You'll start by learning the core features of Selenium (composed of WebDriver, Grid, and IDE) and its ecosystem. Discover why Selenium WebDriver is the de facto library for developing end-to-end tests on your web application. You'll explore ways to use advanced Selenium WebDriver features, including using web browsers in Docker containers or the DevTools protocol. Selenium WebDriver examples in this book are available on GitHub.
With this book, you'll learn how to:
- Set up a Java project containing end-to-end tests that use Selenium WebDriver
- Conduct automated interaction with web applications
- Use strategies for managing browser-specific capabilities and cross-browser testing
- Interact with web forms, manage pop-up messages, and execute JavaScript
- Control remote browsers and use advanced browser infrastructure for Selenium WebDriver tests in the cloud
- Model web pages using object-oriented classes to ease test maintenance and reduce code duplication
You'll start by learning the core features of Selenium (composed of WebDriver, Grid, and IDE) and its ecosystem. Discover why Selenium WebDriver is the de facto library for developing end-to-end tests on your web application. You'll explore ways to use advanced Selenium WebDriver features, including using web browsers in Docker containers or the DevTools protocol. Selenium WebDriver examples in this book are available on GitHub.
With this book, you'll learn how to:
- Set up a Java project containing end-to-end tests that use Selenium WebDriver
- Conduct automated interaction with web applications
- Use strategies for managing browser-specific capabilities and cross-browser testing
- Interact with web forms, manage pop-up messages, and execute JavaScript
- Control remote browsers and use advanced browser infrastructure for Selenium WebDriver tests in the cloud
- Model web pages using object-oriented classes to ease test maintenance and reduce code duplication
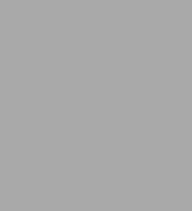
Hands-On Selenium WebDriver with Java: A Deep Dive into the Development of End-to-End Tests
419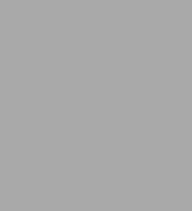
Hands-On Selenium WebDriver with Java: A Deep Dive into the Development of End-to-End Tests
419Product Details
ISBN-13: | 9781098110000 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 05/10/2022 |
Pages: | 419 |
Product dimensions: | 7.00(w) x 9.19(h) x (d) |