Core Python Applications Programming / Edition 3 available in Paperback, eBook
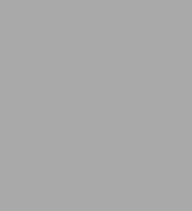
Core Python Applications Programming / Edition 3
- ISBN-10:
- 0132678209
- ISBN-13:
- 9780132678209
- Pub. Date:
- 03/23/2012
- Publisher:
- Prentice Hall
- ISBN-10:
- 0132678209
- ISBN-13:
- 9780132678209
- Pub. Date:
- 03/23/2012
- Publisher:
- Prentice Hall
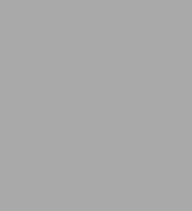
Core Python Applications Programming / Edition 3
Buy New
$54.99Buy Used
$32.01-
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
-
Overview
- Already know Python but want to learn more? A lot more? Dive into a variety of topics used in practice for real-world applications.
- Covers regular expressions, Internet/network programming, GUIs, SQL/databases/ORMs, threading, and Web development.
- Learn about contemporary development trends such as Google+, Twitter, MongoDB, OAuth, Python 3 migration, and Java/Jython. Presents brand new material on Django, Google App Engine, CSV/JSON/XML, and Microsoft Office. Includes Python 2 and 3 code samples to get you started right away!
- Provides code snippets, interactive examples, and practical exercises to help build your Python skills.
The Complete Developer’s Guide to Python
Python is an agile, robust, and expressive programming language that continues to build momentum. It combines the power of compiled languages with the simplicity and rapid development of scripting languages. In Core Python Applications Programming, Third Edition , leading Python developer and corporate trainer Wesley Chun helps you take your Python knowledge to the next level.
This book has everything you need to become a versatile Python developer. You will be introduced to multiple areas of application development and gain knowledge that can be immediately applied to projects, and you will find code samples in both Python 2 and 3, including migration tips if that’s on your roadmap too. Some snippets will even run unmodified on 2.x or 3.x.
- Learn professional Python style, best practices, and good programming habits
- Build clients and servers using TCP, UDP, XML-RPC, and be exposed to higher-level libraries like SocketServer and Twisted
- Develop GUI applications using Tkinter and other available toolkits
- Improve application performance by writing extensions in C/C++, or enhance I/O-bound code with multithreading
- Discover SQL and relational databases, ORMs, and even non-relational (NonSQL) databases like MongoDB
- Learn the basics of Web programming, including Web clients and servers, plus CGI and WSGI
- Expose yourself to regular expressions and powerful text processing tools for creating and parsing CSV, JSON, and XML data
- Interface with popular Microsoft Office applications such as Excel, PowerPoint, and Outlook using COM client programming
- Dive deeper into Web development with the Django framework and cloud computing with Google App Engine
- Explore Java programming with Jython, the way to run Python code on the JVM
- Connect to Web services Yahoo! Finance to get stock quotes, or Yahoo! Mail, Gmail, and others to download or send e-mail
- Jump into the social media craze by learning how to connect to the Twitter and Google+ networks
Core Python Applications Programming, Third Edition, delivers
- Broad coverage of a variety of areas of development used in real-world applications today
- Powerful insights into current and best practices for the intermediate Python programmer
- Dozens of code examples, from quick snippets to full-fledged applications
- A variety of exercises at the end of every chapter to help hammer the concepts home
Product Details
ISBN-13: | 9780132678209 |
---|---|
Publisher: | Prentice Hall |
Publication date: | 03/23/2012 |
Series: | Core Series |
Pages: | 888 |
Product dimensions: | 7.00(w) x 9.10(h) x 1.30(d) |
About the Author
Table of Contents
Preface xvAcknowledgments xxvii
About the Author xxxi
Part I: General Application Topics 1
Chapter 1: Regular Expressions 2
1.1 Introduction/Motivation 3
1.2 Special Symbols and Characters 6
1.3 Regexes and Python 16
1.4 Some Regex Examples 36
1.5 A Longer Regex Example 41
1.6 Exercises 48
Chapter 2: Network Programming 53
2.1 Introduction 54
2.2 What Is Client/Server Architecture? 54
2.3 Sockets: Communication Endpoints 58
2.4 Network Programming in Python 61
2.5 The SocketServer Module 79
2.6 Introduction to the Twisted Framework 84
2.7 Related Modules 88
