The Recursive Book of Recursion: Ace the Coding Interview with Python and JavaScript
An accessible yet rigorous crash course on recursive programming using Python and JavaScript examples.
Recursion has an intimidating reputation: it’s considered to be an advanced computer science topic frequently brought up in coding interviews. But there’s nothing magical about recursion.
The Recursive Book of Recursion uses Python and JavaScript examples to teach the basics of recursion, exposing the ways that it’s often poorly taught and clarifying the fundamental principles of all recursive algorithms. You’ll learn when to use recursive functions (and, most importantly, when not to use them), how to implement the classic recursive algorithms often brought up in job interviews, and how recursive techniques can help solve countless problems involving tree traversal, combinatorics, and other tricky topics.
This project-based guide contains complete, runnable programs to help you learn:
How recursive functions make use of the call stack, a critical data structure almost never discussed in lessons on recursion How the head-tail and “leap of faith” techniques can simplify writing recursive functions How to use recursion to write custom search scripts for your filesystem, draw fractal art, create mazes, and more How optimization and memoization make recursive algorithms more efficient
Al Sweigart has built a career explaining programming concepts in a fun, approachable manner. If you’ve shied away from learning recursion but want to add this technique to your programming toolkit, or if you’re racing to prepare for your next job interview, this book is for you.
1140041607
Recursion has an intimidating reputation: it’s considered to be an advanced computer science topic frequently brought up in coding interviews. But there’s nothing magical about recursion.
The Recursive Book of Recursion uses Python and JavaScript examples to teach the basics of recursion, exposing the ways that it’s often poorly taught and clarifying the fundamental principles of all recursive algorithms. You’ll learn when to use recursive functions (and, most importantly, when not to use them), how to implement the classic recursive algorithms often brought up in job interviews, and how recursive techniques can help solve countless problems involving tree traversal, combinatorics, and other tricky topics.
This project-based guide contains complete, runnable programs to help you learn:
Al Sweigart has built a career explaining programming concepts in a fun, approachable manner. If you’ve shied away from learning recursion but want to add this technique to your programming toolkit, or if you’re racing to prepare for your next job interview, this book is for you.
The Recursive Book of Recursion: Ace the Coding Interview with Python and JavaScript
An accessible yet rigorous crash course on recursive programming using Python and JavaScript examples.
Recursion has an intimidating reputation: it’s considered to be an advanced computer science topic frequently brought up in coding interviews. But there’s nothing magical about recursion.
The Recursive Book of Recursion uses Python and JavaScript examples to teach the basics of recursion, exposing the ways that it’s often poorly taught and clarifying the fundamental principles of all recursive algorithms. You’ll learn when to use recursive functions (and, most importantly, when not to use them), how to implement the classic recursive algorithms often brought up in job interviews, and how recursive techniques can help solve countless problems involving tree traversal, combinatorics, and other tricky topics.
This project-based guide contains complete, runnable programs to help you learn:
How recursive functions make use of the call stack, a critical data structure almost never discussed in lessons on recursion How the head-tail and “leap of faith” techniques can simplify writing recursive functions How to use recursion to write custom search scripts for your filesystem, draw fractal art, create mazes, and more How optimization and memoization make recursive algorithms more efficient
Al Sweigart has built a career explaining programming concepts in a fun, approachable manner. If you’ve shied away from learning recursion but want to add this technique to your programming toolkit, or if you’re racing to prepare for your next job interview, this book is for you.
Recursion has an intimidating reputation: it’s considered to be an advanced computer science topic frequently brought up in coding interviews. But there’s nothing magical about recursion.
The Recursive Book of Recursion uses Python and JavaScript examples to teach the basics of recursion, exposing the ways that it’s often poorly taught and clarifying the fundamental principles of all recursive algorithms. You’ll learn when to use recursive functions (and, most importantly, when not to use them), how to implement the classic recursive algorithms often brought up in job interviews, and how recursive techniques can help solve countless problems involving tree traversal, combinatorics, and other tricky topics.
This project-based guide contains complete, runnable programs to help you learn:
Al Sweigart has built a career explaining programming concepts in a fun, approachable manner. If you’ve shied away from learning recursion but want to add this technique to your programming toolkit, or if you’re racing to prepare for your next job interview, this book is for you.
39.99
In Stock
5
1
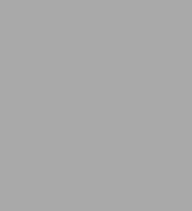
The Recursive Book of Recursion: Ace the Coding Interview with Python and JavaScript
328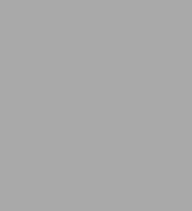
The Recursive Book of Recursion: Ace the Coding Interview with Python and JavaScript
328
39.99
In Stock
Product Details
ISBN-13: | 9781718502024 |
---|---|
Publisher: | No Starch Press |
Publication date: | 08/16/2022 |
Pages: | 328 |
Product dimensions: | 6.90(w) x 9.10(h) x 0.90(d) |
From the B&N Reads Blog