET have now reached the level of practical maturity Java developers have relied on for many years, and you'll explore the leading options, PostSharp, and Castle DynamicProxy.
About the Technology
Core concerns that cut across all parts of your application, such as logging or authorization, are difficult to maintain independently. In aspect-oriented programming (AOP) you isolate these cross-cutting concerns into their own classes, disentangling them from business logic. Mature AOP tools like PostSharp and Castle DynamicProxy now offer .NET developers the level of support Java coders have relied on for years.
About this Book
AOP in .NET introduces aspect-oriented programming and provides guidance on how to get the most practical benefit from this technique. The book's many examples concentrate on modularizing non-functional requirements that often sprawl throughout object-oriented projects. You'll appreciate its straightforward introduction using familiar C#-based examples.
This book requires no prior experience with AOP. Readers should know C# or another OO language.
What's Inside
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Author
Matthew D. Groves is a developer with over ten years of professional experience working with C#, ASP.NET, JavaScript, and PHP.
Table of Contents
AOP in .NET introduces aspect-oriented programming to .NET developers and provides practical guidance on how to get the most benefit from this technique in your everyday coding. The book's many examples concentrate on modularizing non-functional requirements that often sprawl throughout object-oriented projects. Even if you've never tried AOP before, you'll appreciate the straightforward introduction using familiar C#-based examples. AOP tools for .NET have now reached the level of practical maturity Java developers have relied on for many years, and you'll explore the leading options, PostSharp, and Castle DynamicProxy.
About the Technology
Core concerns that cut across all parts of your application, such as logging or authorization, are difficult to maintain independently. In aspect-oriented programming (AOP) you isolate these cross-cutting concerns into their own classes, disentangling them from business logic. Mature AOP tools like PostSharp and Castle DynamicProxy now offer .NET developers the level of support Java coders have relied on for years.
About this Book
AOP in .NET introduces aspect-oriented programming and provides guidance on how to get the most practical benefit from this technique. The book's many examples concentrate on modularizing non-functional requirements that often sprawl throughout object-oriented projects. You'll appreciate its straightforward introduction using familiar C#-based examples.
This book requires no prior experience with AOP. Readers should know C# or another OO language.
What's Inside
- Clear and simple introduction to AOP
- Maximum benefit with minimal theory
- PostSharp and Castle DynamicProxy
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Author
Matthew D. Groves is a developer with over ten years of professional experience working with C#, ASP.NET, JavaScript, and PHP.
Table of Contents
- Introducing AOP
- Acme Car Rental Call this instead: intercepting methods
- Before and after: boundary aspects
- Get this instead: intercepting locations
- Unit testing aspects AOP implementation types
- Using AOP as an architectural tool
- Aspect composition: example and execution
49.99
In Stock
5
1
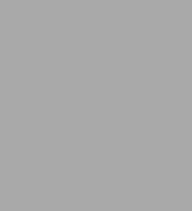
AOP in .NET: Practical Aspect-Oriented Programming
296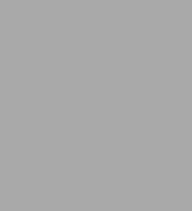
AOP in .NET: Practical Aspect-Oriented Programming
296Paperback(1st Edition)
$49.99
49.99
In Stock
From the B&N Reads Blog