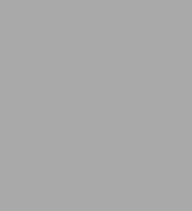
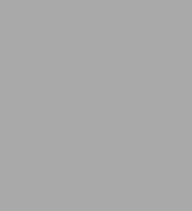
eBook
Related collections and offers
Overview
Java RMI contains a wealth of experience in designing and implementing Java's Remote Method Invocation. If you're a novice reader, you will quickly be brought up to speed on why RMI is such a powerful yet easy to use tool for distributed programming, while experts can gain valuable experience for constructing their own enterprise and distributed systems.With Java RMI, you'll learn tips and tricks for making your RMI code excel. The book also provides strategies for working with serialization, threading, the RMI registry, sockets and socket factories, activation, dynamic class downloading, HTTP tunneling, distributed garbage collection, JNDI, and CORBA. In short, a treasure trove of valuable RMI knowledge packed into one book.
Product Details
ISBN-13: | 9781449315351 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 10/22/2001 |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 576 |
File size: | 5 MB |
About the Author
Read an Excerpt
Chapter 10: Serialization
Serialization is the process of converting a set of object instances that contain references to each other into a linear stream of bytes, which can then be sent through a socket, stored to a file, or simply manipulated as a stream of data. Serialization is the mechanism used by RMI to pass objects between JVMs, either as arguments in a method invocation from a client to a server or as return values from a method invocation. In the first section of this book, I referred to this process several times but delayed a detailed discussion until now. In this chapter, we drill down on the serialization mechanism; by the end of it, you will understand exactly how serialization works and how to use it efficiently within your applications.
The Need for Serialization
Envision the banking application while a client is executing a withdrawal. The part of the application we're looking at has the runtime structure shown in Figure 10-1.
What does it mean for the client to pass an instance of Money
to the server? At a minimum, it means that the server is able to call public methods on the instance of Money
. One way to do this would be to implicitly make Money
into a server as well.1 For example, imagine that the client sends the following two pieces of information whenever it passes an instance as an argument:
- The type of the instance; in this case,
Money
. - A unique identifier for the object (i.e., a logical reference). For example, the address of the instance in memory.
The RMI runtime layer in the server can use this information to construct a stub for the instance of Money
, so that whenever the Account
server calls a method on what it thinks of as the instance of Money
, the method call is relayed over the wire, as shown in Figure 10-2.
Attempting to do things this way has three significant drawbacks:
- You can't access fields on the objects that have been passed as arguments.
Stubs work by implementing an interface. They implement the methods in the interface by simply relaying the method invocation across the network. That is, the stub methods take all their arguments and simply marshall them for transport across the wire. Accessing a public field is really just dereferencing a pointer--there is no method invocation and hence, there isn't a method call to forward over the wire.
- It can result in unacceptable performance due to network latency.
Even in our simple case, the instance of
Account
is going to need to callgetCents( )
on the instance ofMoney
. This means that a simple call tomakeDeposit( )
really involves at least two distinct networked method calls:makeDeposit( )
from the client andgetCents( )
from the server. - It makes the application much more vulnerable to partial failure.
Let's say that the server is busy and doesn't get around to handling the request for 30 seconds. If the client crashes in the interim, or if the network goes down, the server cannot process the request at all. Until all data has been requested and sent, the application is particularly vulnerable to partial failures.
This last point is an interesting one. Any time you have an application that requires a long-lasting and durable connection between client and server, you build in a point of failure. The longer the connection needs to last, or the higher the communication bandwidth the connection requires, the more likely the application is to occasionally break down.
TIP: The original design of the Web, with its stateless connections, serves as a good example of a distributed application that can tolerate almost any transient network failure.
These three reasons imply that what is really needed is a way to copy objects and send them over the wire. That is, instead of turning arguments into implicit servers, arguments need to be completely copied so that no further network calls are needed to complete the remote method invocation. Put another way, we want the result of makeWithdrawal( )
to involve creating a copy of the instance of Money
on the server side. The runtime structure should resemble Figure 10-3.
The desire to avoid unnecessary network dependencies has two significant consequences:
- Once an object is duplicated, the two objects are completely independent of each other.
