C++ from the Ground Up, Third Edition / Edition 3 available in Paperback
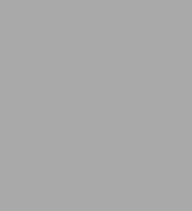
C++ from the Ground Up, Third Edition / Edition 3
- ISBN-10:
- 0072228970
- ISBN-13:
- 9780072228977
- Pub. Date:
- 03/19/2003
- Publisher:
- Osborne
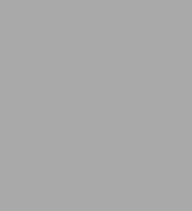
C++ from the Ground Up, Third Edition / Edition 3
Paperback
Buy New
$58.00Buy Used
$39.56-
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
-
Overview
Publisher's Note: Products purchased from Third Party sellers are not guaranteed by the publisher for quality, authenticity, or access to any online entitlements included with the product.
This excellent primer provides a plethora of C++ programming information for beginning to intermediate users on topics like classes, objects, expressions, arrays, pointers. Plus, it also covers advanced topics like inheritance, namespaces, STL, custom string class, and the Standard C++ Class Library. For users of all levels, this guide teaches with examples of source code and a thorough display of the results.
Product Details
ISBN-13: | 9780072228977 |
---|---|
Publisher: | Osborne |
Publication date: | 03/19/2003 |
Series: | From the Ground Up |
Edition description: | Subsequent |
Pages: | 624 |
Product dimensions: | 9.04(w) x 7.34(h) x 1.30(d) |
About the Author
Read an Excerpt
Chapter 1: The Story of C++
...What Is Object-Oriented Programming?
Since object-oriented programming was fundamental to the development of C++, it is important to define precisely what object-oriented programming is. Object-oriented programming has taken the best ideas of structured programming and has combined them with several powerful concepts that allow you to organize your programs more effectively. In general, when programming in an object-oriented fashion, you decompose a problem into its constituent parts. Each component becomes a self-contained object that contains its own instructions and data related to that object. Through this process, complexity is reduced and you can manage larger programs.All object-oriented programming languages have three things in common: encapsulation, polymorphism, and inheritance. Although we will examine these concepts in detail later in this book, let's take a brief look at them now.
Encapsulation
As you probably know, all programs are composed of two fundamental elements: program statements (code) and data. Code is that part of a program that performs actions, and data is the information affected by those actions. Encapsulation is a programming mechanism that binds together code and the data it manipulates, and that keeps both safe from outside interference and misuse. In an object-oriented language, code and data may be bound together in such a way that a self-contained black box is created. Within the box are all necessary data and code. When code and data are linked together in this fashion, an object is created. In other words, an object is the device that supportsencapsulation.Within an object, the code, data, or both may be private to that object or public. Private code or data is known to, and accessible only by, another part of the object. That is, private code or data may not be accessed by a piece of the program that exists outside the object. When code or data is public, other parts of your program may access it even though it is defined within an object. Typically, the public parts of an object are used to provide a controlled interface to the private elements of the object.
Polymorphism
Polymorphism (from the Greek, meaning "many forms") is the quality that allows one interface to be used for a general class of actions. The specific action is determined by the exact nature of the situation. A simple example of polymorphism is found in the steering wheel of an automobile. The steering wheel (i.e., the interface) is the same no matter what type of actual steering mechanism is used. That is, the steering wheel works the same whether your car has manual steering, power steering, or rack-and-pinion steering. Therefore, once you know how to operate the steering wheel, you can drive any type of car. The same principle can also apply to programming. For example, consider a stack (which is a first-in, last-out list). You might have a program that requires three different types of stacks. One stack is used for integer values, one for floating-point values, and one for characters. In this case, the algorithm that implements each stack is the same, even though the data being stored differs. In a non-object-oriented language, you would be required to create three different sets of stack routines, calling each set by a different name, with each set having its own interface. However, because of polymorphism, in C++ you can create one general set of stack routines (one interface) that works for all three specific situations. This way, once you know how to use one stack, you can use them all.More generally, the concept of polymorphism is often expressed by the phrase "one interface, multiple methods." This means that it is possible to design a generic interface to a group of related activities. Polymorphism helps reduce complexity by allowing the same interface to be used to specify a general class of action. It is the compiler's job to select the speciflc action (i.e., method) as it applies to each situation. You, the programmer, don't need to do this selection manually. You need only remember and utilize the general interface.
