JavaScript Application Design: A Build First Approach
Summary
JavaScript Application Design: A Build First Approach introduces JavaScript developers to techniques that will improve the quality of their software as well as their web development workflow. You'll begin by learning how to establish build processes that are appropriate for JavaScript-driven development. Then, you'll walk through best practices for productive day-to-day development, like running tasks when your code changes, deploying applications with a single command, and monitoring the state of your application once it's in production.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Book
The fate of most applications is often sealed before a single line of code has been written. How is that possible? Simply, bad design assures bad results. Good design and effective processes are the foundation on which maintainable applications are built, scaled, and improved. For JavaScript developers, this means discovering the tooling, modern libraries, and architectural patterns that enable those improvements.
JavaScript Application Design: A Build First Approach introduces techniques to improve software quality and development workflow. You'll begin by learning how to establish processes designed to optimize the quality of your work. You'll execute tasks whenever your code changes, run tests on every commit, and deploy in an automated fashion. Then you'll focus on designing modular components and composing them together to build robust applications.
This book assumes readers understand the basics of JavaScript.
What's Inside
About the Author
Nicolas Bevacqua is a freelance developer with a focus on modular JavaScript, build processes, and sharp design. He maintains a blog at ponyfoo.com.
Table of Contents
1119920896
JavaScript Application Design: A Build First Approach introduces JavaScript developers to techniques that will improve the quality of their software as well as their web development workflow. You'll begin by learning how to establish build processes that are appropriate for JavaScript-driven development. Then, you'll walk through best practices for productive day-to-day development, like running tasks when your code changes, deploying applications with a single command, and monitoring the state of your application once it's in production.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Book
The fate of most applications is often sealed before a single line of code has been written. How is that possible? Simply, bad design assures bad results. Good design and effective processes are the foundation on which maintainable applications are built, scaled, and improved. For JavaScript developers, this means discovering the tooling, modern libraries, and architectural patterns that enable those improvements.
JavaScript Application Design: A Build First Approach introduces techniques to improve software quality and development workflow. You'll begin by learning how to establish processes designed to optimize the quality of your work. You'll execute tasks whenever your code changes, run tests on every commit, and deploy in an automated fashion. Then you'll focus on designing modular components and composing them together to build robust applications.
This book assumes readers understand the basics of JavaScript.
What's Inside
- Automated development, testing, and deployment processes
- JavaScript fundamentals and modularity best practices
- Modular, maintainable, and well-tested applications
- Master asynchronous flows, embrace MVC, and design a REST API
About the Author
Nicolas Bevacqua is a freelance developer with a focus on modular JavaScript, build processes, and sharp design. He maintains a blog at ponyfoo.com.
Table of Contents
- Introduction to Build First
- Composing build tasks and flows
- Mastering environments and the development workflow
- Release, deployment, and monitoring Embracing modularity and dependency management
- Understanding asynchronous flow control methods in JavaScript
- Leveraging the Model-View-Controller
- Testing JavaScript components
- REST API design and layered service architectures
JavaScript Application Design: A Build First Approach
Summary
JavaScript Application Design: A Build First Approach introduces JavaScript developers to techniques that will improve the quality of their software as well as their web development workflow. You'll begin by learning how to establish build processes that are appropriate for JavaScript-driven development. Then, you'll walk through best practices for productive day-to-day development, like running tasks when your code changes, deploying applications with a single command, and monitoring the state of your application once it's in production.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Book
The fate of most applications is often sealed before a single line of code has been written. How is that possible? Simply, bad design assures bad results. Good design and effective processes are the foundation on which maintainable applications are built, scaled, and improved. For JavaScript developers, this means discovering the tooling, modern libraries, and architectural patterns that enable those improvements.
JavaScript Application Design: A Build First Approach introduces techniques to improve software quality and development workflow. You'll begin by learning how to establish processes designed to optimize the quality of your work. You'll execute tasks whenever your code changes, run tests on every commit, and deploy in an automated fashion. Then you'll focus on designing modular components and composing them together to build robust applications.
This book assumes readers understand the basics of JavaScript.
What's Inside
About the Author
Nicolas Bevacqua is a freelance developer with a focus on modular JavaScript, build processes, and sharp design. He maintains a blog at ponyfoo.com.
Table of Contents
JavaScript Application Design: A Build First Approach introduces JavaScript developers to techniques that will improve the quality of their software as well as their web development workflow. You'll begin by learning how to establish build processes that are appropriate for JavaScript-driven development. Then, you'll walk through best practices for productive day-to-day development, like running tasks when your code changes, deploying applications with a single command, and monitoring the state of your application once it's in production.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Book
The fate of most applications is often sealed before a single line of code has been written. How is that possible? Simply, bad design assures bad results. Good design and effective processes are the foundation on which maintainable applications are built, scaled, and improved. For JavaScript developers, this means discovering the tooling, modern libraries, and architectural patterns that enable those improvements.
JavaScript Application Design: A Build First Approach introduces techniques to improve software quality and development workflow. You'll begin by learning how to establish processes designed to optimize the quality of your work. You'll execute tasks whenever your code changes, run tests on every commit, and deploy in an automated fashion. Then you'll focus on designing modular components and composing them together to build robust applications.
This book assumes readers understand the basics of JavaScript.
What's Inside
- Automated development, testing, and deployment processes
- JavaScript fundamentals and modularity best practices
- Modular, maintainable, and well-tested applications
- Master asynchronous flows, embrace MVC, and design a REST API
About the Author
Nicolas Bevacqua is a freelance developer with a focus on modular JavaScript, build processes, and sharp design. He maintains a blog at ponyfoo.com.
Table of Contents
- Introduction to Build First
- Composing build tasks and flows
- Mastering environments and the development workflow
- Release, deployment, and monitoring Embracing modularity and dependency management
- Understanding asynchronous flow control methods in JavaScript
- Leveraging the Model-View-Controller
- Testing JavaScript components
- REST API design and layered service architectures
39.99
In Stock
5
1
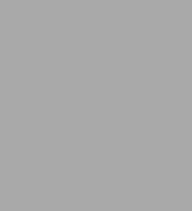
JavaScript Application Design: A Build First Approach
344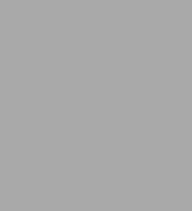
JavaScript Application Design: A Build First Approach
344Paperback(1st Edition)
$39.99
39.99
In Stock
From the B&N Reads Blog