5
1
9780672329784
Python Essential Reference / Edition 4 available in Paperback, eBook
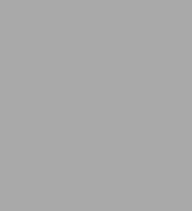
Python Essential Reference / Edition 4
- ISBN-10:
- 0672329786
- ISBN-13:
- 9780672329784
- Pub. Date:
- 07/09/2009
- Publisher:
- Pearson Education
- ISBN-10:
- 0672329786
- ISBN-13:
- 9780672329784
- Pub. Date:
- 07/09/2009
- Publisher:
- Pearson Education
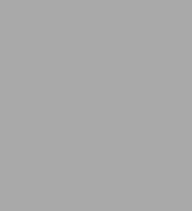
Python Essential Reference / Edition 4
$49.99
Current price is , Original price is $49.99. You
49.99
In Stock
Product Details
ISBN-13: | 9780672329784 |
---|---|
Publisher: | Pearson Education |
Publication date: | 07/09/2009 |
Series: | Developer's Library |
Edition description: | Fourth |
Pages: | 752 |
Sales rank: | 983,180 |
Product dimensions: | 5.90(w) x 8.90(h) x 1.10(d) |
About the Author
From the B&N Reads Blog