In this book's straightforward, step-by-step approach, each lesson builds on everything that's come before, helping readers learn Java's core features and techniques from the ground up. Friendly, accessible, and conversational, this book offers a practical grounding in the language, without ever becoming overwhelming or intimidating.
Week 1 introduces the basic building blocks of the Java programming language: keywords, operators, class and object definitions, packages, interfaces, exceptions, and threads.
Week 2 covers the Swing graphical user interface class libraries and the important classes that support data structures, string handling, dates and times.
Week 3 ventures into the hottest areas of Java programming: web services, Java servlets, network programming, database programming and Android development.
In this book's straightforward, step-by-step approach, each lesson builds on everything that's come before, helping readers learn Java's core features and techniques from the ground up. Friendly, accessible, and conversational, this book offers a practical grounding in the language, without ever becoming overwhelming or intimidating.
Week 1 introduces the basic building blocks of the Java programming language: keywords, operators, class and object definitions, packages, interfaces, exceptions, and threads.
Week 2 covers the Swing graphical user interface class libraries and the important classes that support data structures, string handling, dates and times.
Week 3 ventures into the hottest areas of Java programming: web services, Java servlets, network programming, database programming and Android development.
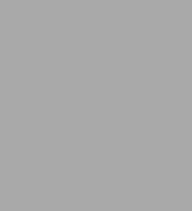
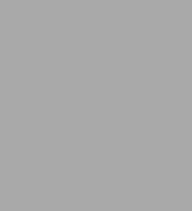
Paperback(8th ed.)
-
SHIP THIS ITEMIn stock. Ships in 1-2 days.PICK UP IN STORE
Your local store may have stock of this item.
Available within 2 business hours
Related collections and offers
Overview
In this book's straightforward, step-by-step approach, each lesson builds on everything that's come before, helping readers learn Java's core features and techniques from the ground up. Friendly, accessible, and conversational, this book offers a practical grounding in the language, without ever becoming overwhelming or intimidating.
Week 1 introduces the basic building blocks of the Java programming language: keywords, operators, class and object definitions, packages, interfaces, exceptions, and threads.
Week 2 covers the Swing graphical user interface class libraries and the important classes that support data structures, string handling, dates and times.
Week 3 ventures into the hottest areas of Java programming: web services, Java servlets, network programming, database programming and Android development.
Product Details
ISBN-13: | 9780672337956 |
---|---|
Publisher: | Pearson Education |
Publication date: | 01/15/2020 |
Series: | Sams Teach Yourself |
Edition description: | 8th ed. |
Pages: | 672 |
Product dimensions: | 7.00(w) x 9.10(h) x 1.50(d) |
About the Author
Table of Contents
Introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1How This Book Is Organized ....................................................................................................2
Who Should Read This Book ...................................................................................................4
Conventions Used in This Book ...............................................................................................5
PART I The Java Language . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .7
LESSON 1: Getting Started with Java 9
The Java Language ..................................................................................................................10
Object-Oriented Programming ................................................................................................13
Objects and Classes .................................................................................................................14
Attributes and Behavior ..........................................................................................................16
Organizing Classes and Class Behavior ..................................................................................25
Summary .................................................................................................................................32
Q&A ........................................................................................................................................33
Quiz .........................................................................................................................................34
Certification Practice ...............................................................................................................35
Exercises ..................................................................................................................................35
LESSON 2: The ABCs of Programming 37
Statements and Expressions ....................................................................................................38
Variables and Data Types ........................................................................................................38
Comments................................................................................................................................46
Literals .....................................................................................................................................47
String Literals ...............................................................................................................50
Expressions and Operators ......................................................................................................51
String Arithmetic .....................................................................................................................60
Summary .................................................................................................................................62
Q&A ........................................................................................................................................62
Quiz .........................................................................................................................................63
Certification Practice ...............................................................................................................64
Exercises ..................................................................................................................................65
LESSON 3: Working with Objects 67
Creating New Objects .............................................................................................................68
Using Class and Instance Variables ........................................................................................72
Calling Methods ......................................................................................................................75
Class Methods ..............................................................................................................79
References to Objects ..............................................................................................................80
Casting Objects and Primitive Types ......................................................................................82
Converting Primitive Types to Objects and Vice Versa ................................................85
Comparing Object Values and Classes ....................................................................................87
Summary .................................................................................................................................89
Q&A ........................................................................................................................................90
Quiz .........................................................................................................................................91
Certification Practice ...............................................................................................................91
Exercises ..................................................................................................................................92
LESSON 4: Lists, Logic, and Loops 93
Arrays ......................................................................................................................................94
Block Statements ...................................................................................................................101
if Conditionals .....................................................................................................................102
Switch Conditionals ..............................................................................................................103
The Ternary Operator ............................................................................................................110
for Loops ..............................................................................................................................111
while and do Loops ..............................................................................................................114
xii Sams Teach Yourself Java in 21 Days
Breaking Out of Loops ..........................................................................................................117
Summary ...............................................................................................................................119
Q&A ......................................................................................................................................119
Quiz .......................................................................................................................................120
Certification Practice .............................................................................................................121
Exercises ................................................................................................................................122
LESSON 5: Creating Classes and Methods 123
Defining Classes ....................................................................................................................124
Creating Instance and Class Variables ..................................................................................124
Creating Methods ..................................................................................................................126
