"This book should be on every C++ programmer’s desk. It’s clear, concise, and valuable." - Rob Green, Bowling Green State University
This bestseller has been updated and revised to cover all the latest changes to C++ 14 and 17! C++ Concurrency in Action, Second Edition teaches you everything you need to write robust and elegant multithreaded applications in C++17.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
You choose C++ when your applications need to run fast. Well-designed concurrency makes them go even faster. C++ 17 delivers strong support for the multithreaded, multiprocessor programming required for fast graphic processing, machine learning, and other performance-sensitive tasks. This exceptional book unpacks the features, patterns, and best practices of production-grade C++ concurrency.
About the Book
C++ Concurrency in Action, Second Edition is the definitive guide to writing elegant multithreaded applications in C++. Updated for C++ 17, it carefully addresses every aspect of concurrent development, from starting new threads to designing fully functional multithreaded algorithms and data structures. Concurrency master Anthony Williams presents examples and practical tasks in every chapter, including insights that will delight even the most experienced developer.
What's inside
About the Reader
Written for intermediate C and C++ developers. No prior experience with concurrency required.
About the Author
Anthony Williams has been an active member of the BSI C++ Panel since 2001 and is the developer of the just::thread Pro extensions to the C++ 11 thread library.
Table of Contents
1132543254
This bestseller has been updated and revised to cover all the latest changes to C++ 14 and 17! C++ Concurrency in Action, Second Edition teaches you everything you need to write robust and elegant multithreaded applications in C++17.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
You choose C++ when your applications need to run fast. Well-designed concurrency makes them go even faster. C++ 17 delivers strong support for the multithreaded, multiprocessor programming required for fast graphic processing, machine learning, and other performance-sensitive tasks. This exceptional book unpacks the features, patterns, and best practices of production-grade C++ concurrency.
About the Book
C++ Concurrency in Action, Second Edition is the definitive guide to writing elegant multithreaded applications in C++. Updated for C++ 17, it carefully addresses every aspect of concurrent development, from starting new threads to designing fully functional multithreaded algorithms and data structures. Concurrency master Anthony Williams presents examples and practical tasks in every chapter, including insights that will delight even the most experienced developer.
What's inside
- Full coverage of new C++ 17 features
- Starting and managing threads
- Synchronizing concurrent operations
- Designing concurrent code
- Debugging multithreaded applications
About the Reader
Written for intermediate C and C++ developers. No prior experience with concurrency required.
About the Author
Anthony Williams has been an active member of the BSI C++ Panel since 2001 and is the developer of the just::thread Pro extensions to the C++ 11 thread library.
Table of Contents
- Hello, world of concurrency in C++!
- Managing threads
- Sharing data between threads
- Synchronizing concurrent operations
- The C++ memory model and operations on atomic types
- Designing lock-based concurrent data structures
- Designing lock-free concurrent data structures
- Designing concurrent code
- Advanced thread management
- Parallel algorithms
- Testing and debugging multithreaded applications
C++ Concurrency in Action
"This book should be on every C++ programmer’s desk. It’s clear, concise, and valuable." - Rob Green, Bowling Green State University
This bestseller has been updated and revised to cover all the latest changes to C++ 14 and 17! C++ Concurrency in Action, Second Edition teaches you everything you need to write robust and elegant multithreaded applications in C++17.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
You choose C++ when your applications need to run fast. Well-designed concurrency makes them go even faster. C++ 17 delivers strong support for the multithreaded, multiprocessor programming required for fast graphic processing, machine learning, and other performance-sensitive tasks. This exceptional book unpacks the features, patterns, and best practices of production-grade C++ concurrency.
About the Book
C++ Concurrency in Action, Second Edition is the definitive guide to writing elegant multithreaded applications in C++. Updated for C++ 17, it carefully addresses every aspect of concurrent development, from starting new threads to designing fully functional multithreaded algorithms and data structures. Concurrency master Anthony Williams presents examples and practical tasks in every chapter, including insights that will delight even the most experienced developer.
What's inside
About the Reader
Written for intermediate C and C++ developers. No prior experience with concurrency required.
About the Author
Anthony Williams has been an active member of the BSI C++ Panel since 2001 and is the developer of the just::thread Pro extensions to the C++ 11 thread library.
Table of Contents
This bestseller has been updated and revised to cover all the latest changes to C++ 14 and 17! C++ Concurrency in Action, Second Edition teaches you everything you need to write robust and elegant multithreaded applications in C++17.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
You choose C++ when your applications need to run fast. Well-designed concurrency makes them go even faster. C++ 17 delivers strong support for the multithreaded, multiprocessor programming required for fast graphic processing, machine learning, and other performance-sensitive tasks. This exceptional book unpacks the features, patterns, and best practices of production-grade C++ concurrency.
About the Book
C++ Concurrency in Action, Second Edition is the definitive guide to writing elegant multithreaded applications in C++. Updated for C++ 17, it carefully addresses every aspect of concurrent development, from starting new threads to designing fully functional multithreaded algorithms and data structures. Concurrency master Anthony Williams presents examples and practical tasks in every chapter, including insights that will delight even the most experienced developer.
What's inside
- Full coverage of new C++ 17 features
- Starting and managing threads
- Synchronizing concurrent operations
- Designing concurrent code
- Debugging multithreaded applications
About the Reader
Written for intermediate C and C++ developers. No prior experience with concurrency required.
About the Author
Anthony Williams has been an active member of the BSI C++ Panel since 2001 and is the developer of the just::thread Pro extensions to the C++ 11 thread library.
Table of Contents
- Hello, world of concurrency in C++!
- Managing threads
- Sharing data between threads
- Synchronizing concurrent operations
- The C++ memory model and operations on atomic types
- Designing lock-based concurrent data structures
- Designing lock-free concurrent data structures
- Designing concurrent code
- Advanced thread management
- Parallel algorithms
- Testing and debugging multithreaded applications
52.99
In Stock
5
1
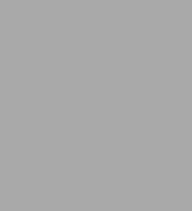
C++ Concurrency in Action
592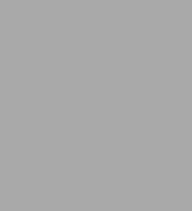
C++ Concurrency in Action
592Related collections and offers
52.99
In Stock
From the B&N Reads Blog