Debugging is crucial to successful software development, but even many experienced programmers find it challenging. Sophisticated debugging tools are available, yet it may be difficult to determine which features are useful in which situations. The Art of Debugging is your guide to making the debugging process more efficient and effective.
The Art of Debugging illustrates the use three of the most popular debugging tools on Linux/Unix platforms: GDB, DDD, and Eclipse. The text-command based GDB (the GNU Project Debugger) is included with most distributions. DDD is a popular GUI front end for GDB, while Eclipse provides a complete integrated development environment.
In addition to offering specific advice for debugging with each tool, authors Norm Matloff and Pete Salzman cover general strategies for improving the process of finding and fixing coding errors, including how to:
–Inspect variables and data structures
–Understand segmentation faults and core dumps
–Know why your program crashes or throws exceptions
–Use features like catchpoints, convenience variables, and artificial arrays
–Avoid common debugging pitfalls
Real world examples of coding errors help to clarify the authors’ guiding principles, and coverage of complex topics like thread, client-server, GUI, and parallel programming debugging will make you even more proficient. You'll also learn how to prevent errors in the first place with text editors, compilers, error reporting, and static code checkers.
Whether you dread the thought of debugging your programs or simply want to improve your current debugging efforts, you'll find a valuable ally in The Art of Debugging.
1100360907
The Art of Debugging illustrates the use three of the most popular debugging tools on Linux/Unix platforms: GDB, DDD, and Eclipse. The text-command based GDB (the GNU Project Debugger) is included with most distributions. DDD is a popular GUI front end for GDB, while Eclipse provides a complete integrated development environment.
In addition to offering specific advice for debugging with each tool, authors Norm Matloff and Pete Salzman cover general strategies for improving the process of finding and fixing coding errors, including how to:
–Inspect variables and data structures
–Understand segmentation faults and core dumps
–Know why your program crashes or throws exceptions
–Use features like catchpoints, convenience variables, and artificial arrays
–Avoid common debugging pitfalls
Real world examples of coding errors help to clarify the authors’ guiding principles, and coverage of complex topics like thread, client-server, GUI, and parallel programming debugging will make you even more proficient. You'll also learn how to prevent errors in the first place with text editors, compilers, error reporting, and static code checkers.
Whether you dread the thought of debugging your programs or simply want to improve your current debugging efforts, you'll find a valuable ally in The Art of Debugging.
The Art of Debugging with GDB, DDD, and Eclipse
Debugging is crucial to successful software development, but even many experienced programmers find it challenging. Sophisticated debugging tools are available, yet it may be difficult to determine which features are useful in which situations. The Art of Debugging is your guide to making the debugging process more efficient and effective.
The Art of Debugging illustrates the use three of the most popular debugging tools on Linux/Unix platforms: GDB, DDD, and Eclipse. The text-command based GDB (the GNU Project Debugger) is included with most distributions. DDD is a popular GUI front end for GDB, while Eclipse provides a complete integrated development environment.
In addition to offering specific advice for debugging with each tool, authors Norm Matloff and Pete Salzman cover general strategies for improving the process of finding and fixing coding errors, including how to:
–Inspect variables and data structures
–Understand segmentation faults and core dumps
–Know why your program crashes or throws exceptions
–Use features like catchpoints, convenience variables, and artificial arrays
–Avoid common debugging pitfalls
Real world examples of coding errors help to clarify the authors’ guiding principles, and coverage of complex topics like thread, client-server, GUI, and parallel programming debugging will make you even more proficient. You'll also learn how to prevent errors in the first place with text editors, compilers, error reporting, and static code checkers.
Whether you dread the thought of debugging your programs or simply want to improve your current debugging efforts, you'll find a valuable ally in The Art of Debugging.
The Art of Debugging illustrates the use three of the most popular debugging tools on Linux/Unix platforms: GDB, DDD, and Eclipse. The text-command based GDB (the GNU Project Debugger) is included with most distributions. DDD is a popular GUI front end for GDB, while Eclipse provides a complete integrated development environment.
In addition to offering specific advice for debugging with each tool, authors Norm Matloff and Pete Salzman cover general strategies for improving the process of finding and fixing coding errors, including how to:
–Inspect variables and data structures
–Understand segmentation faults and core dumps
–Know why your program crashes or throws exceptions
–Use features like catchpoints, convenience variables, and artificial arrays
–Avoid common debugging pitfalls
Real world examples of coding errors help to clarify the authors’ guiding principles, and coverage of complex topics like thread, client-server, GUI, and parallel programming debugging will make you even more proficient. You'll also learn how to prevent errors in the first place with text editors, compilers, error reporting, and static code checkers.
Whether you dread the thought of debugging your programs or simply want to improve your current debugging efforts, you'll find a valuable ally in The Art of Debugging.
23.99
In Stock
5
1
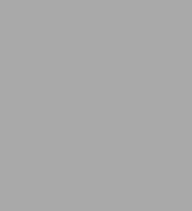
The Art of Debugging with GDB, DDD, and Eclipse
280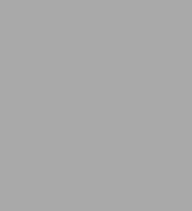
The Art of Debugging with GDB, DDD, and Eclipse
280Related collections and offers
23.99
In Stock
Product Details
ISBN-13: | 9781593272319 |
---|---|
Publisher: | No Starch Press |
Publication date: | 09/15/2008 |
Sold by: | Penguin Random House Publisher Services |
Format: | eBook |
Pages: | 280 |
File size: | 4 MB |
About the Author
From the B&N Reads Blog