Creating Java Applications ....................................................................................................133
Java Applications and Arguments .........................................................................................134
Creating Methods with the Same Name ...............................................................................137
Constructors ..........................................................................................................................142
Overriding Methods ..............................................................................................................146
Summary ...............................................................................................................................150
Q&A ......................................................................................................................................151
Quiz .......................................................................................................................................152
Certification Practice .............................................................................................................153
Exercises ................................................................................................................................154
LESSON 6: Packages, Interfaces, and Other Class Features 155
Modifiers ...............................................................................................................................156
Static Variables and Methods ................................................................................................162
Final Classes, Methods, and Variables ..................................................................................165
Abstract Classes and Methods ..............................................................................................167
Packages ................................................................................................................................167
Creating Your Own Packages ................................................................................................171
Interfaces ...............................................................................................................................174
Creating and Extending Interfaces ........................................................................................177
Summary ...............................................................................................................................185
Q&A ......................................................................................................................................186
Quiz .......................................................................................................................................186
Certification Practice .............................................................................................................187
Exercises ................................................................................................................................188
LESSON 7: Exceptions and Threads 189
Exceptions .............................................................................................................................190
Managing Exceptions ............................................................................................................193
Declaring Methods That Might Throw Exceptions ..............................................................200
Creating and Throwing Exceptions .......................................................................................205
When Not to Use Exceptions ................................................................................................208
Threads ..................................................................................................................................209
Summary ...............................................................................................................................216
Q&A ......................................................................................................................................217
Quiz .......................................................................................................................................217
Certification Practice .............................................................................................................218
Exercises ................................................................................................................................219
PART II: The Java Class Library . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .221
LESSON 8: Data Structures 223
Moving Beyond Arrays .........................................................................................................224
Java Structures .......................................................................................................................224
Generics .................................................................................................................................244
Enumerations .........................................................................................................................247
Summary ...............................................................................................................................249
Q&A ......................................................................................................................................249
Quiz .......................................................................................................................................250
Certification Practice .............................................................................................................251
Exercises ................................................................................................................................252
LESSON 9: Creating a Graphical User Interface 253
Creating an Application ........................................................................................................254
Working with Components ...................................................................................................261
Lists .......................................................................................................................................274
The Java Class Library ..........................................................................................................276
Summary ...............................................................................................................................278
Q&A ......................................................................................................................................279
Quiz .......................................................................................................................................279
Certification Practice .............................................................................................................280
Exercises ................................................................................................................................281
LESSON 10: Building an Interface 283
Swing Features ......................................................................................................................284
Summary ...............................................................................................................................304
Q&A ......................................................................................................................................304
Quiz .......................................................................................................................................305
Certification Practice .............................................................................................................306
Exercises ................................................................................................................................307
LESSON 11: Arranging Components on a User Interface 309
Basic Interface Layout ..........................................................................................................310
Mixing Layout Managers ......................................................................................................320
Card Layout ...........................................................................................................................321
Summary ...............................................................................................................................329
Q&A ......................................................................................................................................330
Quiz .......................................................................................................................................331
Certification Practice .............................................................................................................331
Exercises ................................................................................................................................332
LESSON 12: Responding to User Input 333
Event Listeners ......................................................................................................................334
Working with Methods ..........................................................................................................339
Using Inner Classes ....................................................................................................353
Summary ...............................................................................................................................355
Q&A ......................................................................................................................................355
Quiz .......................................................................................................................................356
Certification Practice .............................................................................................................357
Exercises ................................................................................................................................358
LESSON 13: Creating Java2D Graphics 359
The Graphics2D Class ..........................................................................................................360
