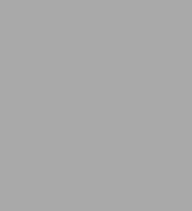
JavaScript: Optimizing Native JavaScript: Designing, Programming, and Debugging Native JavaScript Applications
180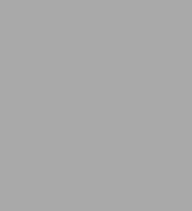
JavaScript: Optimizing Native JavaScript: Designing, Programming, and Debugging Native JavaScript Applications
180Paperback
-
SHIP THIS ITEMIn stock. Ships in 1-2 days.PICK UP IN STORE
Your local store may have stock of this item.
Available within 2 business hours
Related collections and offers
Overview
Product Details
ISBN-13: | 9780986307638 |
---|---|
Publisher: | MiraVista Press |
Publication date: | 05/08/2017 |
Pages: | 180 |
Product dimensions: | 7.40(w) x 9.40(h) x 0.50(d) |
About the Author
Read an Excerpt
JavaScript: Optimizing Native JavaScript
Designing, Programming, and Debugging Native JavaScript Applications
By Robert C. Etheredge
MiraVista Press
Copyright © 2017 MiraVista PressAll rights reserved.
ISBN: 978-0-9863076-5-2
CHAPTER 1
Basic Principles
There are a number of principles you should keep in mind during all stages of your application process, from design to implementation. These principles help drive your coding style and influence all the decisions you have to make during the project. I have been surprised at the number of times they are ignored.
Problems often arise when you are interfacing between different groups involved in the application process. If these groups are not aware of the limitations (and strengths) of JavaScript/HTML, it is often easy for them to overlook some basic design principles. The main outside groups that you will be concerned with are:
Designers. If designers treat each application UI screen as a separate HTML screen, then you will have problems designing an optimized application. They must understand that you are usually better off sharing as many screens and code as possible. Even if your application loads a number of separate screens, you are still better off using shared files since they can be cached for faster loading.
Server programmers. They may often ignore what is best for the application in designing their API's and data structures. They need to understand that their data packets have to be small and appropriate to the needs of the application. You need to work together to maximize the amount of data that can be cached by avoiding unique URL strings. The server data should be formatted to avoid having the client application reformat and remap any data. One of the advantages of the JSON data format is that it can be converted directly into JavaScript objects, reducing code size and garbage production. Parsing XML in JavaScript is much more difficult.
Principles
All things being equal---smaller and faster is better, unless it makes the code hard to understand or maintain. You often don't know what other programs or libraries will be loaded at the same time as your code, so make it as small and fast as you can. Even an extra 20 or 30 milliseconds of execution time may cause your UI to suffer in some cases, especially in fast-scrolling situations.
Size still matters
Smaller is better and bigger is ... well, bigger. It is easy to fall into complacency when programming your application on a powerful desktop computer with lots of memory. But you should always strive to reduce the memory footprint of every function that you code. This applies to the objects and arrays that you create, images that you place in your UI's, and all the data structures that you have to download or create. The reasons you need to keep it small include:
Mobile device users have to download code and data using limited bandwidth that they often have to pay for. And downloads over the cellular network are going to be slower than what you get on your regular Ethernet connection. Your users want their mobile applications to load and become functional as quickly as possible.
You don't necessarily know what other app or data will be loaded at the same time your app is. This is true of frameworks and libraries that may be loaded by your app, or by other apps that are running on the device at the same time.
Creating and destroying strings, or loading and deleting data, creates memory garbage. The way JavaScript is designed, you are unable to force a garbage collection (GC) from the code but must rely on the browser to decide when to force a GC. If this happens at the wrong time, such as when the user is fast scrolling a table, the UI can be adversely affected. So the rule is---just don't make the garbage to begin with.
Speed still matters
Faster is better. You should try to write all your functions to be as fast as possible while keeping the code readable. This does not mean optimizing your code at every step of the project. There is a good argument for waiting until your code logic is in place before optimizing your code. You often won't know what optimizing strategies are best until you have all pieces of the logic in place. But that doesn't mean that you can't apply the best principles as you are writing your code. The methods discussed in later chapters, such as using local variables or declaring an array length variable outside of the for loop, should always be used.
