5
1
9780132129480
Data Structures and Other Objects Using C++ / Edition 4 available in Paperback
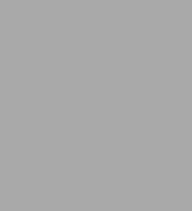
Data Structures and Other Objects Using C++ / Edition 4
- ISBN-10:
- 0132129485
- ISBN-13:
- 9780132129480
- Pub. Date:
- 02/24/2010
- Publisher:
- Pearson Education
- ISBN-10:
- 0132129485
- ISBN-13:
- 9780132129480
- Pub. Date:
- 02/24/2010
- Publisher:
- Pearson Education
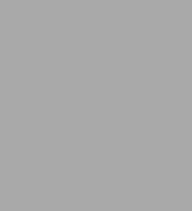
Data Structures and Other Objects Using C++ / Edition 4
$283.72
Current price is , Original price is $283.72. You
283.72
In Stock
Product Details
ISBN-13: | 9780132129480 |
---|---|
Publisher: | Pearson Education |
Publication date: | 02/24/2010 |
Pages: | 856 |
Product dimensions: | 7.30(w) x 9.10(h) x 1.30(d) |
About the Author
From the B&N Reads Blog