Rust in Action
Rust in Action is a hands-on guide to systems programming with Rust. Written for inquisitive programmers, it presents real-world use cases that go far beyond syntax and structure.
Summary
Rust in Action introduces the Rust programming language by exploring numerous systems programming concepts and techniques. You'll be learning Rust by delving into how computers work under the hood. You'll find yourself playing with persistent storage, memory, networking and even tinkering with CPU instructions. The book takes you through using Rust to extend other applications and teaches you tricks to write blindingly fast code. You'll also discover parallel and concurrent programming. Filled to the brim with real-life use cases and scenarios, you'll go beyond the Rust syntax and see what Rust has to offer in real-world use cases.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Rust is the perfect language for systems programming. It delivers the low-level power of C along with rock-solid safety features that let you code fearlessly. Ideal for applications requiring concurrency, Rust programs are compact, readable, and blazingly fast. Best of all, Rust’s famously smart compiler helps you avoid even subtle coding errors.
About the book
Rust in Action is a hands-on guide to systems programming with Rust. Written for inquisitive programmers, it presents real-world use cases that go far beyond syntax and structure. You’ll explore Rust implementations for file manipulation, networking, and kernel-level programming and discover awesome techniques for parallelism and concurrency. Along the way, you’ll master Rust’s unique borrow checker model for memory management without a garbage collector.
What's inside
Elementary to advanced Rust programming
Practical examples from systems programming
Command-line, graphical and networked applications
About the reader
For intermediate programmers. No previous experience with Rust required.
About the author
Tim McNamara uses Rust to build data processing pipelines and generative art. He is an expert in natural language processing and data engineering.
Table of Contents
1 Introducing Rust
PART 1 RUST LANGUAGE DISTINCTIVES
2 Language foundations
3 Compound data types
4 Lifetimes, ownership, and borrowing
PART 2 DEMYSTIFYING SYSTEMS PROGRAMMING
5 Data in depth
6 Memory
7 Files and storage
8 Networking
9 Time and timekeeping
10 Processes, threads, and containers
11 Kernel
12 Signals, interrupts, and exceptions
1134424547
Summary
Rust in Action introduces the Rust programming language by exploring numerous systems programming concepts and techniques. You'll be learning Rust by delving into how computers work under the hood. You'll find yourself playing with persistent storage, memory, networking and even tinkering with CPU instructions. The book takes you through using Rust to extend other applications and teaches you tricks to write blindingly fast code. You'll also discover parallel and concurrent programming. Filled to the brim with real-life use cases and scenarios, you'll go beyond the Rust syntax and see what Rust has to offer in real-world use cases.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Rust is the perfect language for systems programming. It delivers the low-level power of C along with rock-solid safety features that let you code fearlessly. Ideal for applications requiring concurrency, Rust programs are compact, readable, and blazingly fast. Best of all, Rust’s famously smart compiler helps you avoid even subtle coding errors.
About the book
Rust in Action is a hands-on guide to systems programming with Rust. Written for inquisitive programmers, it presents real-world use cases that go far beyond syntax and structure. You’ll explore Rust implementations for file manipulation, networking, and kernel-level programming and discover awesome techniques for parallelism and concurrency. Along the way, you’ll master Rust’s unique borrow checker model for memory management without a garbage collector.
What's inside
Elementary to advanced Rust programming
Practical examples from systems programming
Command-line, graphical and networked applications
About the reader
For intermediate programmers. No previous experience with Rust required.
About the author
Tim McNamara uses Rust to build data processing pipelines and generative art. He is an expert in natural language processing and data engineering.
Table of Contents
1 Introducing Rust
PART 1 RUST LANGUAGE DISTINCTIVES
2 Language foundations
3 Compound data types
4 Lifetimes, ownership, and borrowing
PART 2 DEMYSTIFYING SYSTEMS PROGRAMMING
5 Data in depth
6 Memory
7 Files and storage
8 Networking
9 Time and timekeeping
10 Processes, threads, and containers
11 Kernel
12 Signals, interrupts, and exceptions
Rust in Action
Rust in Action is a hands-on guide to systems programming with Rust. Written for inquisitive programmers, it presents real-world use cases that go far beyond syntax and structure.
