Full Stack Python Security: Cryptography, TLS, and attack resistance
Full Stack Python Security teaches you everything you’ll need to build secure Python web applications.
Summary
In Full Stack Python Security: Cryptography, TLS, and attack resistance, you’ll learn how to:
Use algorithms to encrypt, hash, and digitally sign data
Create and install TLS certificates
Implement authentication, authorization, OAuth 2.0, and form validation in Django
Protect a web application with Content Security Policy
Implement Cross Origin Resource Sharing
Protect against common attacks including clickjacking, denial of service attacks, SQL injection, cross-site scripting, and more
Full Stack Python Security: Cryptography, TLS, and attack resistance teaches you everything you’ll need to build secure Python web applications. As you work through the insightful code snippets and engaging examples, you’ll put security standards, best practices, and more into action. Along the way, you’ll get exposure to important libraries and tools in the Python ecosystem.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Security is a full-stack concern, encompassing user interfaces, APIs, web servers, network infrastructure, and everything in between. Master the powerful libraries, frameworks, and tools in the Python ecosystem and you can protect your systems top to bottom. Packed with realistic examples, lucid illustrations, and working code, this book shows you exactly how to secure Python-based web applications.
About the book
Full Stack Python Security: Cryptography, TLS, and attack resistance teaches you everything you need to secure Python and Django-based web apps. In it, seasoned security pro Dennis Byrne demystifies complex security terms and algorithms. Starting with a clear review of cryptographic foundations, you’ll learn how to implement layers of defense, secure user authentication and third-party access, and protect your applications against common hacks.
What's inside
Encrypt, hash, and digitally sign data
Create and install TLS certificates
Implement authentication, authorization, OAuth 2.0, and form validation in Django
Protect against attacks such as clickjacking, cross-site scripting, and SQL injection
About the reader
For intermediate Python programmers.
About the author
Dennis Byrne is a tech lead for 23andMe, where he protects the genetic data of more than 10 million customers.
Table of Contents
1 Defense in depth
PART 1 - CRYPTOGRAPHIC FOUNDATIONS
2 Hashing
3 Keyed hashing
4 Symmetric encryption
5 Asymmetric encryption
6 Transport Layer Security
PART 2 - AUTHENTICATION AND AUTHORIZATION
7 HTTP session management
8 User authentication
9 User password management
10 Authorization
11 OAuth 2
PART 3 - ATTACK RESISTANCE
12 Working with the operating system
13 Never trust input
14 Cross-site scripting attacks
15 Content Security Policy
16 Cross-site request forgery
17 Cross-Origin Resource Sharing
18 Clickjacking
1139359090
Summary
In Full Stack Python Security: Cryptography, TLS, and attack resistance, you’ll learn how to:
Use algorithms to encrypt, hash, and digitally sign data
Create and install TLS certificates
Implement authentication, authorization, OAuth 2.0, and form validation in Django
Protect a web application with Content Security Policy
Implement Cross Origin Resource Sharing
Protect against common attacks including clickjacking, denial of service attacks, SQL injection, cross-site scripting, and more
Full Stack Python Security: Cryptography, TLS, and attack resistance teaches you everything you’ll need to build secure Python web applications. As you work through the insightful code snippets and engaging examples, you’ll put security standards, best practices, and more into action. Along the way, you’ll get exposure to important libraries and tools in the Python ecosystem.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Security is a full-stack concern, encompassing user interfaces, APIs, web servers, network infrastructure, and everything in between. Master the powerful libraries, frameworks, and tools in the Python ecosystem and you can protect your systems top to bottom. Packed with realistic examples, lucid illustrations, and working code, this book shows you exactly how to secure Python-based web applications.
About the book
Full Stack Python Security: Cryptography, TLS, and attack resistance teaches you everything you need to secure Python and Django-based web apps. In it, seasoned security pro Dennis Byrne demystifies complex security terms and algorithms. Starting with a clear review of cryptographic foundations, you’ll learn how to implement layers of defense, secure user authentication and third-party access, and protect your applications against common hacks.
What's inside
Encrypt, hash, and digitally sign data
Create and install TLS certificates
Implement authentication, authorization, OAuth 2.0, and form validation in Django
Protect against attacks such as clickjacking, cross-site scripting, and SQL injection
About the reader
For intermediate Python programmers.
About the author
Dennis Byrne is a tech lead for 23andMe, where he protects the genetic data of more than 10 million customers.
Table of Contents
1 Defense in depth
PART 1 - CRYPTOGRAPHIC FOUNDATIONS
2 Hashing
3 Keyed hashing
4 Symmetric encryption
5 Asymmetric encryption
6 Transport Layer Security
PART 2 - AUTHENTICATION AND AUTHORIZATION
7 HTTP session management
8 User authentication
9 User password management
10 Authorization
11 OAuth 2
PART 3 - ATTACK RESISTANCE
12 Working with the operating system
13 Never trust input
14 Cross-site scripting attacks
15 Content Security Policy
16 Cross-site request forgery
17 Cross-Origin Resource Sharing
18 Clickjacking
Full Stack Python Security: Cryptography, TLS, and attack resistance
Full Stack Python Security teaches you everything you’ll need to build secure Python web applications.
