- Learn expert programming techniques and strategies to create better applications
- Contains a full section of real-world examples readers can incorporate in their own applications
- CD-ROM contains the complete source code for all of the programs in the book
- Learn expert programming techniques and strategies to create better applications
- Contains a full section of real-world examples readers can incorporate in their own applications
- CD-ROM contains the complete source code for all of the programs in the book
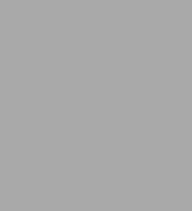
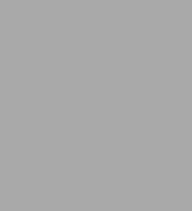
Hardcover(BK&CD-ROM)
-
SHIP THIS ITEMIn stock. Ships in 1-2 days.PICK UP IN STORE
Your local store may have stock of this item.
Available within 2 business hours
Related collections and offers
Overview
- Learn expert programming techniques and strategies to create better applications
- Contains a full section of real-world examples readers can incorporate in their own applications
- CD-ROM contains the complete source code for all of the programs in the book
Product Details
ISBN-13: | 9780672310485 |
---|---|
Publisher: | Sams |
Publication date: | 06/28/1997 |
Edition description: | BK&CD-ROM |
Pages: | 1032 |
Product dimensions: | 7.32(w) x 9.04(h) x 2.00(d) |
Read an Excerpt
[Figures are not included in this sample chapter]
Visual Basic 5 Developers Guide
- 3 -
Classes
Classes enable you to reuse code by creating code components. This chapter shows you how to create these components. A class is the basis for all objects in object-oriented programming. It enables you to create reusable code. Before version 4.0, there was no way to create a class, and therefore Visual Basic was not an object-oriented language--it was considered to be an object-based language. It is still not considered to be a true object-oriented language because even though you can create classes, another programmer cannot truly subclass a class you create. This is referred to as inheritance. It does, however, support polymorphism and encapsulation.
Inheritance is the ability to create a new object or class based on an existing object, which means that you can specify exactly how it is different from the parent object. For example, you not only can inherit the properties from an object, but you also can add other properties. Polymorphism is the concept of objects sharing characteristics from a base class, usually a common interface. This enables a developer to implement the methods and properties of that interface without knowing how the interface works directly. Encapsulation is the ability to wrap up, or encapsulate, different types of data and procedures into a single entity. This is probably the most common type of object-oriented programming.
Classes are useful, in part, because you can create multiple instances of the class. Assigning an object variable to the class is referred to as instantiation, which is the point at which the class is initialized and created. You can assign an object variable to an object in one of two ways. First, you can assign the object with early binding, which means Visual Basic assigns an association to the object as soon as it is declared because it is declared as being of a specific type. For example, assuming you had a class called MyClass, consider these two lines of code for early binding:
Private obj as MyClass Set obj = New MyClass
The first line declares an object variable to be of a specific type. Then, on the second line, the variable is instantiated with a new instance of that class.
The second way to assign an object variable to an object is with late binding, which means Visual Basic does not assign an association to the object until it is used, not just declared. This is because it does not know what type of object to associate it with until it is instantiated. For example, again, assuming you had a class called MyClass, consider these two lines of code for late binding:
Private obj as Object Set obj = New MyClass
The second line will create a reference to a new object called MyClass. Using this syntax, the code component will be reused every time it encounters the New keyword. Every class has any or all of the following associated with it:
- Properties
- Methods
- Events
To view which properties, methods, and events are available within a class, it is useful to use the Object Browser. You invoke the Object Browser by selecting the View | Object Browser menu option or by pressing the F2 key.
One new feature of Visual Basic version 5.0 is the Implements statement. The Implements statement enables you to define one interface and have other classes use (or implement) that interface. Suppose you define the properties, methods, and events in a class module. The procedure of how to do this is discussed throughout this chapter.
Suppose this class is called ClassA. Then, for ClassB to implement the interface defined by ClassA, you would declare ClassB with this line of code:
Implements ClassA
Therefore, the preceding statement implements the interface designed in ClassA.
Creating Classes
You can create classes by selecting the Project | Add Class Module menu option. This brings up a dialog box in which you can choose the types of classes to add. There are three choices:
- Class Module
- VB Class Builder (only Professional and Enterprise editions)
- Add-In
These options make it easy, as with most features in version 5.0, to add classes. Figure 3.1 shows the dialog box that is displayed when you choose to add a class module.
FIGURE 3.1. The Add Class Module dialog box.
Class Module
The Class Module option makes it possible to add your own code manually. Some interfaces are so complex that it might not be advantageous to use a wizard to create the class. Also, some developers like to do everything manually. This makes them feel "in control." To write class modules manually, refer to the section "Coding Classes," later in this chapter.
VB Class Builder
The VB Class Builder is an add-in that makes defining a class's interface easy. This option is available only for the Professional and Enterprise editions of Visual Basic. This add-in can possibly save many hours of your time by prompting you on how the add-in should develop the interface. The VB Class Builder provides tabs and menu options that enable you to view and define your interface. (See Figure 3.2.)
FIGURE 3.2. The VB Class Builder utility.
