”Demystifies object-oriented programming, and lays out how to use it to design truly secure and performant applications.” —Charles Soetan, Plum.io
Key Features
Dozens of techniques for writing object-oriented code that’s easy to read, reuse, and maintain
Write code that other programmers will instantly understand
Design rules for constructing objects, changing and exposing state, and more
Examples written in an instantly familiar pseudocode that’s easy to apply to Java, Python, C#, and any object-oriented language
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About The Book
Well-written object-oriented code is easy to read, modify, and debug. Elevate your coding style by mastering the universal best practices for object design presented in this book. These clearly presented rules, which apply to any OO language, maximize the clarity and durability of your codebase and increase productivity for you and your team.
In Object Design Style Guide, veteran developer Matthias Noback lays out design rules for constructing objects, defining methods, and much more. All examples use instantly familiar pseudocode, so you can follow along in the language you prefer. You’ll go case by case through important scenarios and challenges for object design and then walk through a simple web application that demonstrates how different types of objects can work together effectively.
What You Will Learn
Universal design rules for a wide range of objects
Best practices for testing objects
A catalog of common object types
Changing and exposing state
Test your object design skills with exercises
This Book Is Written For
For readers familiar with an object-oriented language and basic application architecture.
About the Author
Matthias Noback is a professional web developer with nearly two decades of experience. He runs his own web development, training, and consultancy company called “Noback’s Office.”
Table of Contents:
1 ¦ Programming with objects: A primer
2 ¦ Creating services
3 ¦ Creating other objects
4 ¦ Manipulating objects
5 ¦ Using objects
6 ¦ Retrieving information
7 ¦ Performing tasks
8 ¦ Dividing responsibilities
9 ¦ Changing the behavior of services
10 ¦ A field guide to objects
11 ¦ Epilogue
1137832072
Key Features
Dozens of techniques for writing object-oriented code that’s easy to read, reuse, and maintain
Write code that other programmers will instantly understand
Design rules for constructing objects, changing and exposing state, and more
Examples written in an instantly familiar pseudocode that’s easy to apply to Java, Python, C#, and any object-oriented language
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About The Book
Well-written object-oriented code is easy to read, modify, and debug. Elevate your coding style by mastering the universal best practices for object design presented in this book. These clearly presented rules, which apply to any OO language, maximize the clarity and durability of your codebase and increase productivity for you and your team.
In Object Design Style Guide, veteran developer Matthias Noback lays out design rules for constructing objects, defining methods, and much more. All examples use instantly familiar pseudocode, so you can follow along in the language you prefer. You’ll go case by case through important scenarios and challenges for object design and then walk through a simple web application that demonstrates how different types of objects can work together effectively.
What You Will Learn
Universal design rules for a wide range of objects
Best practices for testing objects
A catalog of common object types
Changing and exposing state
Test your object design skills with exercises
This Book Is Written For
For readers familiar with an object-oriented language and basic application architecture.
About the Author
Matthias Noback is a professional web developer with nearly two decades of experience. He runs his own web development, training, and consultancy company called “Noback’s Office.”
Table of Contents:
1 ¦ Programming with objects: A primer
2 ¦ Creating services
3 ¦ Creating other objects
4 ¦ Manipulating objects
5 ¦ Using objects
6 ¦ Retrieving information
7 ¦ Performing tasks
8 ¦ Dividing responsibilities
9 ¦ Changing the behavior of services
10 ¦ A field guide to objects
11 ¦ Epilogue
Object Design Style Guide
”Demystifies object-oriented programming, and lays out how to use it to design truly secure and performant applications.” —Charles Soetan, Plum.io
Key Features
Dozens of techniques for writing object-oriented code that’s easy to read, reuse, and maintain
Write code that other programmers will instantly understand
Design rules for constructing objects, changing and exposing state, and more
Examples written in an instantly familiar pseudocode that’s easy to apply to Java, Python, C#, and any object-oriented language
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About The Book
Well-written object-oriented code is easy to read, modify, and debug. Elevate your coding style by mastering the universal best practices for object design presented in this book. These clearly presented rules, which apply to any OO language, maximize the clarity and durability of your codebase and increase productivity for you and your team.
In Object Design Style Guide, veteran developer Matthias Noback lays out design rules for constructing objects, defining methods, and much more. All examples use instantly familiar pseudocode, so you can follow along in the language you prefer. You’ll go case by case through important scenarios and challenges for object design and then walk through a simple web application that demonstrates how different types of objects can work together effectively.
What You Will Learn
Universal design rules for a wide range of objects
Best practices for testing objects
A catalog of common object types
Changing and exposing state
Test your object design skills with exercises
This Book Is Written For
For readers familiar with an object-oriented language and basic application architecture.
About the Author
Matthias Noback is a professional web developer with nearly two decades of experience. He runs his own web development, training, and consultancy company called “Noback’s Office.”
Table of Contents:
1 ¦ Programming with objects: A primer
2 ¦ Creating services
3 ¦ Creating other objects
4 ¦ Manipulating objects
5 ¦ Using objects
6 ¦ Retrieving information
7 ¦ Performing tasks
8 ¦ Dividing responsibilities
9 ¦ Changing the behavior of services
10 ¦ A field guide to objects
11 ¦ Epilogue
Key Features
Dozens of techniques for writing object-oriented code that’s easy to read, reuse, and maintain
Write code that other programmers will instantly understand
Design rules for constructing objects, changing and exposing state, and more
Examples written in an instantly familiar pseudocode that’s easy to apply to Java, Python, C#, and any object-oriented language
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About The Book
Well-written object-oriented code is easy to read, modify, and debug. Elevate your coding style by mastering the universal best practices for object design presented in this book. These clearly presented rules, which apply to any OO language, maximize the clarity and durability of your codebase and increase productivity for you and your team.
In Object Design Style Guide, veteran developer Matthias Noback lays out design rules for constructing objects, defining methods, and much more. All examples use instantly familiar pseudocode, so you can follow along in the language you prefer. You’ll go case by case through important scenarios and challenges for object design and then walk through a simple web application that demonstrates how different types of objects can work together effectively.
What You Will Learn
Universal design rules for a wide range of objects
Best practices for testing objects
A catalog of common object types
Changing and exposing state
Test your object design skills with exercises
This Book Is Written For
For readers familiar with an object-oriented language and basic application architecture.
About the Author
Matthias Noback is a professional web developer with nearly two decades of experience. He runs his own web development, training, and consultancy company called “Noback’s Office.”
Table of Contents:
1 ¦ Programming with objects: A primer
2 ¦ Creating services
3 ¦ Creating other objects
4 ¦ Manipulating objects
5 ¦ Using objects
6 ¦ Retrieving information
7 ¦ Performing tasks
8 ¦ Dividing responsibilities
9 ¦ Changing the behavior of services
10 ¦ A field guide to objects
11 ¦ Epilogue
28.99
In Stock
5
1
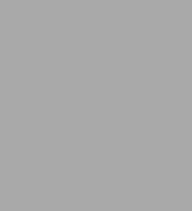
Object Design Style Guide
288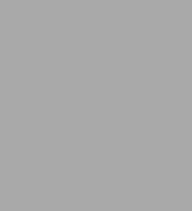
Object Design Style Guide
288Related collections and offers
28.99
In Stock
From the B&N Reads Blog