Summary
In Full Stack Python Security: Cryptography, TLS, and attack resistance, you’ll learn how to:
Use algorithms to encrypt, hash, and digitally sign data
Create and install TLS certificates
Implement authentication, authorization, OAuth 2.0, and form validation in Django
Protect a web application with Content Security Policy
Implement Cross Origin Resource Sharing
Protect against common attacks including clickjacking, denial of service attacks, SQL injection, cross-site scripting, and more
Full Stack Python Security: Cryptography, TLS, and attack resistance teaches you everything you’ll need to build secure Python web applications. As you work through the insightful code snippets and engaging examples, you’ll put security standards, best practices, and more into action. Along the way, you’ll get exposure to important libraries and tools in the Python ecosystem.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Security is a full-stack concern, encompassing user interfaces, APIs, web servers, network infrastructure, and everything in between. Master the powerful libraries, frameworks, and tools in the Python ecosystem and you can protect your systems top to bottom. Packed with realistic examples, lucid illustrations, and working code, this book shows you exactly how to secure Python-based web applications.
About the book
Full Stack Python Security: Cryptography, TLS, and attack resistance teaches you everything you need to secure Python and Django-based web apps. In it, seasoned security pro Dennis Byrne demystifies complex security terms and algorithms. Starting with a clear review of cryptographic foundations, you’ll learn how to implement layers of defense, secure user authentication and third-party access, and protect your applications against common hacks.
What's inside
Encrypt, hash, and digitally sign data
Create and install TLS certificates
Implement authentication, authorization, OAuth 2.0, and form validation in Django
Protect against attacks such as clickjacking, cross-site scripting, and SQL injection
About the reader
For intermediate Python programmers.
About the author
Dennis Byrne is a tech lead for 23andMe, where he protects the genetic data of more than 10 million customers.
Table of Contents
1 Defense in depth
PART 1 - CRYPTOGRAPHIC FOUNDATIONS
2 Hashing
3 Keyed hashing
4 Symmetric encryption
5 Asymmetric encryption
6 Transport Layer Security
PART 2 - AUTHENTICATION AND AUTHORIZATION
7 HTTP session management
8 User authentication
9 User password management
10 Authorization
11 OAuth 2
PART 3 - ATTACK RESISTANCE
12 Working with the operating system
13 Never trust input
14 Cross-site scripting attacks
15 Content Security Policy
16 Cross-site request forgery
17 Cross-Origin Resource Sharing
18 Clickjacking
Summary
In Full Stack Python Security: Cryptography, TLS, and attack resistance, you’ll learn how to:
Use algorithms to encrypt, hash, and digitally sign data
Create and install TLS certificates
Implement authentication, authorization, OAuth 2.0, and form validation in Django
Protect a web application with Content Security Policy
Implement Cross Origin Resource Sharing
Protect against common attacks including clickjacking, denial of service attacks, SQL injection, cross-site scripting, and more
Full Stack Python Security: Cryptography, TLS, and attack resistance teaches you everything you’ll need to build secure Python web applications. As you work through the insightful code snippets and engaging examples, you’ll put security standards, best practices, and more into action. Along the way, you’ll get exposure to important libraries and tools in the Python ecosystem.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Security is a full-stack concern, encompassing user interfaces, APIs, web servers, network infrastructure, and everything in between. Master the powerful libraries, frameworks, and tools in the Python ecosystem and you can protect your systems top to bottom. Packed with realistic examples, lucid illustrations, and working code, this book shows you exactly how to secure Python-based web applications.
About the book
Full Stack Python Security: Cryptography, TLS, and attack resistance teaches you everything you need to secure Python and Django-based web apps. In it, seasoned security pro Dennis Byrne demystifies complex security terms and algorithms. Starting with a clear review of cryptographic foundations, you’ll learn how to implement layers of defense, secure user authentication and third-party access, and protect your applications against common hacks.
What's inside
Encrypt, hash, and digitally sign data
Create and install TLS certificates
Implement authentication, authorization, OAuth 2.0, and form validation in Django
Protect against attacks such as clickjacking, cross-site scripting, and SQL injection
About the reader
For intermediate Python programmers.
About the author
Dennis Byrne is a tech lead for 23andMe, where he protects the genetic data of more than 10 million customers.
Table of Contents
1 Defense in depth
PART 1 - CRYPTOGRAPHIC FOUNDATIONS
2 Hashing
3 Keyed hashing
4 Symmetric encryption
5 Asymmetric encryption
6 Transport Layer Security
PART 2 - AUTHENTICATION AND AUTHORIZATION
7 HTTP session management
8 User authentication
9 User password management
10 Authorization
11 OAuth 2
PART 3 - ATTACK RESISTANCE
12 Working with the operating system
13 Never trust input
14 Cross-site scripting attacks
15 Content Security Policy
16 Cross-site request forgery
17 Cross-Origin Resource Sharing
18 Clickjacking
59.99
In Stock
5
1
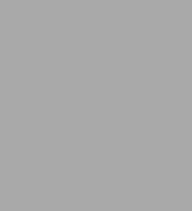
Full Stack Python Security: Cryptography, TLS, and attack resistance
306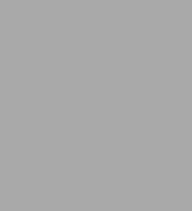
Full Stack Python Security: Cryptography, TLS, and attack resistance
306
59.99
In Stock
From the B&N Reads Blog