Suppose you wanted to create an order-taking system. In its simplest form, you would need to create two classes, one for the customers and one for the orders. The next step would be to rename the default Class1 class by clicking on the name and then clicking the right mouse button and choosing the Rename option. Change the name to Customers. Note that the name of a class module must be less than 40 characters.
The Customers class needs to have the following properties with the shown data type:
Property | Data Type |
CustomerID | Integer |
Name | String |
Company | String |
Address1 | String |
Address2 | String |
City | String |
State | String |
Zip | String |
Phone | String |
Fax | String |
String |
To add a new property, select the File | New | Property menu option. Alternatively, you can right-click the appropriate class and choose the New | Property menu option, or, you can click the New Property toolbar button. Either option will enable you to define the new property. By default, the Properties tab is shown (see Figure 3.3).
FIGURE 3.3. The Properties tab of the Property Builder.
You must specify the name of the property. Using this example, type CustomerID, and then choose from the available datatypes. Here are the choices:
Byte
Boolean
Integer
Long
Single
Double
Currency
Date
String
Variant
Object Collection Variant is the default. In our case, choose Integer. Next, you must choose how to declare the property. Here are your choices:
- Public Property--A property that will be exposed by your object to other interfaces that use objects.
- Friend Property--A property that is available for communication within objects but that is not exposed externally to the project.
- Public Variable--A variable that is available throughout the class but that is not exposed externally to the project.
Next, if this property is to serve as the default property, check the Default Property? check box. This means that if, in code, you do not refer to a property, but simply the class, this property will be used.
In this example, select the Public Property option to make the property available to other objects and environments that can access these objects.
The second tab on the Property Builder dialog box is the Attributes tab. It defines additional attributes, as shown in Figure 3.4.
FIGURE 3.4. The Attributes tab of the Property Builder.
These attributes are not functionally necessary. However, they do help to make the class complete. The Description field is used by the type library, which will be generated with the class. The Project Help File field is read-only. It shows the project's Help file, if one is assigned. Finally, in the Help Context ID field you should enter the Help Context ID if you have a topic assigned to the property inside the Help file shown.
Continue adding all the properties shown earlier. When you have added all the properties, the Class Builder main window will look as it does in Figure 3.5.
FIGURE 3.5. The VB Class Builder with all properties added.
In addition to these properties, you will most likely need a couple of methods to perform some action within the class, such as the following:
- Lookup
- Add
- Delete
- Update
To add a new method, select the File | New | Method menu option. Alternatively, you can right-click the appropriate class and choose the New | Method menu option, or you can click the New Method toolbar button. This will enable you to define the new method. By default, the Properties tab is shown. This dialog box is shown in Figure 3.6.
FIGURE 3.6. The Properties tab of the Method Builder.
You must specify the name of the method. Using the earlier example, type Lookup, and then specify the arguments necessary for the method. Because you are going to be adding the customer whose attributes are specified as class properties, there is no reason to pass in an argument, so leave it blank. However, if you want to add an argument, click the + button. This enables you to add arguments and select data types for those arguments. When you have added the arguments you want, you can reorder them by highlighting each argument and clicking the up- and down-arrow buttons.
The next step is to choose the datatype that will be returned from the method, if applicable. Here are the choices: (None)
Byte
Boolean
Integer
Long
Single
Double
Currency
Date
String
Variant
Object Collection (None) is the default. In this case, this is exactly what you want. Next, you must choose from one of two check boxes. The first is to declare the method as a Friend member. This means, as stated earlier, that the method will be made available internally to the other class modules in the project, but not externally to the outside world, including other interfaces that can use projects. This enables your classes to communicate with each other.
The second tab on the Method Builder dialog box is the Attributes tab. It defines additional attributes, as shown in Figure 3.7.
FIGURE 3.7. The Attributes tab of the Method Builder.
These attributes are not functionally necessary. However, they do help to make the class complete. The Description field is for use by the type library, which will be generated with the class. The Project Help File field is read-only. It will show the project's Help file, if one is assigned. Finally, in the Help Context ID field you should enter the Help Context ID if you have a topic assigned to the property inside the Help file shown.
Continue adding all the methods shown earlier. When all methods are added, the Class Builder main window will look as it does in Figure 3.8.
Finally, to make this class, you'll probably want to construct at least one event, such as ClassError.
To add an event method, select the File | New | Event menu option. Alternatively, you can right-click the appropriate class and choose the New | Event menu option, or you can click the New Event toolbar button. This enables you to define the new event. There are two tabs in the dialog box. The first is the Properties tab, which is shown in Figure 3.9.
FIGURE 3.8. The VB Class Builder with all methods added.
FIGURE 3.9. The Properties tab of the Event Builder.
You must specify the name of the event. Using the preceding example, type ClassError, and then specify the arguments necessary for the event. Our event needs to have one argument, the error number. Therefore, name the argument Err. You add an argument by clicking on the + button. This enables you to add arguments and select datatypes for those arguments. Type Err for the name of the argument and select Integer for the datatype of the argument. After you have added the arguments you want, you can reorder them by highlighting the argument and clicking the up- and down-arrow buttons. Because you are using only one argument, you cannot reorder it.
The second tab on the Event Builder dialog box is the Attributes tab. It defines additional attributes, as shown in Figure 3.10.
