Classic Computer Science Problems in Java
Sharpen your coding skills by exploring established computer science problems! Classic Computer Science Problems in Java challenges you with time-tested scenarios and algorithms.
Summary
Sharpen your coding skills by exploring established computer science problems! Classic Computer Science Problems in Java challenges you with time-tested scenarios and algorithms. You’ll work through a series of exercises based in computer science fundamentals that are designed to improve your software development abilities, improve your understanding of artificial intelligence, and even prepare you to ace an interview. As you work through examples in search, clustering, graphs, and more, you'll remember important things you've forgotten and discover classic solutions to your "new" problems!
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Whatever software development problem you’re facing, odds are someone has already uncovered a solution. This book collects the most useful solutions devised, guiding you through a variety of challenges and tried-and-true problem-solving techniques. The principles and algorithms presented here are guaranteed to save you countless hours in project after project.
About the book
Classic Computer Science Problems in Java is a master class in computer programming designed around 55 exercises that have been used in computer science classrooms for years. You’ll work through hands-on examples as you explore core algorithms, constraint problems, AI applications, and much more.
What's inside
Recursion, memoization, and bit manipulation
Search, graph, and genetic algorithms
Constraint-satisfaction problems
K-means clustering, neural networks, and adversarial search
About the reader
For intermediate Java programmers.
About the author
David Kopec is an assistant professor of Computer Science and Innovation at Champlain College in Burlington, Vermont.
Table of Contents
1 Small problems
2 Search problems
3 Constraint-satisfaction problems
4 Graph problems
5 Genetic algorithms
6 K-means clustering
7 Fairly simple neural networks
8 Adversarial search
9 Miscellaneous problems
10 Interview with Brian Goetz
1137364544
Summary
Sharpen your coding skills by exploring established computer science problems! Classic Computer Science Problems in Java challenges you with time-tested scenarios and algorithms. You’ll work through a series of exercises based in computer science fundamentals that are designed to improve your software development abilities, improve your understanding of artificial intelligence, and even prepare you to ace an interview. As you work through examples in search, clustering, graphs, and more, you'll remember important things you've forgotten and discover classic solutions to your "new" problems!
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Whatever software development problem you’re facing, odds are someone has already uncovered a solution. This book collects the most useful solutions devised, guiding you through a variety of challenges and tried-and-true problem-solving techniques. The principles and algorithms presented here are guaranteed to save you countless hours in project after project.
About the book
Classic Computer Science Problems in Java is a master class in computer programming designed around 55 exercises that have been used in computer science classrooms for years. You’ll work through hands-on examples as you explore core algorithms, constraint problems, AI applications, and much more.
What's inside
Recursion, memoization, and bit manipulation
Search, graph, and genetic algorithms
Constraint-satisfaction problems
K-means clustering, neural networks, and adversarial search
About the reader
For intermediate Java programmers.
About the author
David Kopec is an assistant professor of Computer Science and Innovation at Champlain College in Burlington, Vermont.
Table of Contents
1 Small problems
2 Search problems
3 Constraint-satisfaction problems
4 Graph problems
5 Genetic algorithms
6 K-means clustering
7 Fairly simple neural networks
8 Adversarial search
9 Miscellaneous problems
10 Interview with Brian Goetz
Classic Computer Science Problems in Java
Sharpen your coding skills by exploring established computer science problems! Classic Computer Science Problems in Java challenges you with time-tested scenarios and algorithms.
Summary
Sharpen your coding skills by exploring established computer science problems! Classic Computer Science Problems in Java challenges you with time-tested scenarios and algorithms. You’ll work through a series of exercises based in computer science fundamentals that are designed to improve your software development abilities, improve your understanding of artificial intelligence, and even prepare you to ace an interview. As you work through examples in search, clustering, graphs, and more, you'll remember important things you've forgotten and discover classic solutions to your "new" problems!
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Whatever software development problem you’re facing, odds are someone has already uncovered a solution. This book collects the most useful solutions devised, guiding you through a variety of challenges and tried-and-true problem-solving techniques. The principles and algorithms presented here are guaranteed to save you countless hours in project after project.
About the book
Classic Computer Science Problems in Java is a master class in computer programming designed around 55 exercises that have been used in computer science classrooms for years. You’ll work through hands-on examples as you explore core algorithms, constraint problems, AI applications, and much more.
What's inside
Recursion, memoization, and bit manipulation
Search, graph, and genetic algorithms
Constraint-satisfaction problems
K-means clustering, neural networks, and adversarial search
About the reader
For intermediate Java programmers.
About the author
David Kopec is an assistant professor of Computer Science and Innovation at Champlain College in Burlington, Vermont.
Table of Contents
1 Small problems
2 Search problems
3 Constraint-satisfaction problems
4 Graph problems
5 Genetic algorithms
6 K-means clustering
7 Fairly simple neural networks
8 Adversarial search
9 Miscellaneous problems
10 Interview with Brian Goetz
Summary
Sharpen your coding skills by exploring established computer science problems! Classic Computer Science Problems in Java challenges you with time-tested scenarios and algorithms. You’ll work through a series of exercises based in computer science fundamentals that are designed to improve your software development abilities, improve your understanding of artificial intelligence, and even prepare you to ace an interview. As you work through examples in search, clustering, graphs, and more, you'll remember important things you've forgotten and discover classic solutions to your "new" problems!
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Whatever software development problem you’re facing, odds are someone has already uncovered a solution. This book collects the most useful solutions devised, guiding you through a variety of challenges and tried-and-true problem-solving techniques. The principles and algorithms presented here are guaranteed to save you countless hours in project after project.
About the book
Classic Computer Science Problems in Java is a master class in computer programming designed around 55 exercises that have been used in computer science classrooms for years. You’ll work through hands-on examples as you explore core algorithms, constraint problems, AI applications, and much more.
What's inside
Recursion, memoization, and bit manipulation
Search, graph, and genetic algorithms
Constraint-satisfaction problems
K-means clustering, neural networks, and adversarial search
About the reader
For intermediate Java programmers.
About the author
David Kopec is an assistant professor of Computer Science and Innovation at Champlain College in Burlington, Vermont.
Table of Contents
1 Small problems
2 Search problems
3 Constraint-satisfaction problems
4 Graph problems
5 Genetic algorithms
6 K-means clustering
7 Fairly simple neural networks
8 Adversarial search
9 Miscellaneous problems
10 Interview with Brian Goetz
49.99
In Stock
5
1
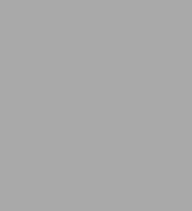
Classic Computer Science Problems in Java
264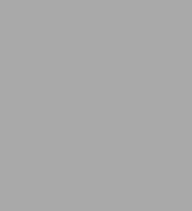
Classic Computer Science Problems in Java
264
49.99
In Stock
From the B&N Reads Blog