Flatter is better
Make your UI designs as flat as possible. This is much more important for single screen applications. Design your screens to include common navigation and filtering controls on the same screen without creating series of nested menus that the user must navigate to carry out required functions.
The server shouldn't make UI decisions
Make sure that the data delivered by the server doesn't restrict or change the functionality of your UI. If the server gives you dates in the wrong format, or unused data, or fields that are unnecessarily long, they are impacting the performance of your application. If the server can't give you enough data at one time to let you fill in your scrollable screens, your user's experience may suffer. If the server gives you excessive data that must be garbage collected on your end, your performance will suffer. Make sure the server team is involved in your design procedure early so they can deliver optimized and appropriate information. I had projects where the server team refused to optimize the data format delivered to the client, resulting in 160mb of data having to be delivered to a mobile device, greatly affecting the user experience. I was able to optimize that to a 3mb payload that the client received almost immediately, allowing almost immediate scrolling of all the data. Think of all the effort you may spend to minify your JavaScript content, but then you go and load huge data files that are unnecessarily large. Other incorrect server choices involve having dates formatted on the server instead of letting the user interface format them according to the user's preferences, and sub-optimal uses of booleans, strings, and numbers.
Share, share, share ...
Share code and screens as much as possible. I am not talking about writing your code to be reusable in other projects. That is a separate subject open for debate as it tends to make your project take longer. What I am talking about is making sure that you don't copy and paste the same routine in multiple places in your code. If you have to do the same thing more than once, spend the time to make one good function that can be called from several places. Put some more time in designing your application up front and create single functions that can be used in several places. The same also applies to your HTML code which can have more impact on your application's performance. Be careful when laying out your HTML screens so you reuse them as often as possible and avoid continual altering or destroying the DOM tree.
Make the appropriate trade-offs
There are almost always trade-offs when you are writing code. Your job as a programmer is to optimize for the proper value when you have a choice. The trade-offs are usually between:
memory use
execution speed
code readability
There hopefully will be many cases in which you can optimize for all three values. In other cases, you will have to analyze whether your UI demands fast execution, or whether you need to conserve memory. If you select a method that results in code that is more difficult to read, make sure that you properly comment the section as much as needed. Keep in mind a few general rules when optimizing your code:
Do not optimize prematurely. However, that doesn't mean that you shouldn't try to use best practices all the time.
Code readability is always important so be sure to comment any section of code that may be difficult to understand. Comments can be easily removed by minifiers when your app is released.
Focus on 'low hanging fruit' first. This follows Pareto's 80-20 principle. You can usually get 80% of the benefit from concentrating on just 20% of the code. Profile your code to determine where memory is used and where performance suffers. Then focus on those areas. Obvious areas of concern are recursive functions and loops.
How many lines of code do you write?
If you are ever asked how many lines of code you wrote, replying with a large number is not necessarily a good thing. Be proud of writing a smaller number of lines---just make them very good lines of code. As Mark Twain (or Blaise Pascal) is said to have commented--"I am sorry this letter is so long but I didn't have the time to make it shorter."
CHAPTER 2JavaScript Formatting
First, we'll go over the simple, but often contentious, subject of formatting your JavaScript code. This seems like a simple topic, but proper formatting greatly affects how easily others can read and maintain your code. It is particularly important when you have more than one programmer working on the same code base and how your version control software works. I include a number of suggestions here based on my experience with writing and particularly with sharing JavaScript code. Because of JavaScript's unique interpretive nature and global context structure, many of these items are more important than they are in compiled languages. It is important to decide on guidelines that will be used across your products. Your programming environment and code validator that you use will also influence your style as you want to be able to leverage those two tools.
NOTE: Some IDE environments or code validators may automatically format or at least suggest formatting changes to your code. These tools may decide a number of formatting conventions for you. You may want to decide on a formatting tool first and base your formatting guidelines on that tool.