FIGURE 3.10. The Attributes tab of the Event Builder.
These attributes are not functionally necessary. However, they do help to make the class complete. The Description field is for use by the type library, which will be generated with the class. The Project Help File field is read-only. It shows the project's Help file, if one is assigned. Finally, in the Help Context ID field you should enter the Help Context ID if you have a topic assigned to the property inside the Help file shown.
Now that you have defined the Customers class, define the Orders class with the members shown in Table 3.1.
Table 3.1. Members that need to be added to the Orders class.
Properties | Methods | Events |
CustomerID (Integer) | Add | ClassError() |
OrderNum (Integer) | Delete | |
LineNum (Integer) | Update | |
StockNum (String) | ||
Qty (Integer) | ||
Price (Currency) | ||
LineTotal (Currency) |
There are several options available to the Class Builder utility. The first option is the properties of the class itself. The properties consist of two tabs: Properties and Attributes. To open the properties, either right-click the class name and select the Properties menu option or select the Edit | Properties menu option. This brings up the Properties tab in the Class Module Builder dialog box, as shown in Figure 3.11.
FIGURE 3.11. The Properties tab of the Class Module Builder dialog box.
Here are the possible options:
- Private
- Public Not Creatable
- Single Use
- Global Single Use
- Multi Use
- Global Multi Use
These options are defined in the "Coding Classes" section, later in this chapter. The second tab in the Class Module Builder dialog box is the Attributes tab. It defines additional attributes, as shown in Figure 3.12.
These attributes are not functionally necessary. However, they do help to make the class complete. The Description field is for use by the type library, which will be generated with the class. The Project Help File field is read-only. It will show the project's Help file, if one is assigned. Finally, in the Help Context ID field you should enter the Help Context ID if you have a topic assigned to the property inside the Help file shown.
The second option available is the Class Builder Options dialog box. You invoke this by clicking the View | Options menu option. There are only two check boxes on this screen, as shown in Figure 3.13.
FIGURE 3.12. The Attributes tab of the Class Module Builder.
FIGURE 3.13. The Class Builder Options dialog box.
You can check the first option, "Include Debug code in Initialize and Terminate events," if you want to trace the Initialize() and Terminate() events. These events are automatically invoked when a class is initialized or terminated, respectively. These events are not always used. The "Include Err.Raise in all generated methods" option will raise an error in an error handler in all the methods you specified earlier.
After you have specified all the properties, methods, events, and options for each class you want to include, choose the File | Update Project menu option to have the Class Builder utility generate the class code for you. Figure 3.14 shows a project with the aforementioned members added.
Listing 3.1 shows the actual code generated from the Class Builder after specifying the Customers class members listed earlier. For further explanation of what the code means, refer to the section "Coding Classes," later in this chapter.
FIGURE 3.14. The project after the Class Builder-generated code.
Listing 3.1. Customers sample class code using the Class Builder.
`local variable(s) to hold property value(s) Private mvar_CustomerID As Integer `local copy Private mvar_Name As String `local copy Private mvar_Company As String `local copy Private mvar_Address1 As String `local copy Private mvar_Address2 As String `local copy Private mvar_City As String `local copy Private mvar_State As String `local copy Private mvar_Zip As String `local copy Private mvar_Phone As String `local copy Private mvar_Fax As String `local copy Private mvar_EMail As String `local copy `To fire this event, use RaiseEvent with the following syntax: `RaiseEvent ClassError[(arg1, arg2, ... , argn)] Public Event ClassError(ByVal Err As Integer) Public Sub Update() End Sub Public Sub Delete() End Sub Public Sub Add() End Sub Public Property Let EMail(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.EMail = 5 mvar_EMail = vData End Property Public Property Get EMail() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.EMail EMail = mvar_EMail End Property Public Property Let Fax(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.Fax = 5 mvar_Fax = vData End Property Public Property Get Fax() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.Fax Fax = mvar_Fax End Property Public Property Let Phone(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.Phone = 5 mvar_Phone = vData End Property Public Property Get Phone() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.Phone Phone = mvar_Phone End Property Public Property Let Zip(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.Zip = 5 mvar_Zip = vData End Property Public Property Get Zip() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.Zip Zip = mvar_Zip End Property Public Property Let State(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.State = 5 mvar_State = vData End Property Public Property Get State() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.State State = mvar_State End Property Public Property Let City(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.City = 5 mvar_City = vData End Property Public Property Get City() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.City City = mvar_City End Property Public Property Let Address2(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.Address2 = 5 mvar_Address2 = vData End Property Public Property Get Address2() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.Address2 Address2 = mvar_Address2 End Property Public Property Let Address1(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.Address1 = 5 mvar_Address1 = vData End Property Public Property Get Address1() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.Address1 Address1 = mvar_Address1 End Property Public Property Let Company(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.Company = 5 mvar_Company = vData End Property Public Property Get Company() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.Company Company = mvar_Company End Property Public Property Let Name(vData As String) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.Name = 5 mvar_Name = vData End Property Public Property Get Name() As String `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.Name Name = mvar_Name End Property Public Property Let CustomerID(vData As Integer) `used when assigning a value to the property, on the left side of an `assignment. Syntax: X.CustomerID = 5 mvar_CustomerID = vData End Property Public Property Get CustomerID() As Integer `used when retrieving value of a property, on the right side of an `assignment. Syntax: Debug.Print X.CustomerID CustomerID = mvar_CustomerID End Property
Add-In
The Add-In option builds a framework for constructing an add-in. Selecting this option will produce standard code for creating an add-in. It must be modified where appropriate. Add-ins are discussed in more detail in Chapter 4, "Add-Ins." There are no options available when you select this type of class module. Visual Basic simply generates the code shown in Listing 3.2. Use it as a reference for determining which Add New Class option you choose.