Drawing Text .........................................................................................................................362
Color ......................................................................................................................................366
Drawing Lines and Polygons ................................................................................................369
Summary ...............................................................................................................................378
Q&A ......................................................................................................................................379
Quiz .......................................................................................................................................379
Certification Practice .............................................................................................................380
Exercises ................................................................................................................................381
LESSON 14: Developing Swing Applications 383
Improving Performance with SwingWorker .........................................................................384
Grid Bag Layout ....................................................................................................................389
Summary ...............................................................................................................................398
Q&A ......................................................................................................................................398
Quiz .......................................................................................................................................398
Certification Practice .............................................................................................................399
Exercises ................................................................................................................................400
PART III: Java Programming . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .401
LESSON 15: Using Inner Classes and Lambda Expressions 403
Inner Classes .........................................................................................................................404
Lambda Expressions .............................................................................................................413
Variable Type Inference ........................................................................................................418
Summary ...............................................................................................................................419
Q&A ......................................................................................................................................420
Quiz .......................................................................................................................................420
Certification Practice .............................................................................................................421
Exercises ................................................................................................................................422
LESSON 16: Working with Input and Output 423
Introduction to Streams .........................................................................................................424
Byte Streams .........................................................................................................................426
Filtering a Stream ..................................................................................................................431
Character Streams .................................................................................................................440
Files and Paths.......................................................................................................................445
Summary ...............................................................................................................................447
Q&A ......................................................................................................................................448
Quiz .......................................................................................................................................448
Certification Practice .............................................................................................................449
Exercises ................................................................................................................................450
LESSON 17: Communicating Over HTTP 451
Networking in Java ................................................................................................................452
The java.nio Package .........................................................................................................465
Summary ...............................................................................................................................480
Q&A ......................................................................................................................................480
Quiz .......................................................................................................................................481
Certification Practice .............................................................................................................482
Exercises ................................................................................................................................482
LESSON 18: Accessing Databases with JDBC and Derby 483
Java Database Connectivity...................................................................................................484
Summary ...............................................................................................................................501
Q&A ......................................................................................................................................502
Quiz .......................................................................................................................................502
Certification Practice .............................................................................................................503
Exercises ................................................................................................................................503
LESSON 19: Reading and Writing RSS Feeds 505
Using XML ...........................................................................................................................506
Designing an XML Dialect ...................................................................................................508
Processing XML with Java ...................................................................................................510
Processing XML with XOM .................................................................................................510
Summary ...............................................................................................................................525
Q&A ......................................................................................................................................525
Quiz .......................................................................................................................................526
Certification Practice .............................................................................................................527
Exercises ................................................................................................................................528
LESSON 20: Making Web Service Requests 529
Introduction to XML-RPC ....................................................................................................530
Communicating with XML-RPC ..........................................................................................531
Choosing an XML-RPC Implementation .............................................................................534
Using an XML-RPC Web Service ........................................................................................536
Creating an XML-RPC Web Service ....................................................................................539
Summary ...............................................................................................................................545
Q&A ......................................................................................................................................545
Quiz .......................................................................................................................................546
Certification Practice .............................................................................................................546
Exercises ................................................................................................................................547
LESSON 21: Writing a Game with Java 549
Playing a Game .....................................................................................................................550
Summary ...............................................................................................................................572
Q&A ......................................................................................................................................572
Quiz .......................................................................................................................................573
Certification Practice .............................................................................................................574
Exercises ................................................................................................................................575
APPENDIXES . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 597
APPENDIX A: Using the NetBeans Integrated Development Environment . . . . . . 599
APPENDIX B: Fixing Package Not Visible Errors in NetBeans . . . . . . . . . . . . . . . . 609
APPENDIX C: This Book’s Website. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 613
APPENDIX D: Using the Java Development Kit . . . . . . . . . . . . . . . . . . . . . . . . . . . 615
APPENDIX E: Programming with the Java Development Kit . . . . . . . . . . . . . . . . . 631
9780672337956, TOC, 11/18/2019