Strict mode
JavaScript 1.8.5 added a new feature called strict mode. To invoke it, you include the expression "use strict" in your code at the beginning of the script or function where you want it to apply, using the following line of code:
"use strict";
It is designed to make it harder to write "bad" JavaScript code. Some of the sections in this book assume you are not running in strict mode. When set, strict mode will throw errors in the following situations:
Using a variable before it is declared
Deleting a variable or object using the delete method
Using the with statement
Restrictions on use of other variable names
arguments.callee is no longer supported
Comments
Comment style
Even something as simple as commenting needs to be handled consistently. In JavaScript, you can use two main types of comments and you may read books or articles recommending each type. Note: You need to use a different type of comment for CSS and HTML files. The comment types for your JavaScript code are:
1. // Two slashes anywhere on a line treats the remainder of that line as a comment. You can use this for blocks of code (each line starting with //), single line comments, or comments on the end of a line after the code.
2. /* */ Any code placed between the starting /* tag and an ending */ tag is treated as a comment. These tags can surround multiple lines of code, or just one line.
I strongly recommend using the first type of comments (//) in your code for three main reasons:
1. If you have a /* */ block and insert another /* */ block inside of it, it will most likely throw a JavaScript error.
2. When you do global searches for variable names, it makes it much easier to find the important strings that are real code and not commented code if you always use //. Otherwise, any code that is inside a block comment may look like valid code.
3. /* */ may fail if it surrounds regular expressions like: var rgex = /c*/;
One good reason for using block comments may be for temporary commenting out a block of code for testing purposes, knowing that you are going to remove the comments later.
What comments to include?
The amount of commenting should be dictated by your programming style, the clarity of your code, and the requirements of your project. Since you most likely will be compressing your code before it is deployed, you don't normally have to worry about the space taken up by your comments. However, excess or extraneous comments can actually make your code harder to read. Make sure you comment any places where the logic is not clear to anyone new to the code (or even to you 6 months later.). You can place comments above logic branch points for clarity, and additional comments to the right of lines that need explanation, such as:
//USER HASN'T ENTERED VALUE
if (x==0) then {
elem.style.backgroundColor = cBackMain;
//USER HAS PICKED SECONDARY COLOR
} else if (x==1) {
elem.style.backgroundColor = cBackAlternate;
}
var rx=regex(/^\d{5}/); //match 5 digits at start of line
But please don't add comments that don't add anything if the code is self-explanatory, such as:
lastIndex = 5; //set last index to 5 UNNECESSARY!
Comments indicating ToDo items
Something else I have found very useful when coding a large project, particularly if there are other programmers involved, is to use your comments to communicate to the other programmers or to make notes to yourself. I include comments with my initials anywhere I have a question or know that I need to go back and fix something. I have also included the initials of another programmer in a section where I know they need to do something. Just keep your tag (i.e. your initials) consistent so you can easily do a global search to find all the comments. Be sure to comment any temporary or debugging code so you can make sure it is removed before final. Some IDE environments automatically support and track TODO type comments for similar usage. I might have comments like:
//RCE: this function needs to be optimized
//RCE: I am not sure what the possible values are here
//DD: Can you add the appropriate code in here?
//RCE: temporary test - take out before final
Comments for documentation such as JSDoc
Documenting your code always seems to be a real pain and something you put off until the very end, or sometimes after the end, of the project. Depending on your project, this can be a real detriment. If items are not documented right away, you may have trouble remembering exactly how functions work or why they were written. If you are writing modules that will be used by other programmers, or have a published API, then proper documentation is crucial.
There are a number of products that simplify your JavaScript code documentation task, such as JSDoc or YUIDoc. These rely on the programmer embedding comments using a format specific to the tool, such as:
/**
* Method description about the following method. Can be several lines.
*
* @method nameOfMethod
* @param {String} objname Description of this string.
* @param {Object} obj Description of object that is passed in
* @return {Boolean} Returns true on success
*/
Whether you want to use one of these methods is up to you and your project team. Most likely the person responsible for producing the formal documentation for the project will have the final say. The advantages of using one of these methods include:
They allow the programmer to communicate complicated information to the documentation team.
They force the programmer to properly document their methods, often resulting in better and more concise API's.
Some IDE's take advantage of embedded documentation to give you popup help, variable typing information, or code completion.