Listing 3.2. Add-in code generated by Visual Basic.
Option Explicit Implements IDTExtensibility Public FormDisplayed As Boolean Public VBInstance As VBIDE.VBE Dim mcbMenuCommandBar As Office.CommandBarControl Dim mfrmAddIn As New frmAddIn Public WithEvents MenuHandler As CommandBarEvents `command bar event handler Sub Hide() On Error Resume Next FormDisplayed = False mfrmAddIn.Hide End Sub Sub Show() On Error Resume Next If mfrmAddIn Is Nothing Then Set mfrmAddIn = New frmAddIn End If Set mfrmAddIn.VBInstance = VBInstance Set mfrmAddIn.Connect = Me FormDisplayed = True mfrmAddIn.Show End Sub `------------------------------------------------------ `this method adds the Add-In to VB `------------------------------------------------------ Private Sub IDTExtensibility_OnConnection(ByVal VBInst As Object, ÂByVal ConnectMode As vbext_ConnectMode, ByVal AddInInst As VBIDE.AddIn, Â custom() As Variant) On Error GoTo error_handler `save the vb instance Set VBInstance = VBInst `this is a good place to set a breakpoint and `test various addin objects, properties and methods Debug.Print VBInst.FullName If ConnectMode = vbext_cm_External Then `Used by the wizard toolbar to start this wizard Me.Show Else Set mcbMenuCommandBar = AddToAddInCommandBar("My AddIn") `sink the event Set Me.MenuHandler = VBInst.Events.CommandBarEvents(mcbMenuCommandBar) End If If ConnectMode = vbext_cm_AfterStartup Then If GetSetting(App.Title, "Settings", "DisplayOnConnect", "0") = "1" Then `set this to display the form on connect Me.Show End If End If Exit Sub error_handler: MsgBox Err.Description End Sub `------------------------------------------------------ `this method removes the Add-In from VB `------------------------------------------------------ Private Sub IDTExtensibility_OnDisconnection(ByVal RemoveMode As Âvbext_DisconnectMode, custom() As Variant) On Error Resume Next `delete the command bar entry mcbMenuCommandBar.Delete `shut down the Add-In If FormDisplayed Then SaveSetting App.Title, "Settings", "DisplayOnConnect", "1" FormDisplayed = False Else SaveSetting App.Title, "Settings", "DisplayOnConnect", "0" End If Unload mfrmAddIn Set mfrmAddIn = Nothing End Sub Private Sub IDTExtensibility_OnStartupComplete(custom() As Variant) If GetSetting(App.Title, "Settings", "DisplayOnConnect", "0") = "1" Then `set this to display the form on connect Me.Show End If End Sub Private Sub IDTExtensibility_OnAddInsUpdate(custom() As Variant) ` End Sub `this event fires when the menu is clicked in the IDE Private Sub MenuHandler_Click(ByVal CommandBarControl As Object, Âhandled As Boolean, CancelDefault As Boolean) Me.Show End Sub Function AddToAddInCommandBar(sCaption As String) As Office.CommandBarControl Dim cbMenuCommandBar As Office.CommandBarControl `command bar object Dim cbMenu As Object On Error GoTo AddToAddInCommandBarErr `see if we can find the Add-Ins menu Set cbMenu = VBInstance.CommandBars("Add-Ins") If cbMenu Is Nothing Then `not available so we fail Exit Function End If `add it to the command bar Set cbMenuCommandBar = cbMenu.Controls.Add(1) `set the caption cbMenuCommandBar.Caption = sCaption Set AddToAddInCommandBar = cbMenuCommandBar Exit Function AddToAddInCommandBarErr: End Function
Coding Classes
Regardless of which of the three options you choose when you add a new class module to your project, you must understand how class modules work and how to code them.
All class modules have a Name property that identifies the name of the class and is referred to in the form of Project.Class. This is the project name followed by the class name. In addition, depending on the type of project you create, you will have an Instancing property available. Table 3.2 shows the possible options and when they are available, based on the type of project created. You will notice that the Standard EXE column has all N/A values. This is because although you can use class modules in a standard executable, the class does not have an Instancing property.
Table 3.2. Instancing property values with different project types.
Instancing | Standard | ActiveX | ActiveX | ActiveX | ActiveX Document | ActiveX Document |
EXE | EXE | DLL | Control | DLL | EXE | |
Private | N/A | Yes | Yes | Yes | Yes | Yes |
PublicNotCreatable | N/A | Yes | Yes | Yes, | Yes | Yes |
Default | ||||||
SingleUse | N/A | Yes | No | No | No | Yes |
GlobalSingleUse | N/A | Yes | No | No | No | Yes |
MultiUse | N/A | Yes, | Yes, | No | Yes, | Yes, |
Default | Default | Default | Default | |||
GlobalMultiUse | N/A | Yes | No | No | No | Yes |
The Private setting dictates that no application can create any instances of the class and that only the component you are creating can use these objects.
