C++ Plus Data Structures is designed for a course in Data Structures where C++ is the programming language. The book focuses on abstract data types as viewed from three different perspectives: their specification, their application, and their implementation. The authors stress computer science theory and software engineering principles, including modularization, data encapsulation, information hiding, data abstraction, object-oriented decomposition, functional decomposition, the analysis of algorithms, and life-cycle software verification methods. Finally, through classic Dale pedagogy students are offered a clear, easy-to-understand discussion of important theoretical constructs and their implementation in C++.
C++ Plus Data Structures is designed for a course in Data Structures where C++ is the programming language. The book focuses on abstract data types as viewed from three different perspectives: their specification, their application, and their implementation. The authors stress computer science theory and software engineering principles, including modularization, data encapsulation, information hiding, data abstraction, object-oriented decomposition, functional decomposition, the analysis of algorithms, and life-cycle software verification methods. Finally, through classic Dale pedagogy students are offered a clear, easy-to-understand discussion of important theoretical constructs and their implementation in C++.
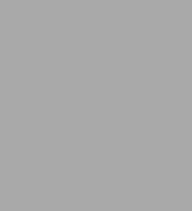
C++ Plus Data Structures, Third Edition
832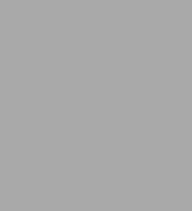
C++ Plus Data Structures, Third Edition
832Hardcover(Third Edition)
Related collections and offers
Product Details
ISBN-13: | 9780763704810 |
---|---|
Publisher: | Jones & Bartlett Learning |
Publication date: | 11/13/2003 |
Series: | Cs2 - C++ Series |
Edition description: | Third Edition |
Pages: | 832 |
Product dimensions: | 8.12(w) x 9.48(h) x 1.41(d) |