If done concurrently with your code, then you will have your documentation ready when your code is done.
However, there are some disadvantages to this approach. Your team (or yourself if working alone) will have to decide on the correct approach. Some disadvantages include:
It takes discipline on each programmer's part to properly fill out each comment section.
You have to be careful that comment sections aren't just copied and pasted without the proper changes being made.
They can make the actual code a lot harder to read. Large sections of comments can make it harder to make sense of your code. Some IDE's make it easy to hide comment sections.
If several programmers are working on the same project, they may have different documentation styles that the documentation team will have to reconcile before producing the final formal documentation.
Programmers tend to give comments a lower priority than the actual code. The project lead or documentation team will have to spend time to ensure that the comments are properly added and updated.
(Continues...)
Excerpted from JavaScript: Optimizing Native JavaScript by Robert C. Etheredge. Copyright © 2017 MiraVista Press. Excerpted by permission of MiraVista Press.
All rights reserved. No part of this excerpt may be reproduced or reprinted without permission in writing from the publisher.
Excerpts are provided by Dial-A-Book Inc. solely for the personal use of visitors to this web site.
Table of Contents
Introduction
1 Basic Principles
Principles 9
2 JavaScript Formatting
Strict mode 13
Comments 14
Punctuation 16
Lines and spacing 18
Variable and function names 19
Global namespace 20
The var declaration statement 23
Code compilers and mini tiers 25
3 JavaScript
JavaScript versions 29
Performance 29
Variable types 30
Using the typeof 'command 32
Testing for 'falsey' values 34
Hoisting 37
Converting strings to numbers 38
Converting numbers to strings 40
Loops 42
Concatenating strings 45
Regular expressions 45
Should I switch to using switch? 46
SetTimeout is your friend 47
Reduce the creation of garbage 49
Sparse arrays 52
Handling typeahead 54
Try/Catch 55
Using fixed arrays 56
Dangers of premature optimization 58
Infinite scrolling on non-touch screens 58
4 Objects
Creating objects 61
Declaring methods for objects 63
Deleting unused object references 64
Assigning object properties 66
Getters and setters 67
Using this and that with objects 68
Enumerating properties of an object 69
5 HTML
How to add HTML elements to your application 71
Getting to your first screen faster 72
Ways to minimize your impact on the DOM 73
Difference between visibility, opacity, and display 74
Finding HTML elements 75
Binding events 77
InnerText vs. innerHTML 78
Getting images to display faster 80
6 CSS Techniques
Including CSS in your application 83
Position property 83
Display property 84
Setting style properties using JavaScript 84
CSS selectors 86
CSS box model 89
Making text selectable 90
Centering elements 91
Other CSS properties I often forget 92
7 Event Handling
Setting event handlers 93
Event propagation 94
Event delegation using event bubbling 97
Setting handlers for mousemove events 98
Removing event handlers 98
Handling dblClick events 99
Getting the event properties you want 99
8 Handling Data Efficiently
Managing server requests 103
Managing memory and bandwidth 104
Managing the DOM 105
Improving performance 106
Real life examples 107
Scrolling, sorting, and filtering large lists 110
Caching 112
9 Fetching Server Data
Using GET or POST requests 115
Cross-domain problems 116
Differences between UNIX and Windows 116
Using canned data 117
Validating your JSON files 118
Caching your server requests 119
10 Localization
Date format 121
Using icons and colors 123
Date and time formatting functions 123
Language localization 127
11 Loading program files
Using a file loader 130
12 Tools
Frameworks 134
Libraries 134
Templates 135
CSS Preprocessors 136
Polyfills and shims 136
Minification tools 136
Script loaders 137
13 Debugging
Chrome developer tools 139
Debugging using console.log 146
Common errors with closures 147
Proper error handling 148
A Interviewing
How to automatically be in the top 50% of candidates 153
Answering technical questions 155
General types of questions at interviews 156
Coding questions 157
Logic/Brainteaser questions 159
Example questions 161
B UI Design
Single page apps 163
General UI goals 163
Models and metaphors 166
Function first-form later 168
Screen size 171
Index 175