The PublicNotCreatable setting indicates that other applications can use the object, but cannot create more than one instance of the object.
The SingleUse setting allows for another application to use the object, but another instance of the component is created, not just another instance of the object.
The GlobalSingleUse setting is the same as the SingleUse setting with one difference: Other applications view the object as if it were a global function.
The MultiUse setting enables other applications to create multiple instances of the object without having to create another instance of the component that calls the object.
Finally, the GlobalMultiUse setting is to the MultiUse setting as the GlobalSingleUse setting is to the SingleUse setting. This means that the members of the object can be used as if they were global functions.
The class module also has two events:
- Initialize()
- Terminate()
The Initialize() event is triggered when an instance of the class is created. If you need to initialize any variables or perform some other initializing code, place the code in this event. The Terminate() event is triggered when an instance of the class is destroyed. You can place any clean-up code in this event. It is not always necessary to place code in these events.
Adding Property Procedures
You declare properties by inserting property procedures. You can insert a property procedure by selecting the Tools | Add Procedure menu option. This brings up a dialog box that contains the types of procedures you can insert. Figure 3.15 shows how this dialog box looks. Select the Property option and type the name of the property.
FIGURE 3.15. The Add Procedure dialog box.
Choose the Public option to make the property available outside the class module. Choose Private to make the property local to the class. Visual Basic will actually create two special types of procedures for use with creating properties. By default, they are called Property Get and Property Let procedures. There is one other type of property procedure, called Property Set. A Property Get procedure will be called automatically, like a Visual Basic event, when a property is retrieved in code. For example, if you place the following code in the Debug window for a property declared as Color, where x is the name of an instance of a class module object, the Property Get Color() procedure will automatically be invoked:
?x.Color
It would then execute any code in that procedure, just as any other Visual Basic procedure. The procedure would be declared like this:
Public Property Get Color() End Sub
A Property Let procedure, as you might expect, will be invoked when you assign a value to a variable. For example, this code fragment would call the Property Let Color() procedure:
x.Color = RED
The declaration of such a procedure would look like this:
Public Property Let Color() End Sub
A Property Set procedure is used for assigning an object variable. Suppose you wanted to assign a form inside a property procedure. The following code fragment would automatically call the Property Set FormName() procedure:
x.FormName = Me
The declaration of this type of property looks like this:
Public Property Set Color() End Sub
To declare a property as being read-write, you place code in the Property Get and Property Let procedures. To make a property read-only, you can do one of two things. The first is to place the desired code in the Property Get procedure and place a warning message about being read-only in the Property Let procedure. The other thing you can do is actually remove the Property Let procedure from the Visual Basic code. This will generate a Visual Basic default message when the user tries to set the property. To declare a property as being write-only (such as an action property), you can do just the opposite. You can place code in the Property Let procedure and a warning message in the Property Get procedure. Otherwise, you can actually remove the Property Get procedure from the Visual Basic code.
Likewise, a Friend property is a property that is available only within the project, not the outside world. This means that, for example, Microsoft Excel cannot access the property, but another class within the same Visual Basic project can. Friend properties are written in pairs, like other property procedures with Get, Let, and Set. You would declare a Friend property with this code:
Friend Property Get Color() End Sub Friend Property Let Color() End Sub
Adding Methods
Adding a method is as simple as declaring a Public Sub. In a regular module, a Public Sub is a routine that will be available throughout the entire project. In a class module, a Public Sub creates a method that is exposed to the outside world. For example, the following code will make a method, called Add, available as a member of the class module that contains it:
Public Sub Add() End Sub
Likewise, a Friend Sub is a subroutine that creates a method, but the method is available only within the project, not the outside world. This means that, for example, Microsoft Excel cannot access the method, but another class within the same Visual Basic project can. You would declare a Friend subroutine with this code:
Friend Sub Add() End Sub
Adding Events
Before version 5.0, you could not add your own events. Adding an event is different than anything you are probably used to now. You add an event in five steps, as follows:
- 1. Declare the public event in the (general)(declarations) section of the class module. This simply declares the event, it doesn't actually fire it.
2. Within the class module, use the RaiseEvent statement to fire the event declared in the previous step. Pass the appropriate number of arguments.
3. In the form that instantiates the class module, you must declare a variable as being of the class module type. You must use the WithEvents keyword in the declaration to let Visual Basic know that an event will be fired for this object (class). For example, if you have a class named Customers, you can declare something like this:
Private WithEvents objCustomers As Customers
- 4. In the form_load event (or somewhere else if it makes sense) instantiate the class with a line of code similar to this:
Set objCustomers = New Customers
- 5. Everything you need has been set up except the coding for the actual event itself. You must write a subroutine that executes code as the event is fired. The subroutine name must be named with the object variable declared with the WithEvents keyword in step 3, followed by an underscore, and finally the name of the event as coded in the class module. This is somewhat of a foreign concept to Visual Basic programmers. For example, the ClassError event coded in the Customers class and declared in Listing 3.1 with the objCustomers variable would have a subroutine coded like this:
Private Sub ObjCustomers_ClassError(ByVal Err As Integer) MsgBox Str$(Err) End Sub
The object variable objCustomers is the name of the object for which the event will be raised. The event follows the object name, such as _ClassError. Note that the underscore character between the object name and the event name is necessary.
