Winner of the 2014 Jolt Award for "Best Book"
“Whether you are an experienced programmer or are starting your career, Python in Practice is full of valuable advice and example to help you improve your craft by thinking about problems from different perspectives, introducing tools, and detailing techniques to create more effective solutions.”
—Doug Hellmann, Senior Developer, DreamHost
If you’re an experienced Python programmer, Python in Practice will help you improve the quality, reliability, speed, maintainability, and usability of all your Python programs.
Mark Summerfield focuses on four key themes: design patterns for coding elegance, faster processing through concurrency and compiled Python (Cython), high-level networking, and graphics. He identifies well-proven design patterns that are useful in Python, illuminates them with expert-quality code, and explains why some object-oriented design patterns are irrelevant to Python. He also explodes several counterproductive myths about Python programming—showing, for example, how Python can take full advantage of multicore hardware.
All examples, including three complete case studies, have been tested with Python 3.3 (and, where possible, Python 3.2 and 3.1) and crafted to maintain compatibility with future Python 3.x versions. All code has been tested on Linux, and most code has also been tested on OS X and Windows. All code may be downloaded at www.qtrac.eu/pipbook.html.
Coverage includes
- Leveraging Python’s most effective creational, structural, and behavioral design patterns
- Supporting concurrency with Python’s multiprocessing, threading, and concurrent.futures modules
- Avoiding concurrency problems using thread-safe queues and futures rather than fragile locks
- Simplifying networking with high-level modules, including xmlrpclib and RPyC
- Accelerating Python code with Cython, C-based Python modules, profiling, and other techniques
- Creating modern-looking GUI applications with Tkinter
- Leveraging today’s powerful graphics hardware via the OpenGL API using pyglet and PyOpenGL
Winner of the 2014 Jolt Award for "Best Book"
“Whether you are an experienced programmer or are starting your career, Python in Practice is full of valuable advice and example to help you improve your craft by thinking about problems from different perspectives, introducing tools, and detailing techniques to create more effective solutions.”
—Doug Hellmann, Senior Developer, DreamHost
If you’re an experienced Python programmer, Python in Practice will help you improve the quality, reliability, speed, maintainability, and usability of all your Python programs.
Mark Summerfield focuses on four key themes: design patterns for coding elegance, faster processing through concurrency and compiled Python (Cython), high-level networking, and graphics. He identifies well-proven design patterns that are useful in Python, illuminates them with expert-quality code, and explains why some object-oriented design patterns are irrelevant to Python. He also explodes several counterproductive myths about Python programming—showing, for example, how Python can take full advantage of multicore hardware.
All examples, including three complete case studies, have been tested with Python 3.3 (and, where possible, Python 3.2 and 3.1) and crafted to maintain compatibility with future Python 3.x versions. All code has been tested on Linux, and most code has also been tested on OS X and Windows. All code may be downloaded at www.qtrac.eu/pipbook.html.
Coverage includes
- Leveraging Python’s most effective creational, structural, and behavioral design patterns
- Supporting concurrency with Python’s multiprocessing, threading, and concurrent.futures modules
- Avoiding concurrency problems using thread-safe queues and futures rather than fragile locks
- Simplifying networking with high-level modules, including xmlrpclib and RPyC
- Accelerating Python code with Cython, C-based Python modules, profiling, and other techniques
- Creating modern-looking GUI applications with Tkinter
- Leveraging today’s powerful graphics hardware via the OpenGL API using pyglet and PyOpenGL
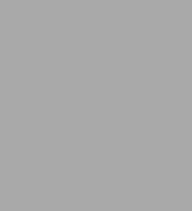
Python in Practice: Create Better Programs Using Concurrency, Libraries, and Patterns
336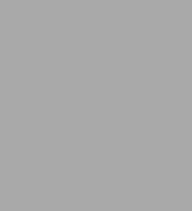
Python in Practice: Create Better Programs Using Concurrency, Libraries, and Patterns
336Product Details
ISBN-13: | 9780133373233 |
---|---|
Publisher: | Pearson Education |
Publication date: | 08/20/2013 |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 336 |
File size: | 31 MB |
Note: | This product may take a few minutes to download. |
Age Range: | 18 Years |