Any attempt to keep the copy and the original in sync would involve propagating changes over the network, entirely defeating the reason for making the copy in the first place.
- The copying mechanism must create deep copies.
If the instance of
Money
references another instance, then copies must be made of both instances. Otherwise, when a method is called on the second object, the call must be relayed across the wire. Moreover, all the copies must be made immediately--we can't wait until the second object is accessed to make the copy because the original might change in the meantime.
These two consequences have a very important third consequence:
- If an object is sent twice, in separate method calls, two copies of the object will be created.
In addition to arguments to method calls, this holds for objects that are referenced by the arguments. If you pass object A, which has a reference to object C, and in another call you pass object B, which also has a reference to C, you will end up with two distinct copies of C on the receiving side.
Drilling Down on Object Creation
To see why this last point holds, consider a client that executes a withdrawal and then tries to cancel the transaction by making a deposit for the same amount of money. That is, the following lines of code are executed:
server.makeWithdrawal(amount);
....
server.makeDeposit(amount);
The client has no way of knowing whether the server still has a copy of amount
. After all, the server may have used it and then thrown the copy away once it was done. This means that the client has to marshall amount
and send it over the wire to the server.
The RMI runtime can demarshall amount
, which is the instance of Money
the client sent. However, even if it has the previous object, it has no way (unless equals( )
has been overridden) to tell whether the instance it just demarshalled is equal to the previous object.
More generally, if the object being copied isn't immutable, then the server might change it. In this case, even if the two objects are currently equal, the RMI runtime has no way to tell if the two copies will always be equal and can potentially be replaced by a single copy. To see why, consider our Printer
example again. At the end of Chapter 3, we considered a list of possible feature requests that could be made. One of them was the following:
Managers will want to track resource consumption. This will involve logging print requests and, quite possibly, building a set of queries that can be run against the printer's log.
This can be implemented by adding a few more fields to DocumentDescription
and having the server store an indexed log of all the DocumentDescription
objects it has received. For example, we may add the following fields to DocumentDescription
:
public Time whenPrinted;
public Person sender;
public boolean printSucceeded;
Now consider what happens when the user actually wants to print two copies of the same document. The client application could call:
server.printDocument(document);
twice with the "same" instance of DocumentDescription
. And it would be an error for the RMI runtime to create only one instance of DocumentDescription
on the server side. Even though the "same" object is passed into the server twice, it is passed as parts of distinct requests and therefore as different objects.
TIP: This is true even if the runtime can tell that the two instances of
DocumentDescription
are equal when it finishes demarshalling. An implementation of a printer may well have a notion of a job queue that holds instances ofDocumentDescription
. So our client makes the first call, and the copy ofdocument
is placed in the queue (say, at number 5), but not edited because the document hasn't been printed yet. Then our client makes the second call. At this point, the two copies ofdocument
are equal. However, we don't want to place the same object in the printer queue twice. We want to place distinct copies in the printer queue.
Thus, we come to the following conclusion: network latency, and the desire to avoid vulnerability to partial failures, force us to have a deep copy mechanism for most arguments to a remote method invocation. This copying mechanism has to make deep copies, and it cannot perform any validation to eliminate "extra" copies across methods.
TIP: While this discussion provides examples of implementation decisions that force two copies to occur, it's important to note that, even without such examples, clients should be written as if the servers make independent copies. That is, clients are written to use interfaces. They should not, and cannot, make assumptions about server-side implementations of the interfaces.
Using Serialization
Serialization is a mechanism built into the core Java libraries for writing a graph of objects into a stream of data. This stream of data can then be programmatically manipulated, and a deep copy of the objects can be made by reversing the process. This reversal is often called deserialization.
In particular, there are three main uses of serialization:
- As a persistence mechanism
- If the stream being used is
FileOutputStream
, then the data will automatically be written to a file. - As a copy mechanism
- If the stream being used is
ByteArrayOutputStream
, then the data will be written to a byte array in memory. This byte array can then be used to create duplicates of the original objects. - As a communication mechanism
- If the stream being used comes from a socket, then the data will automatically be sent over the wire to the receiving socket, at which point another program will decide what to do.
The important thing to note is that the use of serialization is independent of the serialization algorithm itself. If we have a serializable class, we can save it to a file or make a copy of it simply by changing the way we use the output of the serialization mechanism.
