Spring Security in Action
Spring Security in Action shows you how to prevent cross-site scripting and request forgery attacks before they do damage. You’ll start with the basics, simulating password upgrades and adding multiple types of authorization. As your skills grow, you'll adapt Spring Security to new architectures and create advanced OAuth2 configurations. By the time you're done, you'll have a customized Spring Security configuration that protects against threats both common and extraordinary.
Summary
While creating secure applications is critically important, it can also be tedious and time-consuming to stitch together the required collection of tools. For Java developers, the powerful Spring Security framework makes it easy for you to bake security into your software from the very beginning. Filled with code samples and practical examples, Spring Security in Action teaches you how to secure your apps from the most common threats, ranging from injection attacks to lackluster monitoring. In it, you'll learn how to manage system users, configure secure endpoints, and use OAuth2 and OpenID Connect for authentication and authorization.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Security is non-negotiable. You rely on Spring applications to transmit data, verify credentials, and prevent attacks. Adopting "secure by design" principles will protect your network from data theft and unauthorized intrusions.
About the book
Spring Security in Action shows you how to prevent cross-site scripting and request forgery attacks before they do damage. You’ll start with the basics, simulating password upgrades and adding multiple types of authorization. As your skills grow, you'll adapt Spring Security to new architectures and create advanced OAuth2 configurations. By the time you're done, you'll have a customized Spring Security configuration that protects against threats both common and extraordinary.
What's inside
Encoding passwords and authenticating users
Securing endpoints
Automating security testing
Setting up a standalone authorization server
About the reader
For experienced Java and Spring developers.
About the author
Laurentiu Spilca is a dedicated development lead and trainer at Endava, with over ten years of Java experience.
Table of Contents
PART 1 - FIRST STEPS
1 Security Today
2 Hello Spring Security
PART 2 - IMPLEMENTATION
3 Managing users
4 Dealing with passwords
5 Implementing authentication
6 Hands-on: A small secured web application
7 Configuring authorization: Restricting access
8 Configuring authorization: Applying restrictions
9 Implementing filters
10 Applying CSRF protection and CORS
11 Hands-on: A separation of responsibilities
12 How does OAuth 2 work?
13 OAuth 2: Implementing the authorization server
14 OAuth 2: Implementing the resource server
15 OAuth 2: Using JWT and cryptographic signatures
16 Global method security: Pre- and postauthorizations
17 Global method security: Pre- and postfiltering
18 Hands-on: An OAuth 2 application
19 Spring Security for reactive apps
20 Spring Security testing
1136806455
Summary
While creating secure applications is critically important, it can also be tedious and time-consuming to stitch together the required collection of tools. For Java developers, the powerful Spring Security framework makes it easy for you to bake security into your software from the very beginning. Filled with code samples and practical examples, Spring Security in Action teaches you how to secure your apps from the most common threats, ranging from injection attacks to lackluster monitoring. In it, you'll learn how to manage system users, configure secure endpoints, and use OAuth2 and OpenID Connect for authentication and authorization.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Security is non-negotiable. You rely on Spring applications to transmit data, verify credentials, and prevent attacks. Adopting "secure by design" principles will protect your network from data theft and unauthorized intrusions.
About the book
Spring Security in Action shows you how to prevent cross-site scripting and request forgery attacks before they do damage. You’ll start with the basics, simulating password upgrades and adding multiple types of authorization. As your skills grow, you'll adapt Spring Security to new architectures and create advanced OAuth2 configurations. By the time you're done, you'll have a customized Spring Security configuration that protects against threats both common and extraordinary.
What's inside
Encoding passwords and authenticating users
Securing endpoints
Automating security testing
Setting up a standalone authorization server
About the reader
For experienced Java and Spring developers.
About the author
Laurentiu Spilca is a dedicated development lead and trainer at Endava, with over ten years of Java experience.
Table of Contents
PART 1 - FIRST STEPS
1 Security Today
2 Hello Spring Security
PART 2 - IMPLEMENTATION
3 Managing users
4 Dealing with passwords
5 Implementing authentication
6 Hands-on: A small secured web application
7 Configuring authorization: Restricting access
8 Configuring authorization: Applying restrictions
9 Implementing filters
10 Applying CSRF protection and CORS
11 Hands-on: A separation of responsibilities
12 How does OAuth 2 work?
13 OAuth 2: Implementing the authorization server
14 OAuth 2: Implementing the resource server
15 OAuth 2: Using JWT and cryptographic signatures
16 Global method security: Pre- and postauthorizations
17 Global method security: Pre- and postfiltering
18 Hands-on: An OAuth 2 application
19 Spring Security for reactive apps
20 Spring Security testing
Spring Security in Action
Spring Security in Action shows you how to prevent cross-site scripting and request forgery attacks before they do damage. You’ll start with the basics, simulating password upgrades and adding multiple types of authorization. As your skills grow, you'll adapt Spring Security to new architectures and create advanced OAuth2 configurations. By the time you're done, you'll have a customized Spring Security configuration that protects against threats both common and extraordinary.
