Machine Learning with TensorFlow
Summary
Machine Learning with TensorFlow gives readers a solid foundation in machine-learning concepts plus hands-on experience coding TensorFlow with Python.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
TensorFlow, Google's library for large-scale machine learning, simplifies often-complex computations by representing them as graphs and efficiently mapping parts of the graphs to machines in a cluster or to the processors of a single machine.
About the Book
Machine Learning with TensorFlow gives readers a solid foundation in machine-learning concepts plus hands-on experience coding TensorFlow with Python. You'll learn the basics by working with classic prediction, classification, and clustering algorithms. Then, you'll move on to the money chapters: exploration of deep-learning concepts like autoencoders, recurrent neural networks, and reinforcement learning. Digest this book and you will be ready to use TensorFlow for machine-learning and deep-learning applications of your own.
What's Inside
About the Reader
Written for developers experienced with Python and algebraic concepts like vectors and matrices.
About the Author
Author Nishant Shukla is a computer vision researcher focused on applying machine-learning techniques in robotics.
Senior technical editor, Kenneth Fricklas, is a seasoned developer, author, and machine-learning practitioner.
Table of Contents
1124196866
Machine Learning with TensorFlow gives readers a solid foundation in machine-learning concepts plus hands-on experience coding TensorFlow with Python.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
TensorFlow, Google's library for large-scale machine learning, simplifies often-complex computations by representing them as graphs and efficiently mapping parts of the graphs to machines in a cluster or to the processors of a single machine.
About the Book
Machine Learning with TensorFlow gives readers a solid foundation in machine-learning concepts plus hands-on experience coding TensorFlow with Python. You'll learn the basics by working with classic prediction, classification, and clustering algorithms. Then, you'll move on to the money chapters: exploration of deep-learning concepts like autoencoders, recurrent neural networks, and reinforcement learning. Digest this book and you will be ready to use TensorFlow for machine-learning and deep-learning applications of your own.
What's Inside
- Matching your tasks to the right machine-learning and deep-learning approaches
- Visualizing algorithms with TensorBoard
- Understanding and using neural networks
About the Reader
Written for developers experienced with Python and algebraic concepts like vectors and matrices.
About the Author
Author Nishant Shukla is a computer vision researcher focused on applying machine-learning techniques in robotics.
Senior technical editor, Kenneth Fricklas, is a seasoned developer, author, and machine-learning practitioner.
Table of Contents
- PART 1 - YOUR MACHINE-LEARNING RIG
- A machine-learning odyssey
- TensorFlow essentials PART 2 - CORE LEARNING ALGORITHMS
- Linear regression and beyond
- A gentle introduction to classification
- Automatically clustering data
- Hidden Markov models PART 3 - THE NEURAL NETWORK PARADIGM
- A peek into autoencoders
- Reinforcement learning
- Convolutional neural networks
- Recurrent neural networks
- Sequence-to-sequence models for chatbots
- Utility landscape
Machine Learning with TensorFlow
Summary
Machine Learning with TensorFlow gives readers a solid foundation in machine-learning concepts plus hands-on experience coding TensorFlow with Python.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
TensorFlow, Google's library for large-scale machine learning, simplifies often-complex computations by representing them as graphs and efficiently mapping parts of the graphs to machines in a cluster or to the processors of a single machine.
About the Book
Machine Learning with TensorFlow gives readers a solid foundation in machine-learning concepts plus hands-on experience coding TensorFlow with Python. You'll learn the basics by working with classic prediction, classification, and clustering algorithms. Then, you'll move on to the money chapters: exploration of deep-learning concepts like autoencoders, recurrent neural networks, and reinforcement learning. Digest this book and you will be ready to use TensorFlow for machine-learning and deep-learning applications of your own.
What's Inside
About the Reader
Written for developers experienced with Python and algebraic concepts like vectors and matrices.
About the Author
Author Nishant Shukla is a computer vision researcher focused on applying machine-learning techniques in robotics.
Senior technical editor, Kenneth Fricklas, is a seasoned developer, author, and machine-learning practitioner.
Table of Contents
Machine Learning with TensorFlow gives readers a solid foundation in machine-learning concepts plus hands-on experience coding TensorFlow with Python.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
TensorFlow, Google's library for large-scale machine learning, simplifies often-complex computations by representing them as graphs and efficiently mapping parts of the graphs to machines in a cluster or to the processors of a single machine.
About the Book
Machine Learning with TensorFlow gives readers a solid foundation in machine-learning concepts plus hands-on experience coding TensorFlow with Python. You'll learn the basics by working with classic prediction, classification, and clustering algorithms. Then, you'll move on to the money chapters: exploration of deep-learning concepts like autoencoders, recurrent neural networks, and reinforcement learning. Digest this book and you will be ready to use TensorFlow for machine-learning and deep-learning applications of your own.
What's Inside
- Matching your tasks to the right machine-learning and deep-learning approaches
- Visualizing algorithms with TensorBoard
- Understanding and using neural networks
About the Reader
Written for developers experienced with Python and algebraic concepts like vectors and matrices.
About the Author
Author Nishant Shukla is a computer vision researcher focused on applying machine-learning techniques in robotics.
Senior technical editor, Kenneth Fricklas, is a seasoned developer, author, and machine-learning practitioner.
Table of Contents
- PART 1 - YOUR MACHINE-LEARNING RIG
- A machine-learning odyssey
- TensorFlow essentials PART 2 - CORE LEARNING ALGORITHMS
- Linear regression and beyond
- A gentle introduction to classification
- Automatically clustering data
- Hidden Markov models PART 3 - THE NEURAL NETWORK PARADIGM
- A peek into autoencoders
- Reinforcement learning
- Convolutional neural networks
- Recurrent neural networks
- Sequence-to-sequence models for chatbots
- Utility landscape
44.99
Out Of Stock
5
1
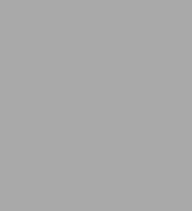
Machine Learning with TensorFlow
272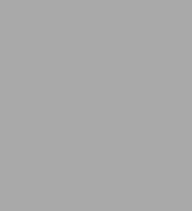
Machine Learning with TensorFlow
272
44.99
Out Of Stock
From the B&N Reads Blog