Summary
Rust in Action introduces the Rust programming language by exploring numerous systems programming concepts and techniques. You'll be learning Rust by delving into how computers work under the hood. You'll find yourself playing with persistent storage, memory, networking and even tinkering with CPU instructions. The book takes you through using Rust to extend other applications and teaches you tricks to write blindingly fast code. You'll also discover parallel and concurrent programming. Filled to the brim with real-life use cases and scenarios, you'll go beyond the Rust syntax and see what Rust has to offer in real-world use cases.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Rust is the perfect language for systems programming. It delivers the low-level power of C along with rock-solid safety features that let you code fearlessly. Ideal for applications requiring concurrency, Rust programs are compact, readable, and blazingly fast. Best of all, Rust’s famously smart compiler helps you avoid even subtle coding errors.
About the book
Rust in Action is a hands-on guide to systems programming with Rust. Written for inquisitive programmers, it presents real-world use cases that go far beyond syntax and structure. You’ll explore Rust implementations for file manipulation, networking, and kernel-level programming and discover awesome techniques for parallelism and concurrency. Along the way, you’ll master Rust’s unique borrow checker model for memory management without a garbage collector.
What's inside
Elementary to advanced Rust programming
Practical examples from systems programming
Command-line, graphical and networked applications
About the reader
For intermediate programmers. No previous experience with Rust required.
About the author
Tim McNamara uses Rust to build data processing pipelines and generative art. He is an expert in natural language processing and data engineering.
Table of Contents
1 Introducing Rust
PART 1 RUST LANGUAGE DISTINCTIVES
2 Language foundations
3 Compound data types
4 Lifetimes, ownership, and borrowing
PART 2 DEMYSTIFYING SYSTEMS PROGRAMMING
5 Data in depth
6 Memory
7 Files and storage
8 Networking
9 Time and timekeeping
10 Processes, threads, and containers
11 Kernel
12 Signals, interrupts, and exceptions
Summary
Rust in Action introduces the Rust programming language by exploring numerous systems programming concepts and techniques. You'll be learning Rust by delving into how computers work under the hood. You'll find yourself playing with persistent storage, memory, networking and even tinkering with CPU instructions. The book takes you through using Rust to extend other applications and teaches you tricks to write blindingly fast code. You'll also discover parallel and concurrent programming. Filled to the brim with real-life use cases and scenarios, you'll go beyond the Rust syntax and see what Rust has to offer in real-world use cases.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Rust is the perfect language for systems programming. It delivers the low-level power of C along with rock-solid safety features that let you code fearlessly. Ideal for applications requiring concurrency, Rust programs are compact, readable, and blazingly fast. Best of all, Rust’s famously smart compiler helps you avoid even subtle coding errors.
About the book
Rust in Action is a hands-on guide to systems programming with Rust. Written for inquisitive programmers, it presents real-world use cases that go far beyond syntax and structure. You’ll explore Rust implementations for file manipulation, networking, and kernel-level programming and discover awesome techniques for parallelism and concurrency. Along the way, you’ll master Rust’s unique borrow checker model for memory management without a garbage collector.
What's inside
Elementary to advanced Rust programming
Practical examples from systems programming
Command-line, graphical and networked applications
About the reader
For intermediate programmers. No previous experience with Rust required.
About the author
Tim McNamara uses Rust to build data processing pipelines and generative art. He is an expert in natural language processing and data engineering.
Table of Contents
1 Introducing Rust
PART 1 RUST LANGUAGE DISTINCTIVES
2 Language foundations
3 Compound data types
4 Lifetimes, ownership, and borrowing
PART 2 DEMYSTIFYING SYSTEMS PROGRAMMING
5 Data in depth
6 Memory
7 Files and storage
8 Networking
9 Time and timekeeping
10 Processes, threads, and containers
11 Kernel
12 Signals, interrupts, and exceptions
59.99
In Stock
5
1
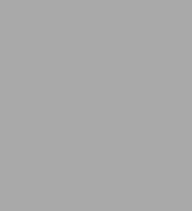
Rust in Action
456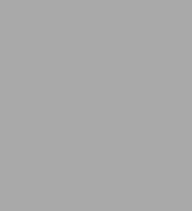
Rust in Action
456
59.99
In Stock
From the B&N Reads Blog