Kotlin is a highly concise, elegant, fluent, and expressive statically typed multi-paradigm language. It is one of the few languages that compiles down to both Java bytecode and JavaScript. You can use it to build server-side, front-end, and Android applications. With Kotlin, you need less code to accomplish your tasks, while keeping the code type-safe and less prone to error. If you want to learn the essentials of Kotlin, from the fundamentals to more advanced concepts, you've picked the right book.
Fire up your favorite IDE and practice hundreds of examples and exercises to sharpen your Kotlin skills. Learn to build standalone small programs to run as scripts, create type safe code, and then carry that knowledge forward to create fully object-oriented and functional style code that's easier to extend. Learn how to program with elegance but without compromising efficiency or performance, and how to use metaprogramming to build highly expressive code and create internal DSLs that exploit the fluency of the language. Explore coroutines, program asynchrony, run automated tests, and intermix Kotlin with Java in your enterprise applications.
This book will help you master one of the few languages that you can use for the entire full stack - from the server to mobile devices - to create performant, concise, and easy to maintain applications.
What You Need:
To try out the examples in the book you'll need a computer with Kotlin SDK, JDK, and a text editor or a Kotlin IDE installed in it.
Kotlin is a highly concise, elegant, fluent, and expressive statically typed multi-paradigm language. It is one of the few languages that compiles down to both Java bytecode and JavaScript. You can use it to build server-side, front-end, and Android applications. With Kotlin, you need less code to accomplish your tasks, while keeping the code type-safe and less prone to error. If you want to learn the essentials of Kotlin, from the fundamentals to more advanced concepts, you've picked the right book.
Fire up your favorite IDE and practice hundreds of examples and exercises to sharpen your Kotlin skills. Learn to build standalone small programs to run as scripts, create type safe code, and then carry that knowledge forward to create fully object-oriented and functional style code that's easier to extend. Learn how to program with elegance but without compromising efficiency or performance, and how to use metaprogramming to build highly expressive code and create internal DSLs that exploit the fluency of the language. Explore coroutines, program asynchrony, run automated tests, and intermix Kotlin with Java in your enterprise applications.
This book will help you master one of the few languages that you can use for the entire full stack - from the server to mobile devices - to create performant, concise, and easy to maintain applications.
What You Need:
To try out the examples in the book you'll need a computer with Kotlin SDK, JDK, and a text editor or a Kotlin IDE installed in it.
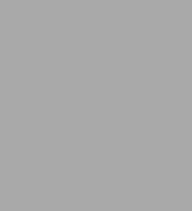
Programming Kotlin: Create Elegant, Expressive, and Performant JVM and Android Applications
462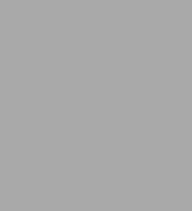
Programming Kotlin: Create Elegant, Expressive, and Performant JVM and Android Applications
462Paperback
Product Details
ISBN-13: | 9781680506358 |
---|---|
Publisher: | Pragmatic Bookshelf |
Publication date: | 10/08/2019 |
Pages: | 462 |
Product dimensions: | 7.40(w) x 9.10(h) x 1.00(d) |