The first object-oriented programming languages were interpreters, so polymorphism was, of course, supported at run time. However, C++ is a compiled language. Therefore, in C++, both run-time and compile-time polymorphism are supported.
Inheritance
Inheritance is the process by which one object can acquire the properties of another object. The reason this is important is that it supports the concept of hierarchical classification. If you think about it, most knowledge is made manageable by hierarchical (i.e., top-down) classifications. For example, a Red Delicious apple is part of the classification apple, which in turn is part of the fruit class, which is under the larger class food. That is, the food class possesses certain qualities (edible, nutritious, etc.) that also apply, logically, to its fruit subclass. In addition to these qualities, the fruit class has specific characteristics (juicy, sweet, etc.) that distinguish it from other food. The apple class defines those qualities specific to an apple (grows on trees, not tropical, etc.). A Red Delicious apple would, in turn, inherit all the qualities of all preceding classes, and would define only those qualities that make it unique. Without the use of hierarchies, each object would have to explicitly define all of its characteristics. However, using inheritance, an object needs to define only those qualities that make it unique within its class. It can inherit its general attributes from its parent. Thus, it is the inheritance mechanism that makes it possible for one object to be a specific instance of a more general case.C++ Implements OOP
As you will see as you progress through this book, many of the features of C++ exist to provide support for encapsulation, polyrnorphism, and inheritance. Remember, however, that you can use C++ to write any type of program, using any type of approach. The fact that C++ supports object-oriented programming does not mean that you can only write object-oriented programs. As with its predecessor, C, one of C++'s strongest advantages is its flexibility.How C++ Relates to Java
As you are probably aware, there is a relatively new force in computer programming languages, called Java. Java is the language of the Internet. It was strongly influenced by C++. Java and C++ both use the same basic syntax, and Java's object-oriented features are similar to C++'s. In fact, at first glance it is possible to mistake a Java program for a C++ program. Because of their surface similarities, it is a common misconception that Java is simply an alternative to C++. Before continuing, it is necessary to set the record straight on this account. Although related, Java and C++ were designed to solve differing sets of problems. C++ is optimized for the creation of high-performance programs. Towards this end, C++ compiles to highly efficient, executable code. Java is optimized for the creation of portable programs. To obtain portability, Java compiles to pseudo-code (called Java bytecode), which is usually interpreted. This makes Java code very portable, but not very efficient. Thus, Java is excellent for Internet applications, which must work on a wide variety of computers. But for high-performance programs, C++ will remain the language of choice. Because of the similarities between Java and C++, most C++ programmers can readily learn Java. The skills and knowledge you gain in C++ will translate easily. Likewise, if you already know Java, then you will have no trouble learning C++. Keep in mind, however, that some important differences do exist, so be careful not to jump to false conclusions.What You Will Need
To use this book effectively, you will need a computer and a C++ compiler. The best way to learn is to try the examples yourself, experimenting with your own variations. There really is no substitute for hands-on experience.If you are using a PC, then you have several C++ compilers from which to choose. just make sure that you use an up-to-date C++ compiler. The code in this book complies with Standard C++ and has been tested with Microsoft C++. It should work with virtually any contemporary C++ compiler. It is best, however, if you can use a compiler that accepts the version of C++ specified by the ANSI/ISO standards committee (i.e., Standard C++).
Now that you know the story behind C++, it is time to start learning how to use it. The next chapter introduces you to the C++ fundamentals...
Table of Contents
1: The Story of C++
2: An Overview of C++ 3: The Basic Data Types 4: Program Control Statements 5: Arrays and Strings 6: Pointers 7: Functions, Part One: The Fundamentals 8: Functions, Part Two: References, Overloading, and Default Arguments 9: More Data Types and Operators 10: Structures and Unions 11: Introducing the Class 12: A Closer Look at Classes 13: Operator Overloading 14: Inheritance 15: Virtual Functions and Polymorphism 16: Templates 17: Exception Handling 18: The C++ I/O System 19: Run-Time Type ID and the Casting Operators 20: Namespaces and Other Advanced Topics 21: Introducing the Standard Template Library 22: The C++ Preprocessor A: C-Based I/O B: Working with an Older C++ Compiler C: The .NET Managed Extensions to C++