iOS Application Security: The Definitive Guide for Hackers and Developers
Eliminating security holes in iOS apps is critical for any developer who wants to protect their users from the bad guys. In iOS Application Security, mobile security expert David Thiel reveals common iOS coding mistakes that create serious security problems and shows you how to find and fix them.
After a crash course on iOS application structure and Objective-C design patterns, you’ll move on to spotting bad code and plugging the holes. You’ll learn about:
–The iOS security model and the limits of its built-in protections
–The myriad ways sensitive data can leak into places it shouldn’t, such as through the pasteboard
–How to implement encryption with the Keychain, the Data Protection API, and CommonCrypto
–Legacy flaws from C that still cause problems in modern iOS applications
–Privacy issues related to gathering user data and how to mitigate potential pitfalls
Don’t let your app’s security leak become another headline. Whether you’re looking to bolster your app’s defenses or hunting bugs in other people’s code, iOS Application Security will help you get the job done well.
1119966124
After a crash course on iOS application structure and Objective-C design patterns, you’ll move on to spotting bad code and plugging the holes. You’ll learn about:
–The iOS security model and the limits of its built-in protections
–The myriad ways sensitive data can leak into places it shouldn’t, such as through the pasteboard
–How to implement encryption with the Keychain, the Data Protection API, and CommonCrypto
–Legacy flaws from C that still cause problems in modern iOS applications
–Privacy issues related to gathering user data and how to mitigate potential pitfalls
Don’t let your app’s security leak become another headline. Whether you’re looking to bolster your app’s defenses or hunting bugs in other people’s code, iOS Application Security will help you get the job done well.
iOS Application Security: The Definitive Guide for Hackers and Developers
Eliminating security holes in iOS apps is critical for any developer who wants to protect their users from the bad guys. In iOS Application Security, mobile security expert David Thiel reveals common iOS coding mistakes that create serious security problems and shows you how to find and fix them.
After a crash course on iOS application structure and Objective-C design patterns, you’ll move on to spotting bad code and plugging the holes. You’ll learn about:
–The iOS security model and the limits of its built-in protections
–The myriad ways sensitive data can leak into places it shouldn’t, such as through the pasteboard
–How to implement encryption with the Keychain, the Data Protection API, and CommonCrypto
–Legacy flaws from C that still cause problems in modern iOS applications
–Privacy issues related to gathering user data and how to mitigate potential pitfalls
Don’t let your app’s security leak become another headline. Whether you’re looking to bolster your app’s defenses or hunting bugs in other people’s code, iOS Application Security will help you get the job done well.
After a crash course on iOS application structure and Objective-C design patterns, you’ll move on to spotting bad code and plugging the holes. You’ll learn about:
–The iOS security model and the limits of its built-in protections
–The myriad ways sensitive data can leak into places it shouldn’t, such as through the pasteboard
–How to implement encryption with the Keychain, the Data Protection API, and CommonCrypto
–Legacy flaws from C that still cause problems in modern iOS applications
–Privacy issues related to gathering user data and how to mitigate potential pitfalls
Don’t let your app’s security leak become another headline. Whether you’re looking to bolster your app’s defenses or hunting bugs in other people’s code, iOS Application Security will help you get the job done well.
49.95
In Stock
5
1
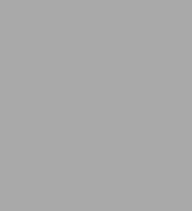
iOS Application Security: The Definitive Guide for Hackers and Developers
296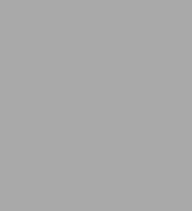
iOS Application Security: The Definitive Guide for Hackers and Developers
296
49.95
In Stock
Product Details
ISBN-13: | 9781593276010 |
---|---|
Publisher: | No Starch Press |
Publication date: | 02/16/2016 |
Pages: | 296 |
Product dimensions: | 6.90(w) x 9.10(h) x 0.90(d) |
About the Author
From the B&N Reads Blog