2.8 Exercises 89
Chapter 3: Internet Client Programming 94
3.1 What Are Internet Clients? 95
3.2 Transferring Files 96
3.3 Network News 104
3.4 E-Mail 114
3.5 Related Modules 146
3.6 Exercises 148
Chapter 4: Multithreaded Programming 156
4.1 Introduction/Motivation 157
4.2 Threads and Processes 158
4.3 Threads and Python 160
4.4 The thread Module 164
4.5 The threading Module 169
4.6 Comparing Single vs. Multithreaded Execution 180
4.7 Multithreading in Practice 182
4.8 Producer-Consumer Problem and the Queue/queue Module 202
4.9 Alternative Considerations to Threads 206
4.10 Related Modules 209
4.11 Exercises 210
Chapter 5: GUI Programming 213
5.1 Introduction 214
5.2 Tkinter and Python Programming 216
5.3 Tkinter Examples 221
5.4 A Brief Tour of Other GUIs 236
5.5 Related Modules and Other GUIs 247
5.6 Exercises 250
Chapter 6: Database Programming 253
6.1 Introduction 254
6.2 The Python DB-API 259
6.3 ORMs 289
6.4 Non-Relational Databases 309
6.5 Related References 316
6.6 Exercises 319
Chapter 7: Programming Microsoft Office 324
7.1 Introduction 325
7.2 COM Client Programming with Python 326
7.3 Introductory Examples 328
7.4 Intermediate Examples 338
7.5 Related Modules/Packages 357
7.6 Exercises 357
Chapter 8: Extending Python 364
8.1 Introduction/Motivation 365
8.2 Extending Python by Writing Extensions 368
8.3 Related Topics 384
8.4 Exercises 388
Part II: Web Development 389
Chapter 9: Web Clients and Servers 390
9.1 Introduction 391
9.2 Python Web Client Tools 396
9.3 Web Clients 410
9.4 Web (HTTP) Servers 428
9.5 Related Modules 433
9.6 Exercises 436
Chapter 10: Web Programming: CGI and WSGI 441
10.1 Introduction 442
10.2 Helping Web Servers Process Client Data 442
10.3 Building CGI Applications 446
10.4 Using Unicode with CGI 464
10.5 Advanced CGI 466
10.6 Introduction to WSGI 478
10.7 Real-World Web Development 487
10.8 Related Modules 488
10.9 Exercises 490
Chapter 11: Web Frameworks: Django 493
11.1 Introduction 494
11.2 Web Frameworks 494
11.3 Introduction to Django 496
11.4 Projects and Apps 501
11.5 Your “Hello World” Application (A Blog) 507
11.6 Creating a Model to Add Database Service 509
11.7 The Python Application Shell 514
11.8 The Django Administration App 518
11.9 Creating the Blog’s User Interface 527
11.10 Improving the Output 537
11.11 Working with User Input 542
11.12 Forms and Model Forms 546
11.13 More About Views 551
11.14 Look-and-Feel Improvements 553
11.15 Unit Testing 554
11.16 An Intermediate Django App: The TweetApprover 564
11.17 Resources 597
11.18 Conclusion 597
11.19 Exercises 598
Chapter 12: Cloud Computing: Google App Engine 604
12.1 Introduction 605
12.2 What Is Cloud Computing? 605
12.3 The Sandbox and the App Engine SDK 612
12.4 Choosing an App Engine Framework 617
12.5 Python 2.7 Support 626
12.6 Comparisons to Django 628
12.7 Morphing “Hello World” into a Simple Blog 631
12.8 Adding Memcache Service 647
12.9 Static Files 651
12.10 Adding Users Service 652
12.11 Remote API Shell 654
12.12 Lightning Round (with Python Code) 656
12.13 Sending Instant Messages by Using XMPP 660
12.14 Processing Images 662
12.15 Task Queues (Unscheduled Tasks) 663
12.16 Profiling with Appstats 670
12.17 The URLfetch Service 672
12.18 Lightning Round (without Python Code) 673
12.19 Vendor Lock-In 675
12.20 Resources 676
12.21 Conclusion 679
12.22 Exercises 680
Chapter 13: Web Services 684
13.1 Introduction 685
13.2 The Yahoo! Finance Stock Quote Server 685
13.3 Microblogging with Twitter 690
13.4 Exercises 707
Part III: Supplemental/Experimental 713
Chapter 14: Text Processing 714
14.1 Comma-Separated Values 715
14.2 JavaScript Object Notation 719
14.3 Extensible Markup Language 724
14.4 References 738
14.5 Related Modules 740
14.6 Exercises 740
Chapter 15: Miscellaneous 743
15.1 Jython 744
15.2 Google+ 748
15.3 Exercises 759
Appendix A: Answers to Selected Exercises 763
Appendix B: Reference Tables 768
Appendix C: Python 3: The Evolution of a Programming Language 798
C.1 Why Is Python Changing? 799
C.2 What Has Changed? 799
C.3 Migration Tools 805
C.4 Conclusion 806
C.5 References 806
Appendix D: Python 3 Migration with 2.6+ 807
D.1 Python 3: The Next Generation 807
D.2 Integers 809
D.3 Built-In Functions 812
D.4 Object-Oriented Programming: Two Different Class Objects 814
D.5 Strings 815
D.6 Exceptions 816
D.7 Other Transition Tools and Tips 817
D.8 Writing Code That is Compatible in Both Versions 2.x and 3.x 818
D.9 Conclusion 822
Index 823