As you might expect, serialization is implemented using a pair of streams. Even though the code that underlies serialization is quite complex, the way you invoke it is designed to make serialization as transparent as possible to Java developers. To serialize an object, create an instance of ObjectOutputStream
and call the writeObject( )
method; to read in a serialized object, create an instance of ObjectInputStream
and call the readObject( )
object....
Table of Contents
PrefacePart I. Designing and Building: The Basics of RMI Applications
1. Streams
The Core Classes
Viewing a File
Layering Streams
Readers and Writers
2. Sockets
Internet Definitions
Sockets
ServerSockets
Customizing Socket Behavior
Special-Purpose Sockets
Using SSL
3. A Socket-Based Printer Server
A Network-Based Printer
The Basic Objects
The Protocol
The Application Itself
Evolving the Application
4. The Same Server, Written Using RMI
The Basic Structure of RMI
The Architecture Diagram Revisited
Implementing the Basic Objects
The Rest of the Server
The Client Application
Summary
5. Introducing the Bank Example
The Bank Example
Sketching a Rough Architecture
The Basic Use Case
Additional Design Decisions
A Distributed Architecture for the Bank Example
Problems That Arise in Distributed Applications
6. Deciding on the Remote Server
A Little Bit of Bias
Important Questions When Thinking About Servers
Should We Implement Bank or Account?
7. Designing the Remote Interface
Important Questions When Designing Remote Interfaces
Building the Data Objects
Accounting for Partial Failure
8. Implementing the Bank Server
The Structure of a Server
Implementing the Server
Generating Stubs and Skeletons
9. The Rest of the Application
The Need for Launch Code
Our Actual Launch Code
Build Test Applications
Buildthe Client Application
Deploying the Application
Part II. Drilling Down: Scalability
10. Serialization
The Need for Serialization
Using Serialization
How to Make a Class Serializable
The Serialization Algorithm
Versioning Classes
Performance Issues
The Externalizable Interface
11. Threads
More Than One Client
Basic Terminology
Threading Concepts
Support for Threads in Java
Deadlock
Threading and RMI
12. Implementing Threading
The Basic Task
Guidelines for Threading
Pools: An Extended Example
Some Final Words on Threading
13. Testing a Distributed Application
Testing the Bank Application
14. The RMI Registry
Why Use a Naming Service?
The RMI Registry
The RMI Registry Is an RMI Server
Examining the Registry
Limitations of the RMI Registry
Security Issues
15. Naming Services
Basic Design, Terminology, and Requirements
Requirements for Our Naming Service
Federation and Threading
The Context Interface
The Value Objects
ContextImpl
Switching Between Naming Services
The Java Naming and Directory Interface (JNDI)
16. The RMI Runtime
Reviewing the Mechanics of a Remote Method Call
Distributed Garbage Collection
RMI's Logging Facilities
Other JVM Parameters
17. Factories and the Activation Framework
Resource Management
Factories
Implementing a Generic Factory
A Better Factory
Persistence and the Server Lifecycle
Activation
A Final Word About Factories
Part III. Advanced Topics
18. Using Custom Sockets
Custom Socket Factories
Incorporating a Custom Socket into an Application
19. Dynamic Classloading
Deploying Can Be Difficult
Classloaders
How Dynamic Classloading Works
The Class Server
Using Dynamic Classloading in an Application
20. Security Policies
A Different Kind of Security Problem
Permissions
Security Managers
Setting Up a Security Policy
21. Multithreaded Clients
Different Types of Remote Methods
Handling Printer-Type Methods
Handling Report-Type Methods
Generalizing from These Examples
22. HTTP Tunneling
Firewalls
CGI and Dynamic Content
HTTP Tunneling
A Servlet Implementation of HTTP Tunneling
Modifying the Tunneling Mechanism
The Bank via HTTP Tunneling
Drawbacks of HTTP Tunneling
Disabling HTTP Tunneling
23. RMI, CORBA, and RMI/IIOP
How CORBA Works
The Bank Example in CORBA
A Quick Comparison of CORBA and RMI
RMI on Top of CORBA
Converting the Bank Example to RMI/IIOP
Index