Summary
While creating secure applications is critically important, it can also be tedious and time-consuming to stitch together the required collection of tools. For Java developers, the powerful Spring Security framework makes it easy for you to bake security into your software from the very beginning. Filled with code samples and practical examples, Spring Security in Action teaches you how to secure your apps from the most common threats, ranging from injection attacks to lackluster monitoring. In it, you'll learn how to manage system users, configure secure endpoints, and use OAuth2 and OpenID Connect for authentication and authorization.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Security is non-negotiable. You rely on Spring applications to transmit data, verify credentials, and prevent attacks. Adopting "secure by design" principles will protect your network from data theft and unauthorized intrusions.
About the book
Spring Security in Action shows you how to prevent cross-site scripting and request forgery attacks before they do damage. You’ll start with the basics, simulating password upgrades and adding multiple types of authorization. As your skills grow, you'll adapt Spring Security to new architectures and create advanced OAuth2 configurations. By the time you're done, you'll have a customized Spring Security configuration that protects against threats both common and extraordinary.
What's inside
Encoding passwords and authenticating users
Securing endpoints
Automating security testing
Setting up a standalone authorization server
About the reader
For experienced Java and Spring developers.
About the author
Laurentiu Spilca is a dedicated development lead and trainer at Endava, with over ten years of Java experience.
Table of Contents
PART 1 - FIRST STEPS
1 Security Today
2 Hello Spring Security
PART 2 - IMPLEMENTATION
3 Managing users
4 Dealing with passwords
5 Implementing authentication
6 Hands-on: A small secured web application
7 Configuring authorization: Restricting access
8 Configuring authorization: Applying restrictions
9 Implementing filters
10 Applying CSRF protection and CORS
11 Hands-on: A separation of responsibilities
12 How does OAuth 2 work?
13 OAuth 2: Implementing the authorization server
14 OAuth 2: Implementing the resource server
15 OAuth 2: Using JWT and cryptographic signatures
16 Global method security: Pre- and postauthorizations
17 Global method security: Pre- and postfiltering
18 Hands-on: An OAuth 2 application
19 Spring Security for reactive apps
20 Spring Security testing
Summary
While creating secure applications is critically important, it can also be tedious and time-consuming to stitch together the required collection of tools. For Java developers, the powerful Spring Security framework makes it easy for you to bake security into your software from the very beginning. Filled with code samples and practical examples, Spring Security in Action teaches you how to secure your apps from the most common threats, ranging from injection attacks to lackluster monitoring. In it, you'll learn how to manage system users, configure secure endpoints, and use OAuth2 and OpenID Connect for authentication and authorization.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Security is non-negotiable. You rely on Spring applications to transmit data, verify credentials, and prevent attacks. Adopting "secure by design" principles will protect your network from data theft and unauthorized intrusions.
About the book
Spring Security in Action shows you how to prevent cross-site scripting and request forgery attacks before they do damage. You’ll start with the basics, simulating password upgrades and adding multiple types of authorization. As your skills grow, you'll adapt Spring Security to new architectures and create advanced OAuth2 configurations. By the time you're done, you'll have a customized Spring Security configuration that protects against threats both common and extraordinary.
What's inside
Encoding passwords and authenticating users
Securing endpoints
Automating security testing
Setting up a standalone authorization server
About the reader
For experienced Java and Spring developers.
About the author
Laurentiu Spilca is a dedicated development lead and trainer at Endava, with over ten years of Java experience.
Table of Contents
PART 1 - FIRST STEPS
1 Security Today
2 Hello Spring Security
PART 2 - IMPLEMENTATION
3 Managing users
4 Dealing with passwords
5 Implementing authentication
6 Hands-on: A small secured web application
7 Configuring authorization: Restricting access
8 Configuring authorization: Applying restrictions
9 Implementing filters
10 Applying CSRF protection and CORS
11 Hands-on: A separation of responsibilities
12 How does OAuth 2 work?
13 OAuth 2: Implementing the authorization server
14 OAuth 2: Implementing the resource server
15 OAuth 2: Using JWT and cryptographic signatures
16 Global method security: Pre- and postauthorizations
17 Global method security: Pre- and postfiltering
18 Hands-on: An OAuth 2 application
19 Spring Security for reactive apps
20 Spring Security testing
59.99
In Stock
5
1
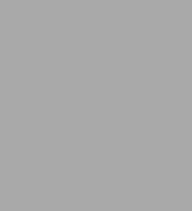
Spring Security in Action
560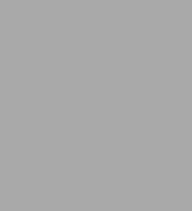
Spring Security in Action
560
59.99
In Stock
From the B&N Reads Blog