Putting It All Together
Now it's time to see how it all fits together. The basic concept we are trying to achieve is that the functionality of the system is encapsulated into the class modules so that to use the functionality, you do not have to know how the class modules work. Using one of the new classes created earlier (Customers), let's see how it all comes together. Consider the code from Listing 3.3, which is the coding for the class module, Customers. It is important to understand what is happening.
Listing 3.3. Customers class module code.
1:'local variable(s) to hold property value(s) 2:Private mvar_CustomerID As Integer `local copy 3:Private mvar_Name As String `local copy 4:Private mvar_Company As String `local copy 5:Private mvar_Address1 As String `local copy 6:Private mvar_Address2 As String `local copy 7:Private mvar_City As String `local copy 8:Private mvar_State As String `local copy 9:Private mvar_Zip As String `local copy 10:Private mvar_Phone As String `local copy 11:Private mvar_Fax As String `local copy 12:Private mvar_EMail As String `local copy 13:'To fire this event, use RaiseEvent with the following syntax: 14:'RaiseEvent ClassError[(arg1, arg2, ... , argn)] 15:Public Event ClassError(ByVal Err As Integer) 16:Public Sub Update() 17: `code to update record in database 18: Dim sCmd As String 19: 20: On Error GoTo UpdateError 21: 22: sCmd = "UPDATE Customers " 23: sCmd = sCmd & "SET Names = `" & mvar_Name & "`," 24: sCmd = sCmd & "Company = `" & mvar_Company & "`," 25: sCmd = sCmd & "Address1 = `" & mvar_Address1 & "`," 26: sCmd = sCmd & "Address2 = `" & mvar_Address2 & "`," 27: sCmd = sCmd & "City = `" & mvar_City & "`," 28: sCmd = sCmd & "State = `" & mvar_State & "`," 29: sCmd = sCmd & "Zip = `" & mvar_Zip & "`," 30: sCmd = sCmd & "Phone = `" & mvar_Phone & "`," 31: sCmd = sCmd & "Fax = `" & mvar_Fax & "`," 32: sCmd = sCmd & "EMail = `" & mvar_EMail & "` " 33: sCmd = sCmd & "WHERE CustomerID = " & mvar_CustomerID 34: dbOrders.Execute sCmd 35: Exit Sub 36: 37:UpdateError: 38: RaiseEvent ClassError(Err) 39:End Sub 40:Public Sub Delete() 41: `code to delete record from database 42: Dim sCmd As String 43: Dim nRes As Integer 44: 45: On Error GoTo DeleteError 46: If mvar_CustomerID = 0 Then 47: MsgBox "You must enter a customer ID" 48: Exit Sub 49: End If 50: 51: nRes = MsgBox("Confirm Delete", vbOKCancel) 52: If nRes = vbOK Then 53: sCmd = "DELETE FROM Customers " 54: sCmd = sCmd & "WHERE CustomerID = " & mvar_CustomerID & " " 55: dbOrders.Execute sCmd 56: End If 57: 58: Exit Sub 59:DeleteError: 60: RaiseEvent ClassError(Err) 61: 62:End Sub 63:Public Sub Add() 64: `code to add record to database 65: On Error GoTo AddError 66: Dim sCmd As String 67: 68: sCmd = "INSERT INTO Customers(CustomerID,Name,Company,Address1, ÂAddress2,City,State,Zip,Phone,Fax,EMail) " 69: sCmd = sCmd & "Values (" & mvar_CustomerID & "," 70: sCmd = sCmd & "`" & mvar_Name & "`," 71: sCmd = sCmd & "`" & mvar_Company & "`," 72: sCmd = sCmd & "`" & mvar_Address1 & "`," 73: sCmd = sCmd & "`" & mvar_Address2 & "`," 74: sCmd = sCmd & "`" & mvar_City & "`," 75: sCmd = sCmd & "`" & mvar_State & "`," 76: sCmd = sCmd & "`" & mvar_Zip & "`," 77: sCmd = sCmd & "`" & mvar_Phone & "`," 78: sCmd = sCmd & "`" & mvar_Fax & "`," 79: sCmd = sCmd & "`" & mvar_EMail & "`)" 80: dbOrders.Execute sCmd 81: Exit Sub 82: 83:AddError: 84: RaiseEvent ClassError(Err) 85:End Sub 86:Public Property Let EMail(vData As String) 87:'used when assigning a value to the property, on the left side of an 88:'assignment. Syntax: X.EMail = 5 89: mvar_EMail = vData 90:End Property 91:Public Property Get EMail() As String 92:'used when retrieving value of a property, on the right side of an 93:'assignment. Syntax: Debug.Print X.EMail 94: EMail = mvar_EMail 95:End Property 96:Public Property Let Fax(vData As String) 97:'used when assigning a value to the property, on the left side of an 98:'assignment. Syntax: X.Fax = 5 99: mvar_Fax = vData 100:End Property 101:Public Property Get Fax() As String 102:'used when retrieving value of a property, on the right side of an 103:'assignment. Syntax: Debug.Print X.Fax 104: Fax = mvar_Fax 105:End Property 106:Public Property Let Phone(vData As String) 107:'used when assigning a value to the property, on the left side of an 108:'assignment. Syntax: X.Phone = 5 109: mvar_Phone = vData 110:End Property 111:Public Property Get Phone() As String 112:'used when retrieving value of a property, on the right side of an 113:'assignment. Syntax: Debug.Print X.Phone 114: Phone = mvar_Phone 115:End Property 116:Public Property Let Zip(vData As String) 117:'used when assigning a value to the property, on the left side of an 118:'assignment. Syntax: X.Zip = 5 119: mvar_Zip = vData 120:End Property 121:Public Property Get Zip() As String 122:'used when retrieving value of a property, on the right side of an 123:'assignment. Syntax: Debug.Print X.Zip 124: Zip = mvar_Zip 125:End Property 