Isomorphic Web Applications: Universal Development with React
Summary
Isomorphic Web Applications teaches you to build production-quality web apps using isomorphic architecture. Designed for working developers, this book offers examples in relevant frameworks like React, Redux, Angular, Ember, and webpack.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Build secure web apps that perform beautifully with high, low, or no bandwidth. Isomorphic web apps employ a pattern that exploits the full stack, storing data locally and minimizing server hits. They render flawlessly, maximize SEO, and offer opportunities to share code and libraries between client and server.
About the Book
Isomorphic Web Applications teaches you to build production-quality web apps using isomorphic architecture. You'll learn to create and render views for both server and browser, optimize local storage, streamline server interactions, and handle data serialization. Designed for working developers, this book offers examples in relevant frameworks like React, Redux, Angular, Ember, and webpack. You'll also explore unique debugging and testing techniques and master specific SEO skills.
What's Inside
About the Reader
To benefit from this book, readers need to know JavaScript, HTML5, and a framework of their choice, including React and Angular.
About the Author
Elyse Kolker Gordon runs the growth engineering team at Strava. Previously, she was director of web engineering at Vevo, where she regularly solved challenges with isomorphic apps.
Table of Contents
1135862547
Isomorphic Web Applications teaches you to build production-quality web apps using isomorphic architecture. Designed for working developers, this book offers examples in relevant frameworks like React, Redux, Angular, Ember, and webpack.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Build secure web apps that perform beautifully with high, low, or no bandwidth. Isomorphic web apps employ a pattern that exploits the full stack, storing data locally and minimizing server hits. They render flawlessly, maximize SEO, and offer opportunities to share code and libraries between client and server.
About the Book
Isomorphic Web Applications teaches you to build production-quality web apps using isomorphic architecture. You'll learn to create and render views for both server and browser, optimize local storage, streamline server interactions, and handle data serialization. Designed for working developers, this book offers examples in relevant frameworks like React, Redux, Angular, Ember, and webpack. You'll also explore unique debugging and testing techniques and master specific SEO skills.
What's Inside
- Controlling browser and server user sessions
- Combining server-rendered and SPA architectures
- Building best-practice React applications
- Debugging and testing
About the Reader
To benefit from this book, readers need to know JavaScript, HTML5, and a framework of their choice, including React and Angular.
About the Author
Elyse Kolker Gordon runs the growth engineering team at Strava. Previously, she was director of web engineering at Vevo, where she regularly solved challenges with isomorphic apps.
Table of Contents
- PART 1 - FIRST STEPS
- Introduction to isomorphic web application architecture
- A sample isomorphic app PART 2 - ISOMORPHIC APP BASICS
- React overview
- Applying React
- Tools: webpack and Babel
- Redux PART 3 - ISOMORPHIC ARCHITECTURE
- Building the server
- Isomorphic view rendering
- Testing and debugging
- Handling server/browser differences 203
- Optimizing for production PART 4 - APPLYING ISOMORPHIC ARCHITECTURE WITH OTHER TOOLS
- Other frameworks: implementing isomorphic without React
- Where to go from here
Isomorphic Web Applications: Universal Development with React
Summary
Isomorphic Web Applications teaches you to build production-quality web apps using isomorphic architecture. Designed for working developers, this book offers examples in relevant frameworks like React, Redux, Angular, Ember, and webpack.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Build secure web apps that perform beautifully with high, low, or no bandwidth. Isomorphic web apps employ a pattern that exploits the full stack, storing data locally and minimizing server hits. They render flawlessly, maximize SEO, and offer opportunities to share code and libraries between client and server.
About the Book
Isomorphic Web Applications teaches you to build production-quality web apps using isomorphic architecture. You'll learn to create and render views for both server and browser, optimize local storage, streamline server interactions, and handle data serialization. Designed for working developers, this book offers examples in relevant frameworks like React, Redux, Angular, Ember, and webpack. You'll also explore unique debugging and testing techniques and master specific SEO skills.
What's Inside
About the Reader
To benefit from this book, readers need to know JavaScript, HTML5, and a framework of their choice, including React and Angular.
About the Author
Elyse Kolker Gordon runs the growth engineering team at Strava. Previously, she was director of web engineering at Vevo, where she regularly solved challenges with isomorphic apps.
Table of Contents
Isomorphic Web Applications teaches you to build production-quality web apps using isomorphic architecture. Designed for working developers, this book offers examples in relevant frameworks like React, Redux, Angular, Ember, and webpack.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Build secure web apps that perform beautifully with high, low, or no bandwidth. Isomorphic web apps employ a pattern that exploits the full stack, storing data locally and minimizing server hits. They render flawlessly, maximize SEO, and offer opportunities to share code and libraries between client and server.
About the Book
Isomorphic Web Applications teaches you to build production-quality web apps using isomorphic architecture. You'll learn to create and render views for both server and browser, optimize local storage, streamline server interactions, and handle data serialization. Designed for working developers, this book offers examples in relevant frameworks like React, Redux, Angular, Ember, and webpack. You'll also explore unique debugging and testing techniques and master specific SEO skills.
What's Inside
- Controlling browser and server user sessions
- Combining server-rendered and SPA architectures
- Building best-practice React applications
- Debugging and testing
About the Reader
To benefit from this book, readers need to know JavaScript, HTML5, and a framework of their choice, including React and Angular.
About the Author
Elyse Kolker Gordon runs the growth engineering team at Strava. Previously, she was director of web engineering at Vevo, where she regularly solved challenges with isomorphic apps.
Table of Contents
- PART 1 - FIRST STEPS
- Introduction to isomorphic web application architecture
- A sample isomorphic app PART 2 - ISOMORPHIC APP BASICS
- React overview
- Applying React
- Tools: webpack and Babel
- Redux PART 3 - ISOMORPHIC ARCHITECTURE
- Building the server
- Isomorphic view rendering
- Testing and debugging
- Handling server/browser differences 203
- Optimizing for production PART 4 - APPLYING ISOMORPHIC ARCHITECTURE WITH OTHER TOOLS
- Other frameworks: implementing isomorphic without React
- Where to go from here
39.99
In Stock
5
1
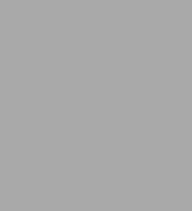
Isomorphic Web Applications: Universal Development with React
320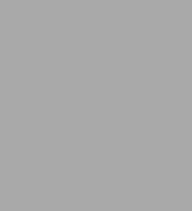
Isomorphic Web Applications: Universal Development with React
320Paperback(1st Edition)
$39.99
39.99
In Stock
From the B&N Reads Blog