When it comes to writing efficient code, every software professional needs to have an effective working knowledge of algorithms. In this practical book, author George Heineman (Algorithms in a Nutshell) provides concise and informative descriptions of key algorithms that improve coding. Software developers, testers, and maintainers will discover how algorithms solve computational problems creatively.
Each chapter builds on earlier chapters through eye-catching visuals and a steady rollout of essential concepts, including an algorithm analysis to classify the performance of every algorithm presented in the book. At the end of each chapter, you'll get to apply what you've learned to a novel challenge problem -- simulating the experience you might find in a technical code interview.
With this book, you will:
- Examine fundamental algorithms central to computer science and software engineering
- Learn common strategies for efficient problem solving -- such as divide and conquer, dynamic programming, and greedy approaches
- Analyze code to evaluate time complexity using big O notation
- Use existing Python libraries and data structures to solve problems using algorithms
- Understand the main steps of important algorithms
When it comes to writing efficient code, every software professional needs to have an effective working knowledge of algorithms. In this practical book, author George Heineman (Algorithms in a Nutshell) provides concise and informative descriptions of key algorithms that improve coding. Software developers, testers, and maintainers will discover how algorithms solve computational problems creatively.
Each chapter builds on earlier chapters through eye-catching visuals and a steady rollout of essential concepts, including an algorithm analysis to classify the performance of every algorithm presented in the book. At the end of each chapter, you'll get to apply what you've learned to a novel challenge problem -- simulating the experience you might find in a technical code interview.
With this book, you will:
- Examine fundamental algorithms central to computer science and software engineering
- Learn common strategies for efficient problem solving -- such as divide and conquer, dynamic programming, and greedy approaches
- Analyze code to evaluate time complexity using big O notation
- Use existing Python libraries and data structures to solve problems using algorithms
- Understand the main steps of important algorithms
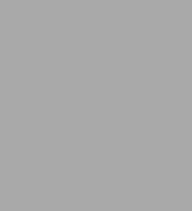
Learning Algorithms: A Programmer's Guide to Writing Better Code
280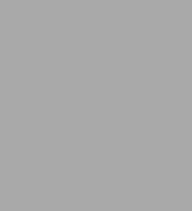
Learning Algorithms: A Programmer's Guide to Writing Better Code
280Related collections and offers
Product Details
ISBN-13: | 9781492091011 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 07/20/2021 |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 280 |
File size: | 9 MB |
About the Author
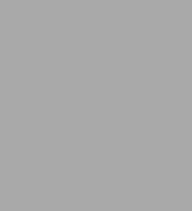