126:Public Property Let State(vData As String) 127:'used when assigning a value to the property, on the left side of an 128:'assignment. Syntax: X.State = 5 129: mvar_State = vData 130:End Property 131:Public Property Get State() As String 132:'used when retrieving value of a property, on the right side of an 133:'assignment. Syntax: Debug.Print X.State 134: State = mvar_State 135:End Property 136:Public Property Let City(vData As String) 137:'used when assigning a value to the property, on the left side of an 138:'assignment. Syntax: X.City = 5 139: mvar_City = vData 140:End Property 141:Public Property Get City() As String 142:'used when retrieving value of a property, on the right side of an 143:'assignment. Syntax: Debug.Print X.City 144: City = mvar_City 145:End Property 146:Public Property Let Address2(vData As String) 147:'used when assigning a value to the property, on the left side of an 148:'assignment. Syntax: X.Address2 = 5 149: mvar_Address2 = vData 150:End Property 151:Public Property Get Address2() As String 152:'used when retrieving value of a property, on the right side of an 153:'assignment. Syntax: Debug.Print X.Address2 154: Address2 = mvar_Address2 155:End Property 156:Public Property Let Address1(vData As String) 157:'used when assigning a value to the property, on the left side of an 158:'assignment. Syntax: X.Address1 = 5 159: mvar_Address1 = vData 160:End Property 161:Public Property Get Address1() As String 162:'used when retrieving value of a property, on the right side of an 163:'assignment. Syntax: Debug.Print X.Address1 164: Address1 = mvar_Address1 165:End Property 166:Public Property Let Company(vData As String) 167:'used when assigning a value to the property, on the left side of an 168:'assignment. Syntax: X.Company = 5 169: mvar_Company = vData 170:End Property 171:Public Property Get Company() As String 172:'used when retrieving value of a property, on the right side of an 173:'assignment. Syntax: Debug.Print X.Company 174: Company = mvar_Company 175:End Property 176:Public Property Let Name(vData As String) 177:'used when assigning a value to the property, on the left side of an 178:'assignment. Syntax: X.Name = 5 179: mvar_Name = vData 180:End Property 181:Public Property Get Name() As String 182:'used when retrieving value of a property, on the right side of an 183:'assignment. Syntax: Debug.Print X.Name 184: Name = mvar_Name 185:End Property 186:Public Property Let CustomerID(vData As Integer) 187:'used when assigning a value to the property, on the left side of an 188:'assignment. Syntax: X.CustomerID = 5 189: mvar_CustomerID = vData 190:End Property 191:Public Property Get CustomerID() As Integer 192:'used when retrieving value of a property, on the right side of an 193:'assignment. Syntax: Debug.Print X.CustomerID 194: CustomerID = mvar_CustomerID 195:End Property 196:Public Sub Lookup() 197: `code to lookup record to database 198: Dim sCmd As String 199: Dim rsTemp As Recordset 200: 201: sCmd = "SELECT * FROM Customers " 202: sCmd = sCmd & "WHERE CustomerID = " & mvar_CustomerID & " " 203: Set rsTemp = dbOrders.OpenRecordset(sCmd) 204: If Not rsTemp.EOF Then 205: If Not IsNull(rsTemp.Fields("Name")) Then 206: mvar_Name = rsTemp.Fields("Name") 207: Else 208: mvar_Name = "" 209: End If 210: 211: If Not IsNull(rsTemp.Fields("Company")) Then 212: mvar_Company = rsTemp.Fields("Company") 213: Else 214: mvar_Company = "" 215: End If 216: 217: If Not IsNull(rsTemp.Fields("Address1")) Then 218: mvar_Address1 = rsTemp.Fields("Address1") 219: Else 220: mvar_Address1 = "" 221: End If 222: 223: If Not IsNull(rsTemp.Fields("Address2")) Then 224: mvar_Address2 = rsTemp.Fields("Address2") 225: Else 226: mvar_Address2 = "" 227: End If 228: 229: If Not IsNull(rsTemp.Fields("City")) Then 230: mvar_City = rsTemp.Fields("City") 231: Else 232: mvar_City = "" 233: End If 234: 235: If Not IsNull(rsTemp.Fields("State")) Then 236: mvar_State = rsTemp.Fields("State") 237: Else 238: mvar_State = "" 239: End If 240: 241: If Not IsNull(rsTemp.Fields("Zip")) Then 242: mvar_Zip = rsTemp.Fields("Zip") 243: Else 244: mvar_Zip = "" 245: End If 246: 247: If Not IsNull(rsTemp.Fields("Phone")) Then 248: mvar_Phone = rsTemp.Fields("Phone") 249: Else 250: mvar_Phone = "" 251: End If 252: 253: If Not IsNull(rsTemp.Fields("Fax")) Then 254: mvar_Fax = rsTemp.Fields("Fax") 255: Else 256: mvar_Fax = "" 257: End If 258: 259: If Not IsNull(rsTemp.Fields("EMail")) Then 260: mvar_EMail = rsTemp.Fields("EMail") 261: Else 262: mvar_EMail = "" 263: End If 264: Else 265: MsgBox "ID does not exist" 266: mvar_CustomerID = -1 267: End If 268: 269:End Sub
Lines 2-12 declare local copies of variables that equate one-for-one with the properties declared. This is because the property itself is available in the instantiated class that is available in a form or module. The property is not available inside the class; therefore, the value is assigned to a local copy of a variable.
L ine 15 declares the ClassError event.
Lines 16-39 contain the code for the Update method of the Customers class. Notice that the code on line 38 raises the ClassError event.
Lines 40-62 contain the code for the Delete method of the Customers class. Notice that the code on line 60 raises the ClassError event.
Lines 63-85 contain the code for the Add method of the Customers class. Notice that the code on line 84 raises the ClassError event.
Lines 86-195 declare and code the Property Let and Property Get procedures for all of the properties of the Customers class.
Lines 196-269 contain the code for the Lookup method of the Customers class.
You can expand this functionality in your own application to include a form for the Orders class. You can also take this application one step further and add multiple customers to a collection of customers. Collections are discussed in Chapter 22, "Collections."
Summary
Classes were first introduced in Visual Basic version 4.0. Classes give Visual Basic the possibility to reuse code and create properties, methods, and events with which you can encapsulate code. Encapsulating code is an important concept that enables a developer to create and distribute classes or objects in order to shield other developers and applications from the complexity of the code in the class. The capability to create custom events is new to version 5.0. This is made possible through the use of the WithEvents keyword.
This chapter went into great detail in creating classes both manually and with the Class Builder Utility. Use it as a reference for your applications.
© Copyright, Macmillan Computer Publishing. All rights reserved.
Table of Contents
Visual Basic 5 Developers Guide
Table of Contents:
- Introduction
I Concepts -
- Chapter 1 - The Visual Basic Environment and Development Life Cycle
- Chapter 2 - Stock Wizards
- Chapter 3 - Classes
- Chapter 4 - Add-Ins
- Chapter 5 - Testing and Debugging
II The Windows Execution Environment
- Chapter 6 - The Registry
- Chapter 7 - Working with Files
- Chapter 8 - Graphics
- Chapter 9 - Printing
- Chapter 10 - Writing Resource Files
- Chapter 11 - Multimedia
- Chapter 12 - Dynamic Link Libraries
- Chapter 13 - Using the WIN32 API
- Chapter 14 - Integrating with Office 97
III Enterprise-Specific Features
- Chapter 15 - Visual SourceSafe
- Chapter 16 - Remote Data Objects (RDO)
- Chapter 17 - T-SQL Debugger
- Chapter 18 - Microsoft Transaction Server
IV Controls and Containers
- Chapter 19 - Components and Objects
- Chapter 20 - ActiveX Controls
- Chapter 21 - OLE and OLE Automation
- Chapter 22 - Collections
V Communications, Internet, and Intranet Building
- Chapter 23 - The Internet
- Chapter 24 - Special Considerations
- Chapter 25 - HTML Primer
- Chapter 26 - MAPI and Microsoft Exchange
- Chapter 27 - TAPI
VI Databases
- Chapter 28 - Database Concepts
- Chapter 29 - Structured Query Language (SQL)
- Chapter 30 - ODBC
- Chapter 31 - Database Applications with Access 97
- Chapter 32 - Visual Basic's Role in Client/Server Computing
- Chapter 33 - SQL Server 6.5 Features
- Chapter 34 - Crystal Reports
VII Rapid Application Development
- Chapter 35 - Developing a CD Player Application
- Chapter 36 - Developing a MAPI/Microsoft Exchange Application
- Chapter 37 - Developing a TAPI Application
- Chapter 38 - Data Sharing with Microsoft Office
- Chapter 39 - Creating a Wizard Add-In
- Chapter 40 - Developing a SAPI Application
- Chapter 41 - Developing an Access-to-SQL Server Upsizing Application
- Chapter 42 - A Client/Server Application
- Chapter 43 - Creating an Electronic Commerce Internet Application
VIII Appendixes
- Appendix A - Third-Party Vendors
- Appendix B - Error Tables
© Copyright, Macmillan Computer Publishing. All rights reserved.